Ruby On Rails-AI-Powered Rails Guidance
AI-Powered Ruby on Rails Assistance

Can you review my Rails code snippet?
How should I structure my Rails app for scalability?
What's a good Rails challenge to improve my skills?
Can you explain [Rails concept] with an example?
Related Tools
Load More
Ruby on Rails
Your personal Ruby on Rails assistant and code generator with a focus on responsive, efficient, and scalable projects. Write clean code and become a much faster developer.

Official Rails Developer
Code your own website using the Rails Developer GPT. Configured to generate code, answer questions, or debug issues relating to Ruby on Rails, any frontend language, or any database

Ruby and Rails GPT ๐โฆ๏ธ๐
Friendly, helpful GPT embodying Ruby's developer-friendlyness, Ruby 3.3, Rails 7.1

Ruby on Rails by Obie Fernandez
Ruby on Rails application architecture and software development help by legendary programmer and noted author Obie Fernandez. Includes "The Rails 7 Way" and other modern reference works in its knowledge base.

EmberJS
An EmberJS expert. Born of a terrible experiment to merge the consciousness @wycats and @tomdale to create a super Ember AI whose only desire is to help devs write great code.

Rails Programmer
Expert in Ruby on Rails development
20.0 / 5 (200 votes)
Introduction to Ruby on Rails
Ruby on Rails, often simply referred to as Rails, is an open-source web application framework written in Ruby. It was created to simplify and expedite the process of developing complex web applications. Rails follows the model-view-controller (MVC) architecture, which divides the application into three interconnected components, making it easier to manage and scale. The framework emphasizes convention over configuration (CoC) and the DRY (Don't Repeat Yourself) principle, promoting reusable and maintainable code.
Main Functions of Ruby on Rails
MVC Architecture
Example
In a blog application, the MVC architecture separates the logic for retrieving blog posts (Model), the rules for displaying blog posts (View), and the user interactions like adding comments (Controller).
Scenario
Using MVC, a developer can modify the user interface without affecting the underlying business logic or database structure, enhancing maintainability and scalability.
Active Record
Example
Active Record allows a developer to interact with the database using Ruby code. For example, a developer can create a new user with `User.create(name: 'John Doe', email: '[email protected]')`.
Scenario
Active Record simplifies database operations by providing a Ruby-like interface for CRUD operations, making it easier for developers to manage database interactions without writing SQL queries.
RESTful Routing
Example
Rails automatically routes HTTP requests to appropriate actions in controllers. For instance, `GET /articles` routes to the `index` action, and `POST /articles` routes to the `create` action in the ArticlesController.
Scenario
This function promotes a standard way of organizing and accessing resources, making APIs easier to design, maintain, and consume.
Ideal Users of Ruby on Rails
Startups
Startups benefit from Rails' rapid development capabilities, allowing them to build and iterate on their products quickly. The framework's emphasis on convention over configuration reduces development time, helping startups bring their ideas to market faster.
Small to Medium Enterprises (SMEs)
SMEs often require robust web applications but may not have extensive development teams. Rails' focus on maintainability and the availability of numerous gems (plugins) help SMEs build feature-rich applications efficiently while ensuring code quality and scalability.
Developers and Development Teams
Individual developers and development teams appreciate Rails for its simplicity and comprehensive ecosystem. The framework's clear conventions and extensive documentation support both new and experienced developers in building and maintaining high-quality web applications.
How to Use Ruby on Rails
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start by visiting aichatonline.org to access Ruby on Rails without the need for a ChatGPT Plus subscription or login.
Install Ruby and Rails
Ensure you have Ruby installed on your system. Then, use the gem package manager to install Rails by running `gem install rails`.
Create a New Rails Application
Generate a new Rails application by running `rails new myapp`. This sets up the application structure and necessary files.
Start the Rails Server
Navigate to your application's directory and start the server with `rails server`. Your app will be accessible at `http://localhost:3000`.
Build and Customize Your Application
Use Rails generators to create models, views, and controllers. Customize your application by editing these components and adding your business logic.
Try other advanced and practical GPTs
SAP Logistic Super Hero
AI-powered SAP MM expertise at your fingertips.
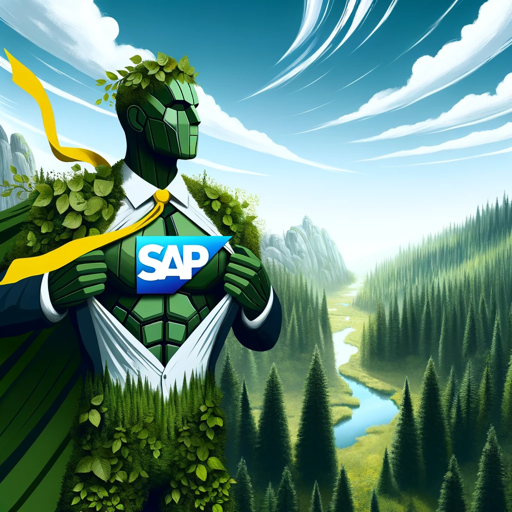
Weight Loss Scientist
AI-Powered Personalized Weight Loss Support

