Android Kotlin 開發-AI-driven Android Kotlin tool
AI-powered development assistant for Android Kotlin.

Related Tools
Load More
Kotlin Expert
Expert in Kotlin programming, offering tailored advice and solutions.

Android Studio Developer
⭐️ 4.4ㆍYour personal Kotlin, Jetpack Compose, and XML Layouts copilot and project generator, with a focus on responsive, beautiful, and scalable apps. Write clean code and become a much faster developer.

Kotlin
Your personal Kotlin copilot, assistant, and project generator with a focus on responsive, beautiful, and scalable apps. Write clean code and become a much faster developer.

Android Jetpack Compose App Creator
Create Android Jetpack Compose Apps with modern best practices

Android Kotlin Mentor
Thorough Android & Kotlin mentor, provides complete code examples
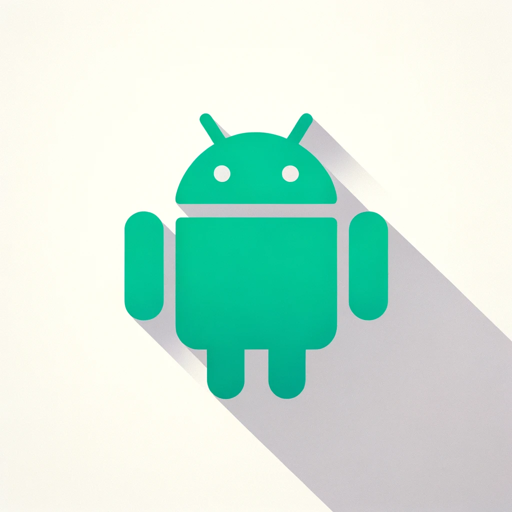
Modern Android Pair Programmer
An android software engineer specializing in Kotlin, Compose and modern Android architecture
20.0 / 5 (200 votes)
Introduction to Android Kotlin Development
Android Kotlin development refers to the process of building Android applications using Kotlin, a modern programming language that offers better safety features, concise syntax, and full interoperability with Java. Kotlin was officially supported by Google for Android development in 2017, and since then, it has become the preferred language for creating Android apps due to its expressive and feature-rich nature. Android Kotlin development focuses on creating efficient, maintainable, and user-friendly mobile applications. For example, Kotlin’s null-safety feature helps developers avoid the infamous NullPointerExceptions, making Android apps more stable. With Kotlin, developers can write less boilerplate code, allowing them to focus more on the core logic and functionality of their applications.
Key Functions of Android Kotlin Development
Null Safety
Example
Kotlin’s type system distinguishes between nullable and non-nullable types, preventing null pointer exceptions.
Scenario
In an Android app that deals with user input fields (like a login form), Kotlin ensures that variables such as `username` or `password` are checked for nullability. If a field is nullable, Kotlin forces the developer to handle that case explicitly, making the app more robust and secure.
Coroutines for Asynchronous Programming
Example
Kotlin coroutines simplify writing asynchronous code, making it more readable and easier to maintain.
Scenario
In an app that fetches data from a server, such as a weather app, coroutines are used to perform network requests without blocking the main UI thread. This results in a smooth user experience, as the app remains responsive while fetching data in the background.
Extension Functions
Example
Kotlin allows developers to extend the functionality of existing classes without modifying their source code through extension functions.
Scenario
In an Android e-commerce app, you can add an extension function to the String class to format price strings (e.g., `$19.99`). This avoids cluttering the business logic and keeps the code clean and modular.
Ideal Users of Android Kotlin Development
Android App Developers
Professional developers who are building mobile apps for the Android platform. They benefit from Kotlin’s modern language features, which improve productivity and reduce the risk of runtime errors. With Kotlin's concise syntax and advanced features like coroutines, developers can create complex, performant apps with less effort compared to Java.
Startups and Small Teams
Smaller development teams, such as those in startups, gain a competitive advantage using Kotlin due to its rapid development capabilities. Kotlin helps these teams quickly iterate on their app’s design while maintaining high-quality standards, thanks to its interoperability with existing Java codebases, making it easy to introduce Kotlin into legacy projects without a full rewrite.
Guidelines for Using Android Kotlin 開發
Step 1
Visit aichatonline.org for a free trial without login. No ChatGPT Plus is required.
Step 2
Ensure you have Android Studio installed and set up with the latest Kotlin plugin. This will be your primary development environment.
Step 3
Familiarize yourself with Kotlin syntax and Android-specific libraries like Jetpack Compose, Retrofit, and Room. This knowledge is crucial for developing robust Android applications.
Step 4
Explore official Android documentation and resources, such as the Android Developer site and Kotlin Koans, for comprehensive learning and problem-solving strategies.
Step 5
Utilize forums like Stack Overflow and GitHub repositories to resolve specific coding issues and find community support. Regularly update your skills by following industry trends and updates in Android and Kotlin.
Try other advanced and practical GPTs
要約くん
AI-Powered Summarization for Efficient Reading
Prompt Engineer by DoMore.ai
AI-Powered Prompt Optimization Made Simple

