Solidity-Solidity coding solutions
AI-powered Solidity Developer

✏️ Write a smart contract for decentralized voting
💰 Create a token swap feature with liquidity pools
🪲 Find any bug or improvement in my smart contract
💡 Teach me a useful skill or trick in Solidity
Related Tools
Load More
Solidity Developer
An expert Solidity developer aiding in smart contract creation and optimization.

CryptoDo - smart contract builder
Turn your idea into high-quality Solidity smart contracts

Solidity AI
I'm an expert in Solidity and EVM, here to write code, audit, and advise on web3 architecture.
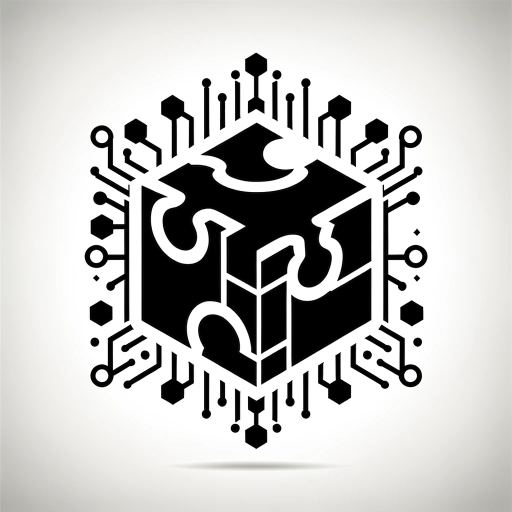
Smart Contract Analyzer
Analyzes the source code & ABI of smart contracts compatible with EVM. Supports networks: Ethereum, BSC, Polygon, Arbitrum, Optimism, ZkSync.

Ethereum GPT
Answers any Ethereum spec related question

Solidity Master
I'll help you master Solidity to become a better smart contract developer.
20.0 / 5 (200 votes)
Introduction to Solidity
Solidity is a statically-typed programming language designed for developing smart contracts on the Ethereum blockchain. It was influenced by C++, Python, and JavaScript and is compiled to bytecode for the Ethereum Virtual Machine (EVM). The primary purpose of Solidity is to enable developers to create contracts for applications that can execute complex transactions, enforce agreements, and automate workflows securely and transparently. These contracts are self-executing with the terms of the agreement directly written into code. Solidity supports inheritance, libraries, and complex user-defined types, providing developers with extensive capabilities to build sophisticated decentralized applications (DApps).
Main Functions of Solidity
Contract Creation
Example
pragma solidity ^0.8.0; contract SimpleStorage { uint public data; function set(uint x) public { data = x; } function get() public view returns (uint) { return data; }}
Scenario
This basic example illustrates a simple storage contract where a user can store and retrieve an integer value. Such a contract could be a foundational element for more complex applications requiring data persistence, such as a decentralized database.
Token Implementation
Example
pragma solidity ^0.8.0; import '@openzeppelin/contracts/token/ERC20/ERC20.sol'; contract MyToken is ERC20 { constructor() ERC20('MyToken', 'MTK') { _mint(msg.sender, 1000 * 10 ** decimals()); }}
Scenario
This example demonstrates the creation of an ERC20 token using the OpenZeppelin library. Tokens are the backbone of many blockchain applications, including ICOs, DeFi platforms, and blockchain-based games. Implementing a token contract can facilitate the creation of a new cryptocurrency, support governance models, or enable asset transfer within a DApp.
Crowdfunding
Example
pragma solidity ^0.8.0; contract Crowdfunding { address public owner; uint public goal; uint public endTime; mapping(address => uint) public contributions; constructor(uint _goal, uint _duration) { owner = msg.sender; goal = _goal; endTime = block.timestamp + _duration; } function contribute() public payable { require(block.timestamp < endTime, 'Crowdfunding ended'); contributions[msg.sender] += msg.value; } function withdraw() public { require(msg.sender == owner, 'Only owner'); require(block.timestamp > endTime, 'Crowdfunding ongoing'); require(address(this).balance >= goal, 'Goal not met'); payable(owner).transfer(address(this).balance); }}
Scenario
This contract represents a basic crowdfunding campaign where users can contribute funds towards a project goal within a specific timeframe. If the goal is met, the project owner can withdraw the funds; otherwise, contributors can reclaim their contributions. This is useful for fundraising projects, enabling transparency and trust in the collection and allocation of funds.
Ideal Users of Solidity
Blockchain Developers
These are programmers and software engineers who specialize in creating decentralized applications. Solidity provides them with the tools to write smart contracts that run on the Ethereum blockchain. They benefit from Solidity's strong typing, inheritance, and user-defined types, which enable the creation of secure, scalable, and efficient contracts.
Entrepreneurs and Startups
Individuals or small companies looking to leverage blockchain technology for innovative solutions can benefit from Solidity. Whether it's launching a new cryptocurrency, developing a decentralized finance (DeFi) platform, or creating a decentralized marketplace, Solidity allows entrepreneurs to implement their ideas securely and transparently on the Ethereum network.
Guidelines for Using Solidity
Visit aichatonline.org
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Install Required Tools
Ensure you have Node.js, npm, and Truffle installed. These tools are essential for Solidity development.
Set Up Development Environment
Create a new project directory and initialize it with Truffle. Configure your Truffle project with necessary settings.
Write Smart Contracts
Create Solidity files (.sol) and start writing your smart contracts. Use best practices for security and efficiency.
Test and Deploy
Write test scripts in JavaScript and use Truffle to test and deploy your contracts to a blockchain network.
Try other advanced and practical GPTs
GlitchB!tch2
Unleash Digital Chaos with AI Glitch