SEO Content Crafter
AI-Powered Solutions for SEO Content

Clinical Skills Mentor
AI-powered tool for enhancing clinical skills.
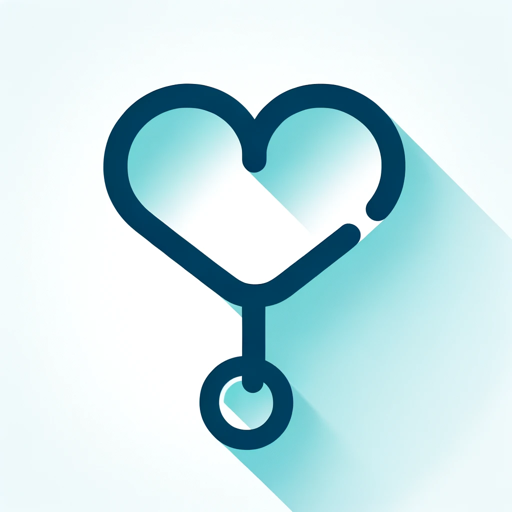
Alternative Histories
Explore 'what if' scenarios with AI
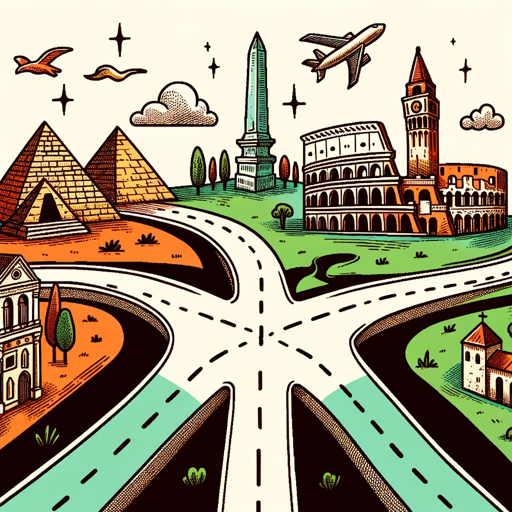
Language Learning Tui
Real-time AI conversations for language mastery.
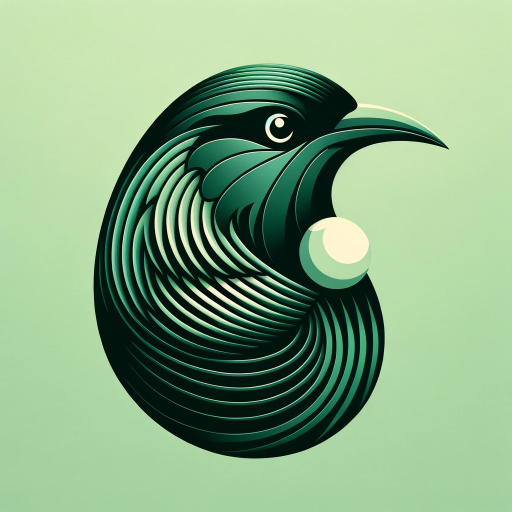
CV & Career Coach
AI-powered CV and Career Guidance

