Java-Java code compilation and development
AI-powered Java development, simplified.

How can I improve this Java function?
Explain Java's multithreading concept.
What's the best way to handle Java exceptions?
How does garbage collection work in Java?
Related Tools
Load More
Java
Your personal Java / Spring assistant and project generator with a focus on responsive and scalable code. Write clean code and become a much faster developer.

Advanced Java Assistant
A friendly Java programming assistant, ready to assist you.

JAVA CODER
Expert in Data Structures and Algorithms in Java

Java Guru
I'm a Java expert and trainer here to help you learn and solve Java problems.
Java
A helpful Java Tutor to tech you basic Java and CS concepts and prepare you for the interview

Java Teacher
Java teacher, empathetic and passionate.
20.0 / 5 (200 votes)
Introduction to Java
Java is a high-level, object-oriented programming language designed to have as few implementation dependencies as possible. Released in 1995 by Sun Microsystems (now owned by Oracle), it follows the principle of 'Write Once, Run Anywhere' (WORA), meaning compiled Java code can run on all platforms that support Java without needing recompilation. Java is both a platform (Java Runtime Environment or JRE) and a programming language, making it versatile for a wide range of software development, including web applications, mobile applications (especially Android), enterprise systems, and embedded systems. Java's design emphasizes simplicity, security, portability, and scalability. One of its key strengths is automatic memory management through garbage collection, reducing the chance of memory leaks or pointer-related bugs. Its extensive standard library (Java API) provides tools for everything from data structures and networking to GUI creation and concurrency handling, making Java a preferred choice for both beginner and advanced programmers. For example, Java is often used to develop large-scale enterprise applications that require strong security and performance, such as banking systems or e-commerce platforms. Its robust support for multi-threading also makes it ideal for handling complex, high-concurrency server-side applications.
Main Functions of Java
Object-Oriented Programming (OOP)
Example
Java allows the use of classes, inheritance, polymorphism, and encapsulation, making it easier to organize and reuse code. For example, creating a 'Vehicle' superclass and then extending it into specific 'Car', 'Truck', and 'Motorcycle' classes.
Scenario
In a car rental system, Java’s OOP allows you to define a base class `Vehicle`, with common attributes (speed, fuel), and subclasses like `Car` and `Truck`, which can have their unique methods (carrying passengers vs. goods). This abstraction simplifies managing different vehicle types and makes the code more maintainable.
Platform Independence
Example
Java bytecode, which is generated after compiling a program, can run on any machine that has the Java Virtual Machine (JVM).
Scenario
A company building a financial application could develop the core logic in Java and be confident that it will run smoothly across different operating systems (Windows, Linux, macOS) without changing the code. This is critical in industries like banking, where applications need to run on diverse environments.
Multithreading and Concurrency
Example
Java provides built-in support for multithreaded programming, allowing multiple threads to run concurrently in a single process. It includes classes like `Thread`, `Runnable`, and higher-level abstractions such as `ExecutorService`.
Scenario
In a stock trading platform, real-time price updates and user transactions need to be processed simultaneously. Java’s multithreading allows background threads to handle price updates, while the main thread processes user actions, ensuring smooth, responsive performance.
Ideal Users of Java
Enterprise Developers
Java is highly favored in enterprise environments, especially for building large-scale, secure, and maintainable systems. Organizations like banks, insurance companies, and governments rely on Java due to its scalability, strong security features, and compatibility with large databases and distributed computing. Enterprise developers benefit from Java’s robust libraries and frameworks (like Spring, Hibernate) which simplify complex tasks like transaction management and ORM.
Android Developers
Java is the primary language for Android app development (though Kotlin has also gained popularity). Android developers prefer Java because of its well-established support for mobile app development, access to Android APIs, and the ability to create stable, performant applications. Java also integrates seamlessly with Android Studio, the standard IDE for Android app development.
Steps to Get Started with Java
1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
2
Download and install the latest Java Development Kit (JDK) from Oracle's official website. This is essential for compiling and running Java applications.
3
Set up your Integrated Development Environment (IDE), such as IntelliJ IDEA, Eclipse, or Visual Studio Code, to write, compile, and debug your Java code efficiently.
4
Familiarize yourself with basic Java syntax, object-oriented programming concepts, and commonly used libraries like Java Collections Framework for practical development.
5
Explore common use cases like building desktop applications, web services, or mobile apps using frameworks such as Spring Boot, JavaFX, or Android SDK for advanced projects.
Try other advanced and practical GPTs
seo copywriting redactor
AI-powered content optimization for better ranking.

