Backend API Python/ Django/ Django REST Framework-trial backend API toolkit
AI-powered backend API development tool
How do I optimize a Django query?
Explain Django's MVT architecture.
Best practices for REST API in Django?
How to handle authentication in Django REST?
Related Tools
Load More
Django Copilot
Your personal Django assistant and code generator with a focus on responsive, efficient, and scalable projects. Write clean code and become a much faster developer.

Django Pro
Your Dedicated Assistant for Streamlined Python and Django App Development

FastAPI
A helpful guide for learning and using FastAPI in development.
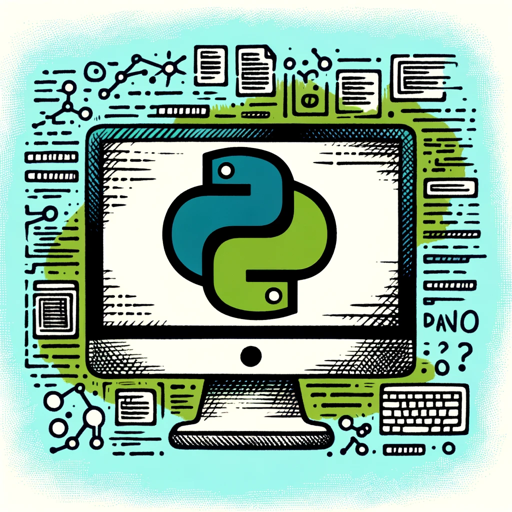
Django Dev Helper
Your go-to Django development assistant.

FastAPI
Your personal Fast API assistant and code generator with a focus on responsive, efficient, and scalable projects. Write clean code and become a much faster developer.

BACK END FAST API
API Gateway & Security Expert
20.0 / 5 (200 votes)
Introduction to Backend API Development with Python, Django, and Django REST Framework
Backend API development involves creating the server-side logic that powers applications, manages databases, and serves data to client-side applications (such as web or mobile apps). Python is a popular language for backend development due to its readability, simplicity, and extensive libraries. Django is a high-level Python web framework that promotes rapid development, clean design, and scalability. Django REST Framework (DRF) is an extension of Django, specifically designed to build robust and flexible APIs. DRF simplifies the process of creating RESTful APIs by providing powerful tools for serialization, authentication, viewsets, and routing, all while adhering to the principles of Django’s ORM and request/response handling mechanisms. For example, a typical scenario might involve building a social media platform where you need to expose various APIs to manage users, posts, and comments. Django handles the database and model logic, while DRF allows you to create APIs that return JSON data to the frontend. This way, your frontend apps can interact with the backend seamlessly, whether it's through fetching posts, creating new comments, or managing user profiles.
Main Functions of Backend API Development Using Django and Django REST Framework
Database Management and ORM
Example
Django provides a powerful Object-Relational Mapping (ORM) system that simplifies interactions with the database by mapping Python objects to database tables.
Scenario
Consider an e-commerce application where you need to manage products, customers, and orders. Instead of writing raw SQL, you define models in Python that correspond to these entities. Django automatically handles the creation and manipulation of database tables based on these models, allowing you to focus on business logic rather than SQL queries.
Serialization and Deserialization
Example
DRF provides serializers to convert complex data types, such as querysets and model instances, into Python-native datatypes (e.g., JSON). This is crucial for API responses.
Scenario
In a blogging platform, you might need to expose a list of blog posts to be displayed on a mobile app. Using serializers, you can convert a queryset of blog post objects into JSON format, which can be consumed by the frontend. Conversely, when creating a new post via API, DRF can deserialize the incoming JSON data back into a Python object and save it to the database.
Authentication and Permissions
Example
DRF offers built-in authentication schemes such as Token Authentication, Session Authentication, and support for third-party OAuth providers.
Scenario
Imagine you're developing a task management tool with different user roles (e.g., admin, user). Using DRF's authentication and permission classes, you can enforce that only authenticated users can access certain endpoints, while admins have full access to all resources. For instance, regular users may be allowed to create and update their tasks, but only admins can delete tasks.
Ideal Users of Backend API Development with Django and Django REST Framework
Web and Mobile Application Developers
Developers building dynamic applications with a need for a reliable, scalable backend. These users benefit from Django's ORM, built-in admin interface, and DRF’s powerful tools to create APIs. For example, a team building a social networking site or a project management tool can use Django and DRF to easily manage complex data relationships, user roles, and secure API endpoints.
Startups and Rapid Prototyping Teams
Startups or teams working on MVPs (Minimum Viable Products) benefit from Django’s rapid development features, including its extensive out-of-the-box functionality. For instance, a startup developing an online marketplace can quickly define models for products, categories, and transactions, and use DRF to expose APIs to mobile apps or frontend frameworks like React or Vue.js.
Guidelines for Using Backend API with Python, Django, and Django REST Framework
Visit aichatonline.org for a free trial without login
Explore the features and capabilities of the Backend API Python/Django/Django REST Framework without needing to sign up or purchase ChatGPT Plus. Get started immediately with a free trial.
Set Up Your Development Environment
Ensure you have Python installed (version 3.8 or higher is recommended). Install Django using pip (`pip install django`) and add Django REST Framework (`pip install djangorestframework`).
Create a Django Project and App
Start a new Django project using `django-admin startproject projectname`. Then, create an app within the project using `python manage.py startapp appname`. This app will contain your API logic.
Define Models and Serializers
Design your database schema using Django models. Create serializers to convert complex data types (like querysets) into JSON. Serializers are crucial for data validation and manipulation in APIs.
Implement API Views and Routes
Use Django REST Framework views (Function-Based Views or Class-Based Views) to handle API requests. Set up URL routing to connect these views to specific endpoints. Test your API with tools like Postman.
Try other advanced and practical GPTs
OHIF-Bot
AI-Powered Support for Medical Imaging Tools.