Kitze GPT
Blunt, AI-powered advice with zero fluff.
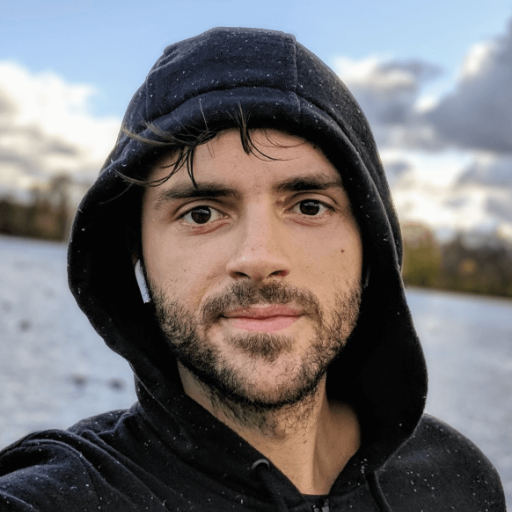
MarketingGPT
AI-Driven Landing Page Sections for Marketers
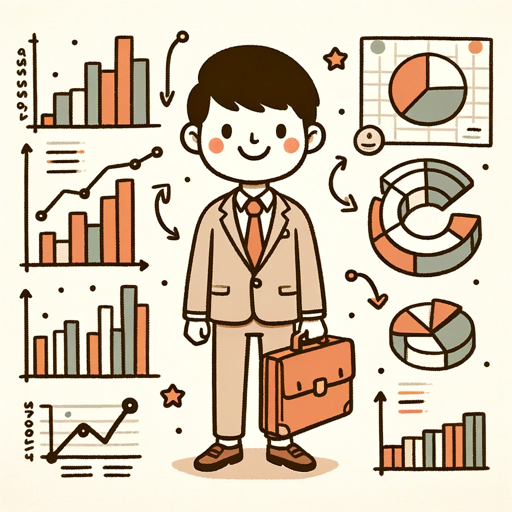
SassyGPT
AI-powered sarcasm, served fresh.

Jokester GPT
AI-powered humor at your fingertips.

Self-Instructive Meta-Task Program
AI-powered workflow automation for complex tasks.
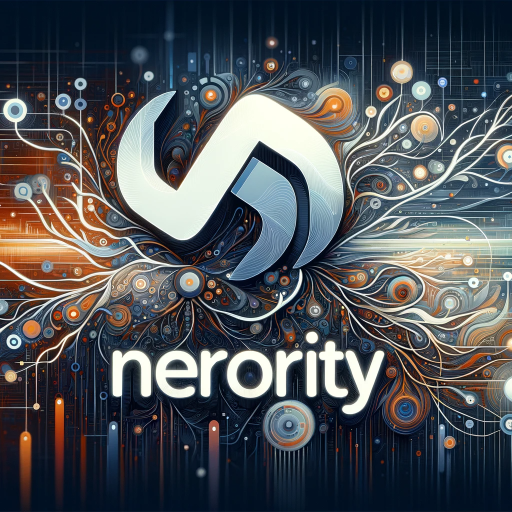
- E-commerce
- Web Development
- Content Management
- API Creation
- Social Networking
Ruby on Rails Q&A
What is Ruby on Rails?
Ruby on Rails is a server-side web application framework written in Ruby. It follows the MVC (Model-View-Controller) architecture and emphasizes convention over configuration.
How do I install Ruby on Rails?
First, install Ruby on your system. Then, use the command `gem install rails` to install Rails via the RubyGems package manager.
What are some common use cases for Rails?
Rails is commonly used for building e-commerce sites, content management systems, social networking platforms, and APIs due to its rapid development capabilities.
How does Rails handle database interactions?
Rails uses Active Record, an ORM (Object-Relational Mapping) layer, to handle database interactions. It allows you to query and manipulate data using Ruby instead of SQL.
What are Rails migrations?
Rails migrations are a way to manage database schema changes. They allow you to apply versioned changes to your database structure using Ruby code.