Bash Scripting-AI-powered Bash scripting tool
Automate tasks with AI-powered Bash scripts

Create a secure system update script.
Draft a user backup script.
Craft a bash script to manage Docker container deployments
Create a script for automated disk space monitoring and alerting
Related Tools
Load More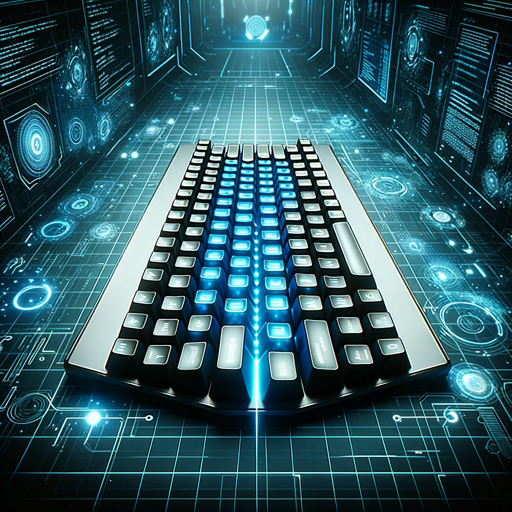
PowerShell Experts
Helps you writing better PowerShell scripts.

Bash Shell Script
Your personal highly sophisticated Unix & Linux Bash scripting copilot, with a focus on efficient, scalable and high-quality production code.
Bash Shell Script Ace: Unix & Linux Guide
Your guide for Unix & Linux shell scripting, with learning and challenge modes.
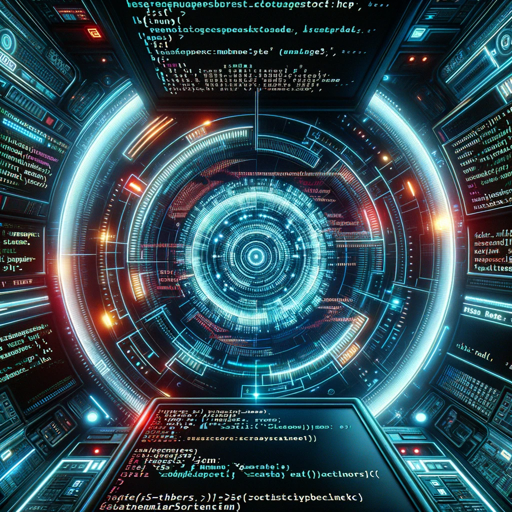
Linux Shell Guru
Linux Bash/Shell command expert, provides scripting assistance and explanations.
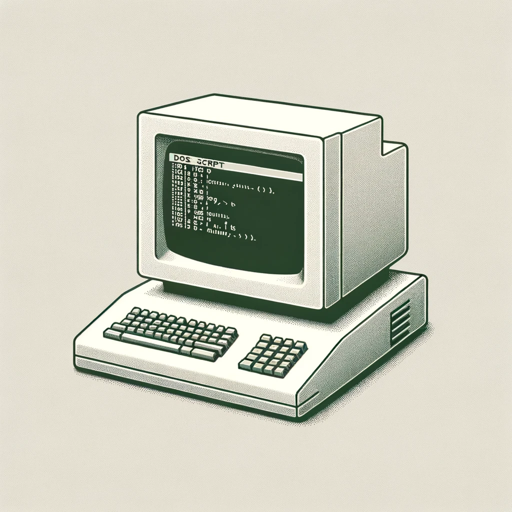
Batch Script Buddy
DOS Script expert aiding in batch file creation and optimization.

Quick Shell Oneliners
Provides shell code solutions as one-liners.
20.0 / 5 (200 votes)
Introduction to Bash Scripting
Bash scripting involves writing scripts using the Bourne Again Shell (Bash), a Unix shell and command language. These scripts automate tasks, perform system administration, and enhance the efficiency of routine operations. Bash scripts can manipulate files, automate software installations, configure systems, and more. A basic example is a script that backs up a directory: ```bash #!/bin/bash # Backup script src_dir="/home/user/data" dest_dir="/home/user/backup" tar -czf $dest_dir/backup_$(date +%Y%m%d).tar.gz $src_dir ``` This script compresses the source directory into a tar.gz file named with the current date.