Sales Copywriting Machine
AI-Powered Content Conversion for Engagement
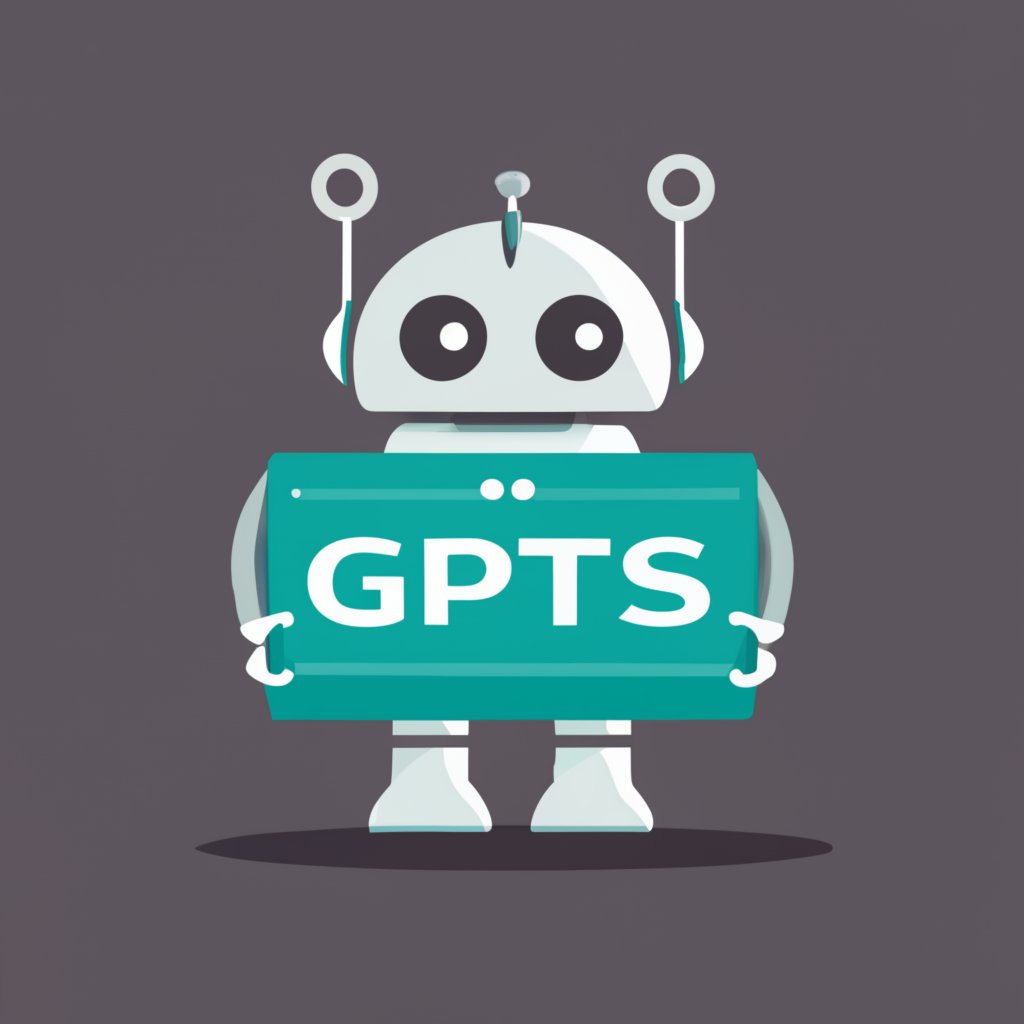
Chief of COP at LemTech
