Advanced Object Oriented Programming-advanced OOP guidance and tutorials
AI-Powered Advanced OOP Insights

Explain the first concept from the slides.
Detail the second concept with an example.
How does the third concept apply in practice?
Illustrate the fourth concept with Java code.
Related Tools
Load More
Java
Your personal Java / Spring assistant and project generator with a focus on responsive and scalable code. Write clean code and become a much faster developer.

Typescript
Advanced Typescript assistant and code generator with a focus on responsive, efficient, and scalable code. Write clean code and become a much faster developer.

Advanced PHP Assistant
A friendly PHP programming assistant, ready to assist you.
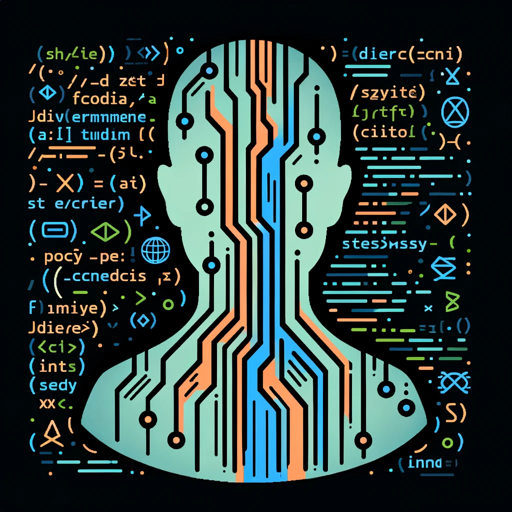
Expert Programmer
Expert in programming with a focus on simplifying code

Advanced Java Assistant
A friendly Java programming assistant, ready to assist you.

My OpenGL C++ Buddy
A C++ programming assistant offering code help and explanations.
20.0 / 5 (200 votes)
Introduction to Advanced Object Oriented Programming
Advanced Object Oriented Programming (A-OOP) builds upon the fundamental principles of Object-Oriented Programming (OOP), such as encapsulation, inheritance, and polymorphism, by introducing more complex concepts and techniques that allow developers to design more modular, maintainable, and scalable software systems. A-OOP is crucial for tackling large-scale software projects, where the architecture must be flexible enough to accommodate future changes and extensions. Key components of A-OOP include design patterns, SOLID principles, metaprogramming, and advanced use of inheritance and composition. For instance, consider a scenario in a financial trading application where you need to handle various types of financial instruments (e.g., stocks, bonds, options). Basic OOP would allow you to define classes for each instrument, but A-OOP principles would enable you to implement patterns like Strategy or Decorator to handle different pricing algorithms or additional features dynamically, making your system more adaptable and easier to maintain.
Main Functions of Advanced Object Oriented Programming
Design Patterns
Example
Using the Observer pattern to implement an event notification system.
Scenario
In a stock trading application, different parts of the system (e.g., user interface, data logger, analytics engine) need to be updated whenever there is a change in stock prices. By implementing the Observer pattern, you can ensure that all these components are notified and updated automatically whenever a stock price changes, without them needing to be tightly coupled.
SOLID Principles
Example
Applying the Single Responsibility Principle (SRP) to a class that handles both user authentication and data validation.
Scenario
In an e-commerce application, a single class might initially be responsible for authenticating users and validating their input data. By applying SRP, you can refactor this class into two distinct classes—one for authentication and another for data validation—making each class easier to maintain, test, and extend independently.
Metaprogramming
Example
Using reflection to dynamically create instances of classes based on configuration files.
Scenario
In a plugin-based architecture, where new modules can be added without recompiling the entire application, reflection allows you to load classes and create objects at runtime based on external configuration, thus supporting a highly flexible and extensible system.
Ideal Users of Advanced Object Oriented Programming
Software Architects and Senior Developers
These users are responsible for designing the overall architecture of large-scale software systems. They benefit from A-OOP because it provides them with the tools and methodologies to create flexible, scalable, and maintainable software architectures. By utilizing advanced design patterns, SOLID principles, and metaprogramming techniques, they can ensure that the system is robust and can evolve over time without requiring a complete overhaul.
Developers Working on Complex, Modular Applications
Developers who are tasked with building or maintaining complex, modular applications—such as enterprise software, large web applications, or systems that require high levels of customization—will find A-OOP essential. It helps them manage the complexity of their codebase, reduce technical debt, and improve code reusability, leading to more efficient development processes and easier long-term maintenance.
Guidelines for Using Advanced Object Oriented Programming
Step 1
Visit aichatonline.org for a free trial without login, no need for ChatGPT Plus.
Step 2
Ensure you have a foundational understanding of basic object-oriented programming principles, such as classes, objects, inheritance, and polymorphism, as these are prerequisites for advanced concepts.
Step 3
Explore advanced concepts like design patterns (Singleton, Factory, Observer), SOLID principles, and dependency injection to understand their applications in large-scale software development.
Step 4
Apply these advanced techniques in real-world projects or coding challenges, focusing on modularity, reusability, and maintainability of code. Use IDEs like IntelliJ IDEA or Eclipse for efficient coding and debugging.
Step 5
Continuously refine your skills by reviewing open-source projects, participating in coding forums, and practicing with advanced exercises that push the boundaries of your object-oriented design abilities.
Try other advanced and practical GPTs
GrammarGPT
AI-powered grammar and translation assistant

Rebel Replies
AI-powered claims negotiation for contractors.

Český Právník
AI-powered tool for understanding Czech law.

Daily Biblical Decrees and Declarations!
AI-powered biblical prayer declarations

Red Pill Alpha Coach
AI-Powered Guidance for Modern Men

Optics Expert
AI-powered Optical Engineering Assistant

Python hacker
AI-Powered Python Mastery

Video Summary Pro
AI-powered video summarization tool.

Legal Memo Writer
AI-powered objective legal memo writer

SunoBeats
AI-powered music creation at your fingertips

Calculation Expert
AI-driven solutions for everyday tasks

Professional Writer, Analysis and Calculation Pro
AI-powered writing and analysis made simple.

- Design Patterns
- Software Architecture
- Coding Practices
- Code Design
- OOP Principles
Q&A on Advanced Object Oriented Programming
What is Advanced Object Oriented Programming?
Advanced Object Oriented Programming builds on the basics of OOP by introducing complex concepts such as design patterns, principles like SOLID, and advanced techniques for improving software architecture and code maintainability.
How can Advanced Object Oriented Programming improve software development?
By using advanced OOP techniques, developers can create more modular, reusable, and maintainable code, which is critical for large-scale projects. It also allows for better scalability and easier debugging.
What are some common design patterns in Advanced Object Oriented Programming?
Common design patterns include Singleton, Factory, Observer, and Strategy. These patterns provide proven solutions to common design problems, making your code more efficient and easier to understand.
Why is SOLID important in Advanced Object Oriented Programming?
SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) guide the creation of robust, flexible, and maintainable object-oriented systems by promoting clear and effective design practices.
How can I practice Advanced Object Oriented Programming?
You can practice by working on complex projects, contributing to open-source, solving advanced coding problems, and experimenting with design patterns and principles in different coding environments.