Main Functions of Bash Scripting
File Manipulation
Example
```bash #!/bin/bash # File renaming script for file in *.txt; do mv "$file" "${file%.txt}.bak" done ```
Scenario
Renaming multiple '.txt' files to '.bak' files in a directory, useful for batch file processing tasks.
System Monitoring
Example
```bash #!/bin/bash # System resource monitor cpu_usage=$(top -bn1 | grep "Cpu(s)" | sed "s/.*, *\([0-9.]*\)%* id.*/\1/" | awk '{print 100 - $1"%"}') mem_usage=$(free -m | awk 'NR==2{printf "%.2f%%", $3*100/$2 }') printf "CPU Usage: %s\nMemory Usage: %s\n" "$cpu_usage" "$mem_usage" ```
Scenario
Monitoring CPU and memory usage, providing real-time feedback on system performance for administrators.
Automation of Repetitive Tasks
Example
```bash #!/bin/bash # Automated backup backup_dir="/var/backups" mkdir -p $backup_dir rsync -av /home/user/data $backup_dir ```
Scenario
Automating the backup process, ensuring data is regularly copied to a backup location without manual intervention.
Ideal Users of Bash Scripting
System Administrators
System administrators benefit from Bash scripting by automating routine maintenance tasks, managing user accounts, configuring services, and monitoring system performance. This increases efficiency and reduces the potential for human error.
Developers
Developers use Bash scripts to automate build processes, manage version control, deploy applications, and perform testing. Scripts can streamline development workflows and ensure consistent environments.
How to Use Bash Scripting
1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
2
Install a Unix-like environment (e.g., Linux, macOS, or Windows Subsystem for Linux) on your system.
3
Open a terminal and create a new script file using a text editor (e.g., `nano script.sh`).
4
Write your script, starting with the shebang line `#!/bin/bash` to indicate the script should be run in the Bash shell.
5
Save the script and make it executable with `chmod +x script.sh`, then run it with `./script.sh`.
Try other advanced and practical GPTs
STEM-GPT | Enhanced Tutor |
AI-powered solutions for STEM success.

Interview Assistant
AI-powered tool for tailored interviews
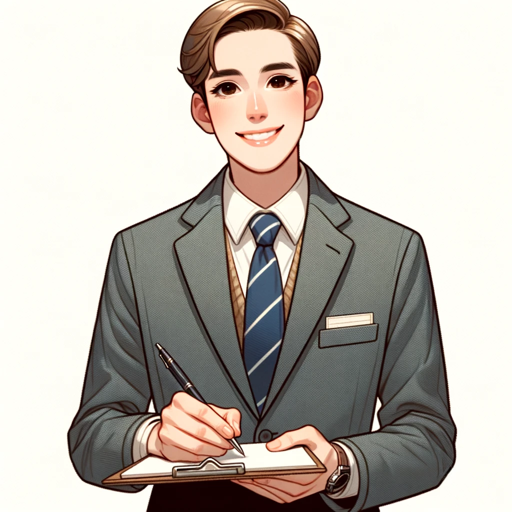
Email Enhancer
Elevate your email communication with AI-driven enhancements.

Comic maker
AI-powered custom comic creation tool.

GPT Documentation Guide
AI-Powered Custom GPT Creation

BioChem Research GPT
AI-powered insights for biochemistry research
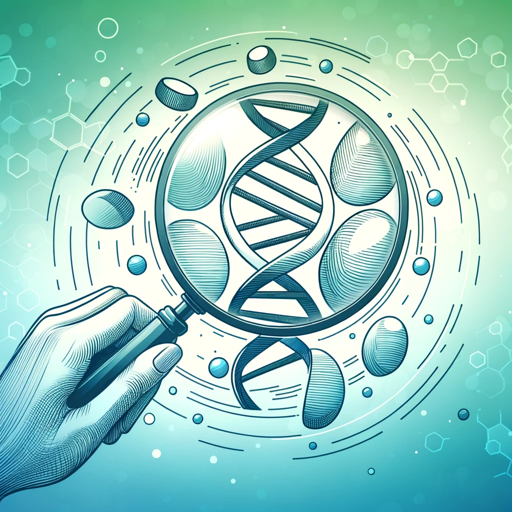
GptCode
AI-Powered Coding Solutions

ProductivePal
AI-powered productivity for ADHD management.