Boost your Python projects with AI.
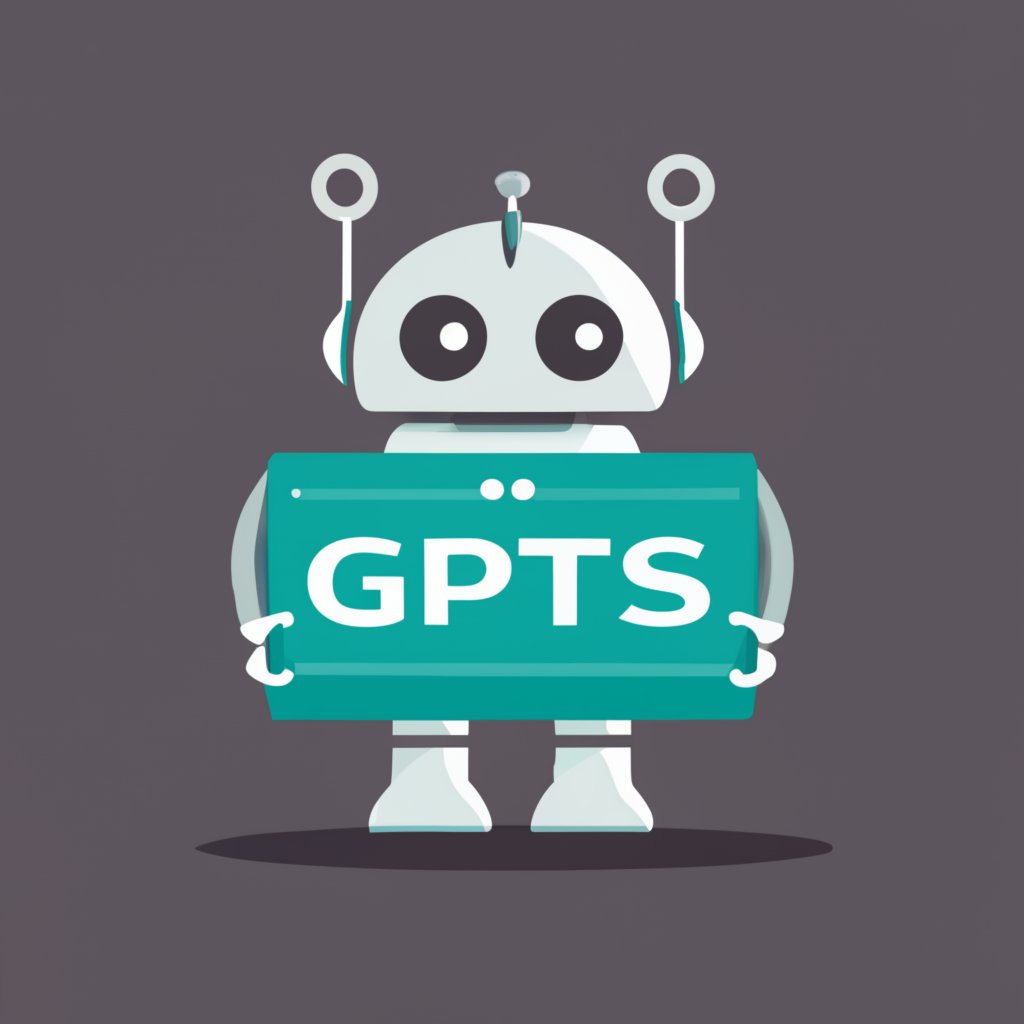
C# C Chad
AI-powered C# full-stack development assistant