+COPYWRITER+
AI-Powered Copywriting, Simplified

不思議体験談
AI-Powered Creative and Informative Tool

PPT演讲稿生成器
AI-powered speech scripts for presentations

核心拟题
AI-driven tool for precise research titles

网页信息读取器
AI-powered web content extractor

YoeuTube 阿美英文
Learn English naturally with AI.

Plant Simulation Help Navigator
AI-powered guidance for Plant Simulation.

PolisEthic
AI-driven solutions for a better society.

StoryCraft Studio
Unleash Your Creativity with AI-Powered Storytelling

Academic literature search and review GPT
AI-Powered Academic Literature Review.
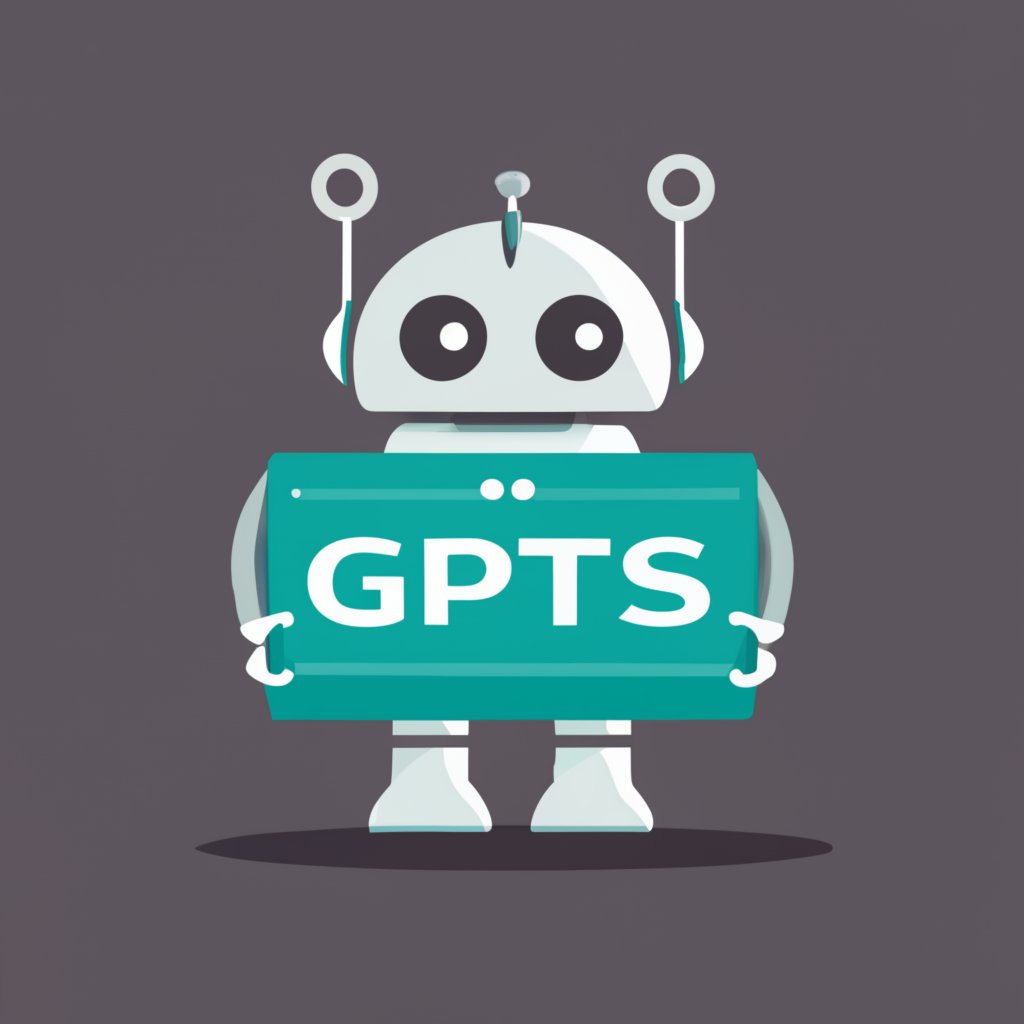
Discussion Post Writer
AI-powered tool for creating graduate-level discussion posts.

- Query Optimization
- API Design
- Authentication
- Data Serialization
- Web APIs
Common Questions About Backend API Python/Django/Django REST Framework
What is Django REST Framework used for?
Django REST Framework (DRF) is a powerful toolkit for building Web APIs in Django. It simplifies the creation of RESTful services by providing essential features such as serialization, authentication, and view handling, making it easier to expose your Django models as RESTful endpoints.
How do serializers work in Django REST Framework?
Serializers in Django REST Framework convert complex data types, such as Django querysets or model instances, into JSON. They also validate input data to ensure it conforms to the expected format before saving it to the database.
Can Django REST Framework handle authentication?
Yes, Django REST Framework offers built-in support for various authentication schemes, including token-based authentication, session authentication, and OAuth. You can customize or extend these mechanisms to suit your project's needs.
What are the key benefits of using Django REST Framework?
The key benefits include rapid development of APIs, extensive customization options, seamless integration with Django's ORM, and a rich ecosystem of third-party packages. DRF also provides comprehensive documentation and strong community support.
How do you optimize API performance in Django REST Framework?
To optimize API performance in Django REST Framework, you can use query optimization techniques like select_related and prefetch_related, enable caching, paginate large querysets, and use tools like Django Debug Toolbar to identify bottlenecks.