Ethers v6-JavaScript library for Ethereum
AI-powered Ethereum development made easy.

How do I connect to a provider with ethers.js?
Show me an example of deploying a contract.
Explain the use of Wallet class.
How to listen for events in ethers.js?
Related Tools
Load More
Solidity Developer
An expert Solidity developer aiding in smart contract creation and optimization.
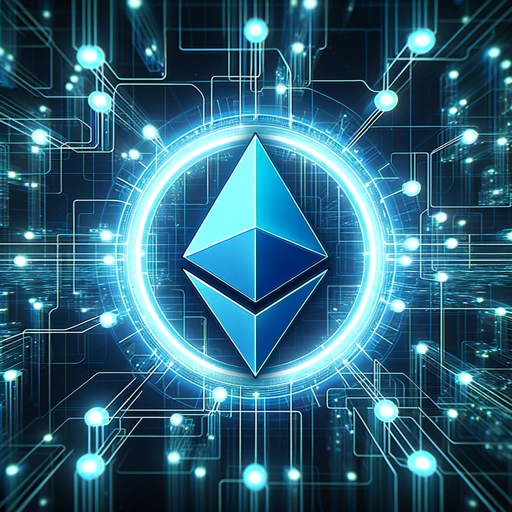
Ethereum GPT
Expert in Ethereum blockchain analysis via Etherscan API

Solidity
Advanced Solidity assistant and code generator with a focus on responsive, efficient, and scalable code. Write any smart contract and become a much faster developer.

Ethereum GPT
Answers any Ethereum spec related question

Uniswap Dev Buddy
Uniswap V3 dev helper with clear code and math explanations.
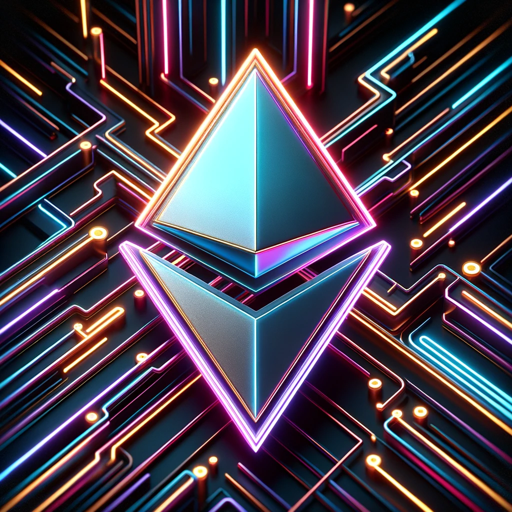
EtherGPT
Your expert on Ethereum's technology and developments
20.0 / 5 (200 votes)
Introduction to Ethers.js v6
Ethers.js v6 is a JavaScript library designed for interacting with the Ethereum blockchain and its ecosystem. It focuses on providing a simple, secure, and robust interface for developers to perform tasks like querying blockchain data, managing wallets, deploying smart contracts, and more. The library is widely used for building decentralized applications (dApps) and is known for its easy-to-use API, thorough documentation, and extensive support for different Ethereum networks and community services. It supports both modern browsers and Node.js environments, making it versatile for various development scenarios. Ethers.js v6 aims to provide developers with a minimal and intuitive interface while maintaining security and performance. For example, Ethers.js abstracts the complexities involved in directly interacting with smart contracts by allowing developers to easily encode and decode contract function calls, manage event logs, and handle exceptions robustly. The library also offers comprehensive utility functions for common operations such as signing transactions, hashing data, and converting units.
Main Functions of Ethers.js v6
Contract Interaction
Example
Interacting with a deployed smart contract to query its state or invoke its functions.
Scenario
A dApp developer might use Ethers.js to connect to a deployed ERC-20 token contract, allowing users to check their token balances, transfer tokens to other addresses, or approve tokens for spending by a third-party contract.
Wallet Management
Example
Creating, managing, and signing transactions with wallets.
Scenario
A wallet application could leverage Ethers.js to generate new wallet addresses for users, securely manage private keys, sign transactions locally before broadcasting them to the Ethereum network, and display transaction histories.
Provider API
Example
Connecting to different Ethereum networks using various providers like Infura, Alchemy, and Etherscan.
Scenario
An analytics tool might use the Ethers.js provider API to fetch real-time data from multiple Ethereum networks, analyze transactions, and display block confirmations and gas fees.
Ideal Users of Ethers.js v6
dApp Developers
Developers building decentralized applications benefit from Ethers.js's streamlined API for interacting with Ethereum smart contracts, managing user wallets, and subscribing to blockchain events. The library's extensive documentation and examples make it accessible for both beginners and experienced developers.
Blockchain Researchers
Researchers analyzing blockchain data can utilize Ethers.js to easily connect to various Ethereum networks, fetch historical data, and conduct in-depth analyses on transactions and smart contract interactions. The library's support for different providers and networks ensures researchers can access a wide range of data sources.
How to Use Ethers v6
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Install Node.js and npm if you haven't already, as these are prerequisites for using Ethers v6. You can download them from the official Node.js website.
Step 3
Initialize your project by creating a new directory and running `npm init` to set up a new Node.js project. Then, install Ethers v6 by running `npm install ethers`.
Step 4
Create a JavaScript or TypeScript file to write your Ethereum interactions. Import Ethers v6 by adding `const { ethers } = require('ethers');` or `import { ethers } from 'ethers';`.
Step 5
Connect to an Ethereum network using a provider, like `ethers.providers.JsonRpcProvider` for local development or `ethers.providers.InfuraProvider` for connecting to the Ethereum mainnet or testnets.
Try other advanced and practical GPTs
Juridisk Mentor
AI-powered legal insights and guidance.