Orçamentos Rápidos da R3 Rental Car
AI-powered car rental quotes

The CryptoSage
AI-powered cryptocurrency insights and analysis.
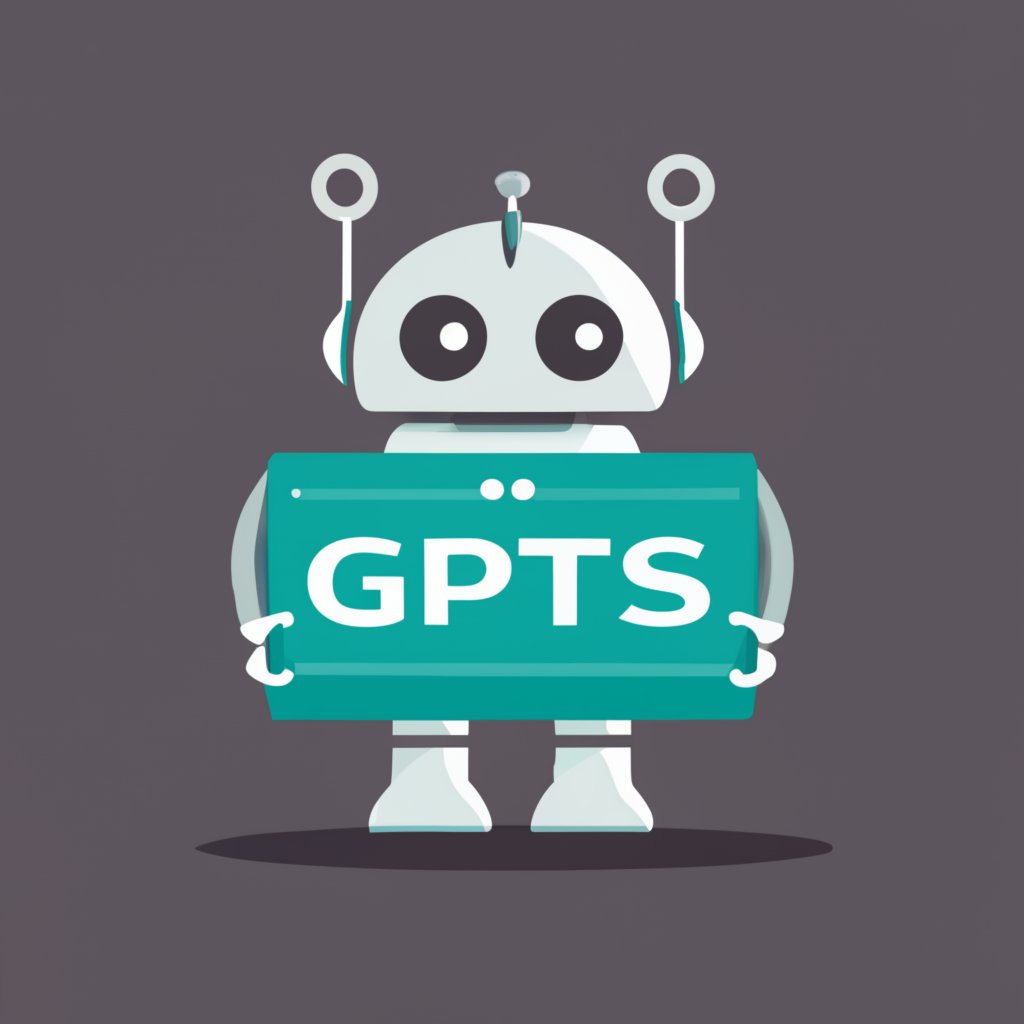
Home Assistant
AI-powered smart home automation

Coupert
AI-Powered Savings and Cashback Tool

Mamacita GPT
Your AI-powered Latina amiga

Marketing RRSS - Magenta Consultores
Empower Your Marketing with AI

Light Yagami
AI-Powered Intelligence for Your Needs

LetzAI
AI-powered Image Creation Tool

Nuxt
AI-powered framework for web development

漫画読んで評価する姐さん
AI-powered manga evaluation tool

のInstagram Big Writer
AI-powered Instagram content creation

- Smart Contracts
- Blockchain Development
- Token Creation
- DApp Development
- DeFi Applications
Common Solidity Q&A
What is Solidity?
Solidity is a high-level programming language used for writing smart contracts on the Ethereum blockchain.
How do you compile Solidity code?
You can compile Solidity code using the Solidity compiler (solc) or tools like Truffle that integrate with solc.
What are smart contracts?
Smart contracts are self-executing contracts with the terms directly written into code. They run on blockchain networks like Ethereum.
How do you handle errors in Solidity?
Error handling in Solidity is done using revert(), require(), and assert() statements to enforce conditions and handle exceptions.
Can Solidity interact with other contracts?
Yes, Solidity can interact with other contracts through external calls using interfaces or direct contract addresses.