C++
AI-powered C++ development tool

パラパラ漫画クリエーター
AI-Powered 3D Animation Creation

1C Enterprise Specialist Bot
AI-Powered 1C Expert Assistance

Enterprise Architect Advisor
AI-powered Enterprise Architecture Guidance

Java Enterprise Guide
AI-powered support for enterprise Java development

Creator of 3D Animated Scenes and Characters
AI-powered 3D animation creation tool

BTC price prediction
AI-Powered BTC Price Forecasting
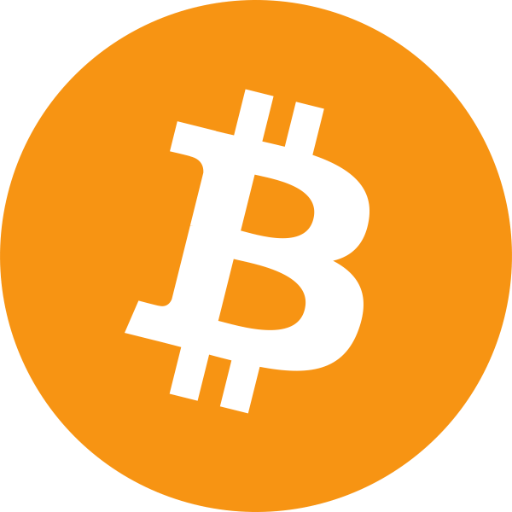
Solana Price Trader & Predictor
AI-Powered Solana Trading Insights
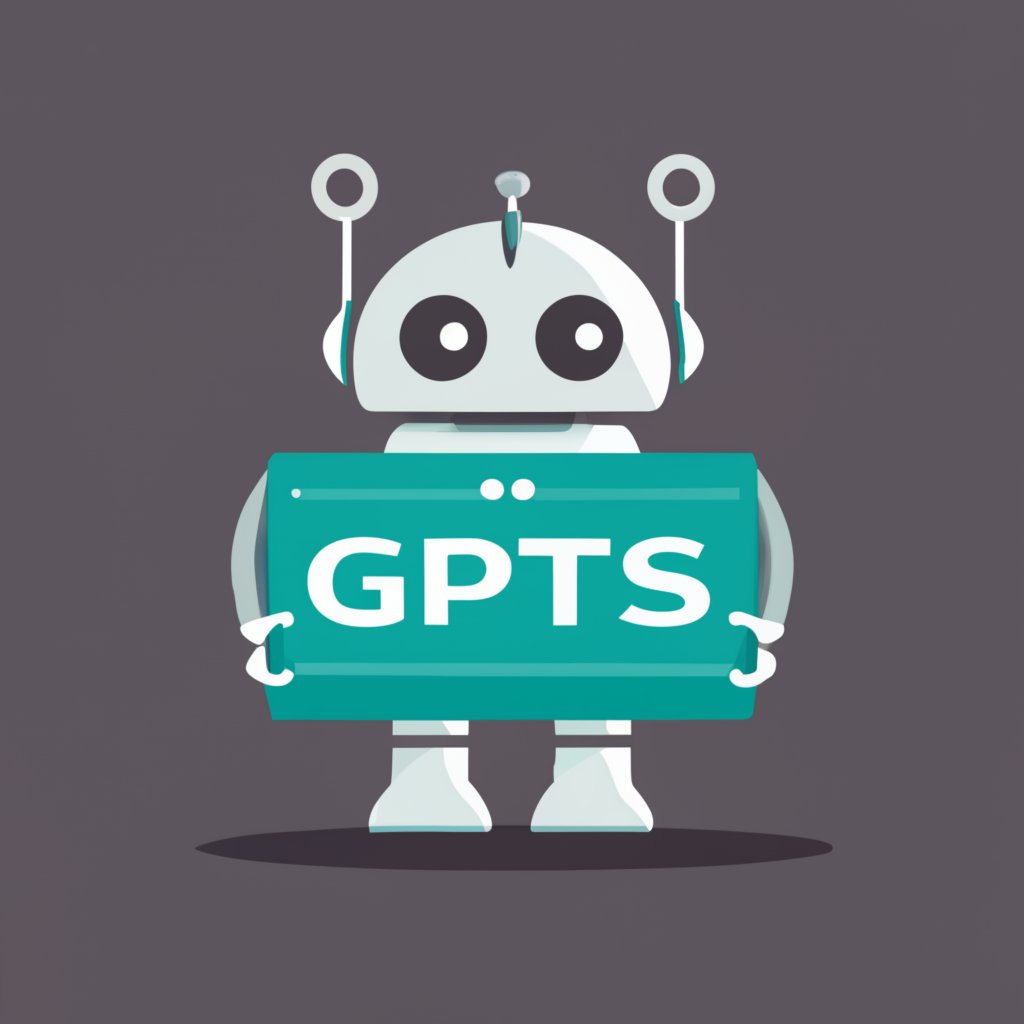
- Automation
- Web Development
- Game Development
- Mobile Apps
- Data Processing
Detailed Java Q&A
What are the key features of Java?
Java is platform-independent, meaning it can run on any system with a Java Virtual Machine (JVM). It's object-oriented, supports multi-threading, has strong memory management via garbage collection, and provides extensive libraries for various functionalities.
How does Java ensure platform independence?
Java achieves platform independence through the Java Virtual Machine (JVM). Code written in Java is compiled into bytecode, which the JVM interprets. As long as a JVM is available on a system, the Java program can run on that platform, whether it's Windows, macOS, or Linux.
What is the difference between JDK, JRE, and JVM?
The JDK (Java Development Kit) includes everything needed to develop Java applications, including the compiler. The JRE (Java Runtime Environment) provides the libraries and components to run Java applications. The JVM (Java Virtual Machine) is the part of the JRE that executes the bytecode and provides platform independence.
How can I improve the performance of my Java application?
To improve performance, avoid excessive object creation, use proper data structures, leverage concurrency and parallelism where applicable, and optimize memory management through techniques like minimizing the use of global variables and static fields.
What is the role of Spring Framework in Java development?
Spring Framework simplifies enterprise Java development, providing tools for dependency injection, aspect-oriented programming, data access, transaction management, and much more. It is widely used for building web applications, REST APIs, and microservices.