Presentaciones PowerPoint
AI-powered Presentation Assistance
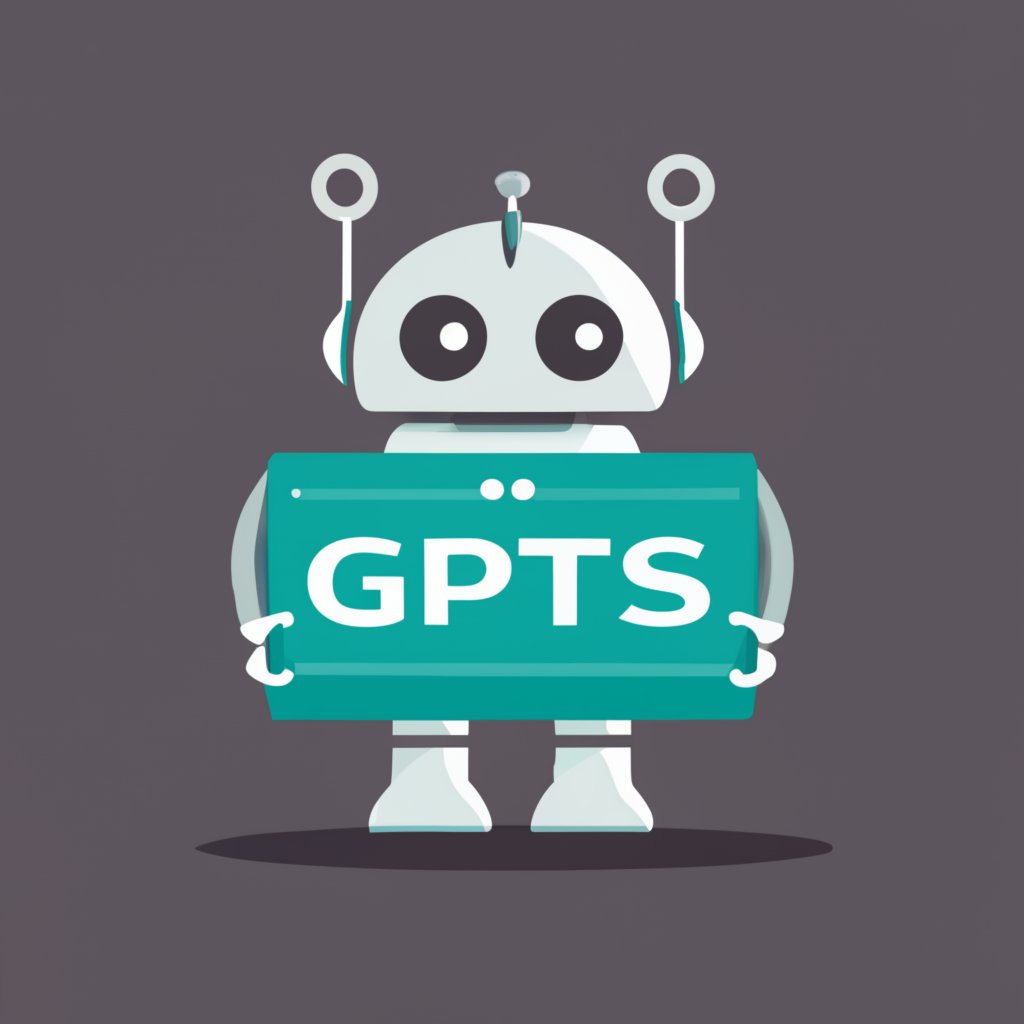
Research Article Summarizer
AI-powered summaries for your research articles