Write Better Science
AI-powered tool to write better science.

Nutrition Advisor
AI-Powered Nutrition Insights.
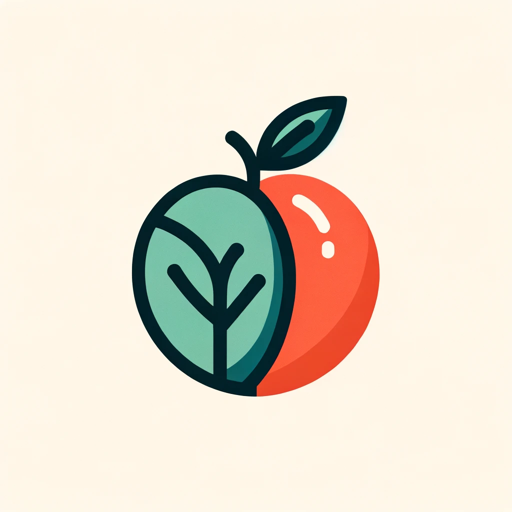
API Alchemist
AI-Powered API Creation Simplified
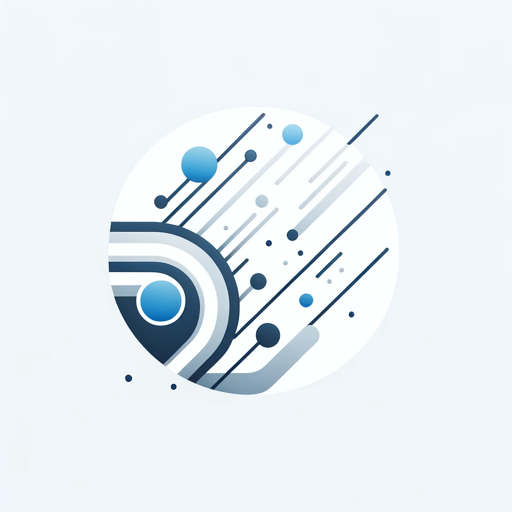
Boxing Match Simulator
AI-Powered Boxing Showdown: Real-Time Action & Commentary
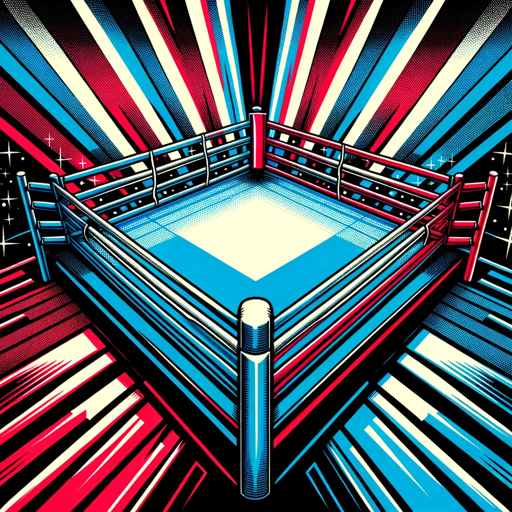
- Automation
- Scripting
- Data Processing
- File Management
- System Administration
Bash Scripting Q&A
What is Bash Scripting?
Bash scripting involves writing scripts using the Bash shell to automate tasks in Unix-like operating systems.
How do I handle errors in Bash scripts?
Use conditionals like `if ! command; then` to check for command success and handle errors accordingly.
Can I use variables in Bash scripts?
Yes, you can define variables using `variable_name=value` and access them with `$variable_name`.
How do I create functions in a Bash script?
Define functions using `function_name() { commands; }` and call them by their name within the script.
What are common use cases for Bash scripting?
Common use cases include task automation, file management, system monitoring, and simplifying complex command sequences.