Typescript-advanced JavaScript superset
AI-powered TypeScript Development Tool

⚙️ Transform javascript code into typescript
✨ Create types and interfaces for this code
🪲 Find any bug or improvement in my code
💡 Teach me a useful skill or trick in Typescript
Related Tools
Load More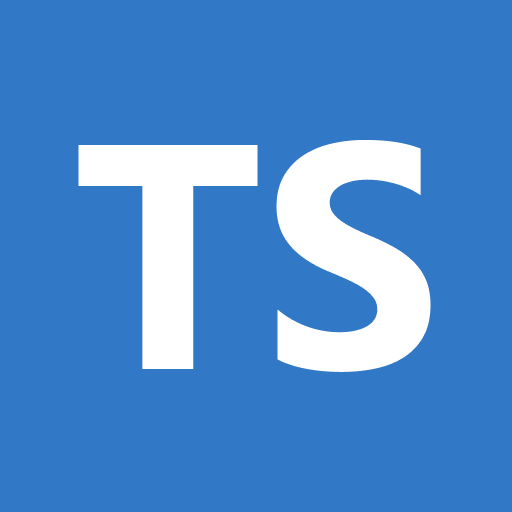
TypeScript Engineer
An expert TypeScript engineer to help you solve and debug problems together.

Typescript/React/Tailwind
Frontend dev assistant for TypeScript, Tailwind, React, with minimal code comments.

Typescript Nodejs Developer
Node.js expert with step-by-step problem solving focus

TypeScript/NextJS 14/Supabase Expert
Technical assistant for NextJS 14, Supabase, TypeScript development

React TypeScript Pro
Friendly React & TypeScript expert with contextual memory.

TypeScript Expert
Advanced TypeScript coding assistant informed by official docs
20.0 / 5 (200 votes)
Introduction to TypeScript
TypeScript is a strongly typed, compiled superset of JavaScript that aims to enhance JavaScript's capabilities by adding static typing and other features. Developed by Microsoft and first released in 2012, TypeScript enables developers to write clearer, more maintainable code. By providing static types, TypeScript helps catch errors at compile time, rather than runtime, which leads to more reliable and predictable code. It is particularly beneficial for large-scale projects and applications where maintainability and code quality are critical. For instance, when building a complex web application, TypeScript helps enforce type constraints, making the codebase easier to understand and less prone to bugs. It also integrates seamlessly with popular JavaScript frameworks like React, Angular, and Vue.js, enhancing their development experience.
Main Functions of TypeScript
Static Typing
Example
In TypeScript, you can define the type of a variable, function parameter, or return type. For example, `let age: number = 30;` ensures that `age` can only be a number.
Scenario
Static typing is crucial in scenarios where you need to manage a large codebase, ensuring that functions receive and return the expected data types. This reduces bugs and makes the code more understandable, as the types act as a form of documentation.
Interface and Type Aliases
Example
Interfaces in TypeScript allow you to define the structure of an object. For example, `interface Person { name: string; age: number; }`.
Scenario
Using interfaces is beneficial in situations where multiple functions or classes share common structures. For instance, in a web application handling user data, interfaces ensure that all user-related functions and objects adhere to the same structure.
Advanced Type Features
Example
TypeScript supports advanced features like Union types (`let value: string | number;`), Intersection types, and Generics (`function identity<T>(arg: T): T { return arg; }`).
Scenario
These advanced types are useful in complex scenarios, such as when creating reusable components or functions that can handle different data types. For example, generics are heavily used in libraries and frameworks to provide type-safe operations.
Ideal Users of TypeScript
Enterprise Developers
Enterprise developers working on large-scale applications benefit significantly from TypeScript. It helps manage complex codebases with ease, provides better tooling and error checking, and ensures a more robust, maintainable codebase. TypeScript's type safety and tooling integration reduce bugs and improve the overall quality of the software.
JavaScript Developers
JavaScript developers looking to enhance their coding experience and improve the reliability of their code are also ideal users of TypeScript. By adding static typing and modern JavaScript features, TypeScript helps developers catch errors early and write cleaner, more predictable code. It's especially beneficial for those working with frameworks like React or Angular, where TypeScript's features can be fully leveraged to improve development efficiency.
Steps to Use TypeScript
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Install Node.js and npm, which are prerequisites for installing TypeScript.
Step 3
Install TypeScript globally using the npm command `npm install -g typescript`.
Step 4
Create a TypeScript configuration file `tsconfig.json` to specify the compiler options.
Step 5
Write TypeScript code in `.ts` files and compile them to JavaScript using the `tsc` command.
Try other advanced and practical GPTs
Django Copilot
AI-powered Django Development Assistant

JurisHand AI: Vade Mecum de Direito
AI-Powered Legal Insight at Your Fingertips

Tabletop Map Architect
Create detailed maps for tabletop games with AI.

DataAnalyst GPT | Intelligent Analysis 📊🤖
AI-driven insights for smarter decisions

The Polymath
AI-Powered Insight for Every Query.

UX Design and Research. ai
AI-powered UX design and research.
Web Search Copilot
AI-Powered Searches, Insights Delivered.
Medical Diagnosis Analysis
AI-Powered Medical Insights

PPT and PDF Analyst with Image Analysis
AI-powered insights for your documents.

Stata Analyst
AI-powered Stata code generator.

Flutter Expert
AI-powered Flutter development guidance.

Correcteur linguistique français
AI-powered French grammar and spelling correction
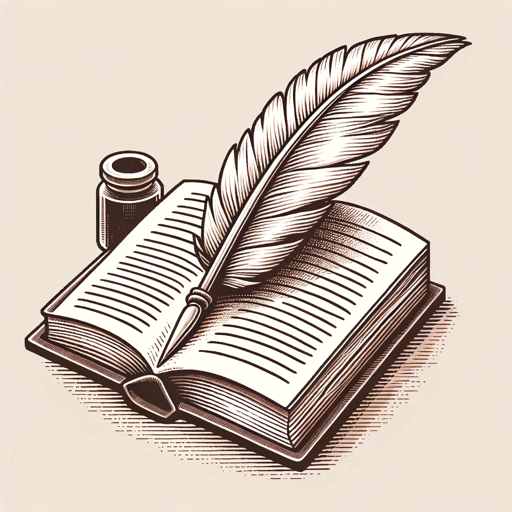
- Web Development
- Code Refactoring
- Type Safety
- Backend Programming
- Static Typing
TypeScript Q&A
What is TypeScript?
TypeScript is a strongly typed, object-oriented, compiled language. It is a superset of JavaScript, providing optional static typing and class-based object-oriented programming.
How do you compile TypeScript code?
TypeScript code is compiled using the TypeScript compiler (tsc). You can compile a TypeScript file by running `tsc filename.ts`, which generates a corresponding JavaScript file.
What are the benefits of using TypeScript?
TypeScript offers several benefits, including early detection of errors through static type checking, enhanced IDE support, and features like classes, interfaces, and modules that facilitate code organization and reuse.
Can I use existing JavaScript libraries in TypeScript?
Yes, TypeScript is compatible with existing JavaScript code and libraries. You can use declaration files to describe the shape of these libraries for better integration and type checking.
What is the difference between TypeScript and JavaScript?
The main difference is that TypeScript is a superset of JavaScript that adds optional static types. This helps catch errors at compile time, provides better tooling and refactoring capabilities, and supports modern JavaScript features.