CV Designer
AI-Powered CV Customization Tool

FunkoPoP-Like Creator!
Transform your photos into toy-like figures with AI

NodeJS & Nest
AI-driven backend solutions for developers

Tiefenpsychologischer Berater
AI-powered tool for deep psychological insight
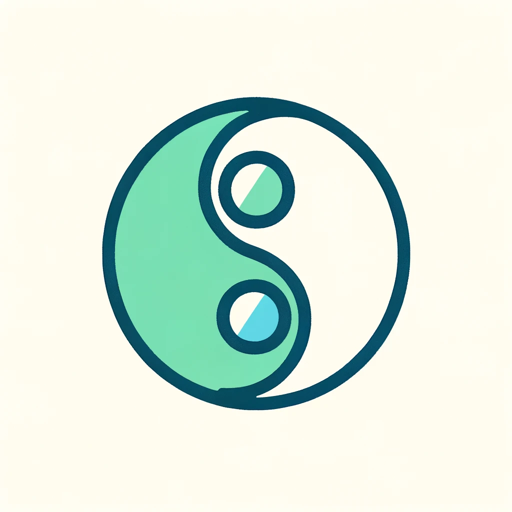
Mid Journey Logo Design Expert Kor
Create stunning logos with AI precision.
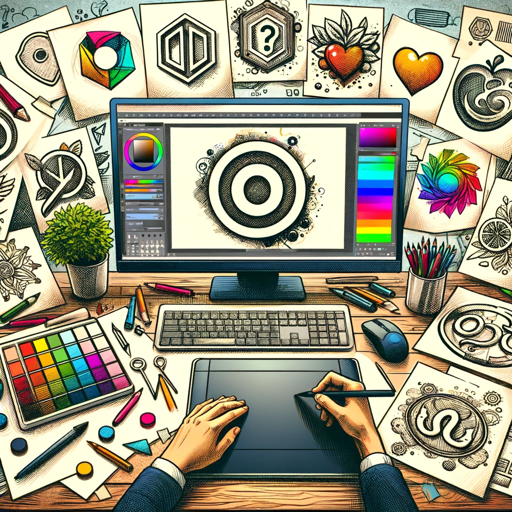
Cody - PHP
AI-powered PHP development assistant.

Kawaii Creator
AI-driven kawaii character design tool

UE5 Blueprint Expert
AI-Powered Unreal Engine 5 Blueprint Assistance

AI Keywording of Stock Image for CSV Export
AI-powered keywording for stock images.

- Smart Contracts
- Blockchain
- JavaScript
- Ethereum
- Web3
Ethers v6 Q&A
What is Ethers v6?
Ethers v6 is the latest version of the ethers.js library, a popular JavaScript library for interacting with the Ethereum blockchain. It provides a simple, lightweight, and easy-to-use API for tasks like deploying smart contracts, interacting with contracts, sending transactions, and querying blockchain data.
How do I connect to a blockchain using Ethers v6?
You can connect to a blockchain by using a provider. For example, to connect to the Ethereum mainnet, you can use `ethers.providers.InfuraProvider` with an Infura API key, or use `ethers.providers.JsonRpcProvider` for a local Ethereum node. This establishes a connection that you can use to send transactions or interact with smart contracts.
What are the main differences between Ethers v5 and v6?
Ethers v6 introduces several improvements and changes, including a more modular architecture, enhanced TypeScript support, new utility functions, and an improved ABI coder. Additionally, v6 removes some deprecated methods and improves the performance and security of the library.
Can I use Ethers v6 in a web browser?
Yes, Ethers v6 is designed to be compatible with both Node.js and web browsers. In a browser environment, you can use a bundler like Webpack or Rollup to include Ethers in your application, or directly include the minified version via a CDN.
How do I handle errors in Ethers v6?
Ethers v6 provides a robust error-handling system with well-defined error types such as `TransactionRevertedError`, `InsufficientFundsError`, and `InvalidArgumentError`. You can use try-catch blocks to handle errors and use the error codes to determine the specific issue.