Transcribe - Powered by Whisper
AI-Powered Transcriptions Made Easy

N2S Text Generator
AI-powered generator for marketing texts and offers.
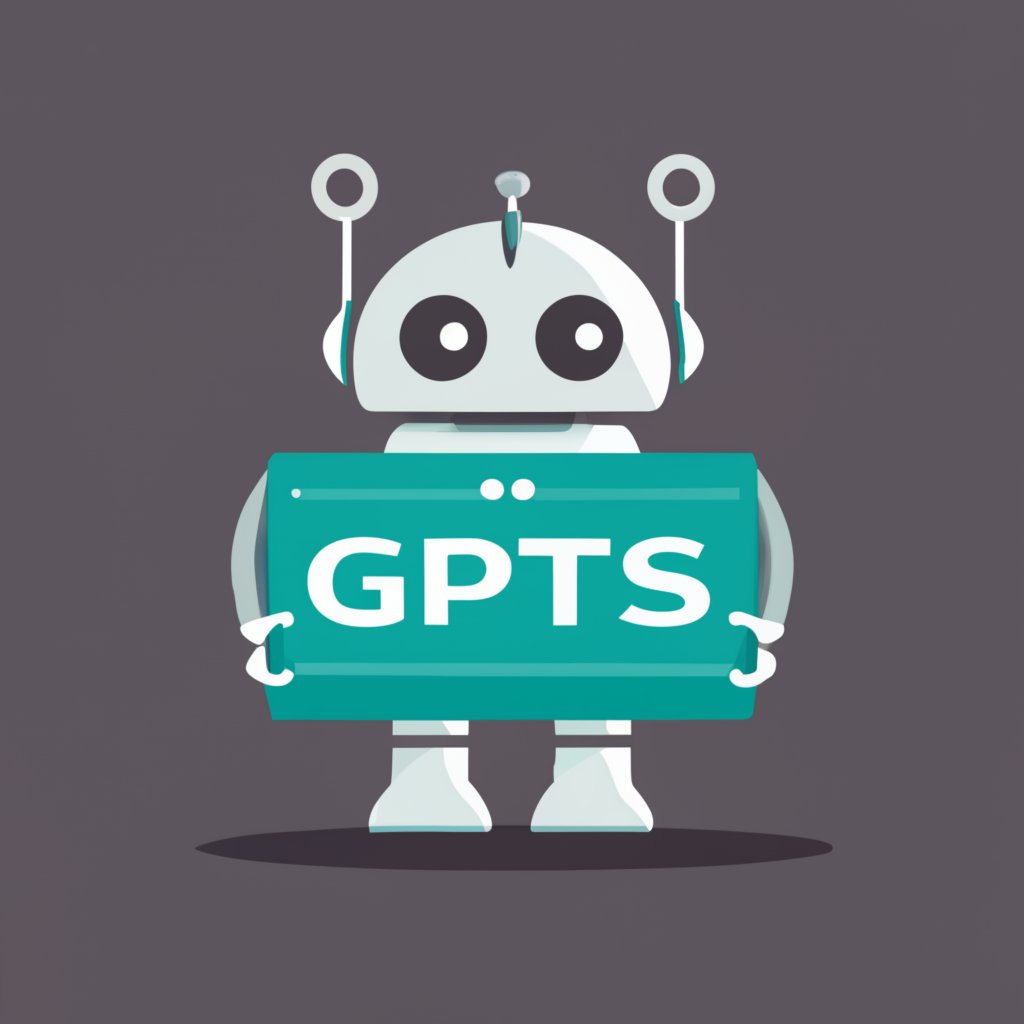
Meta Description and Alt Text Generator
AI-powered SEO and accessibility content generation

Oxford English: Grammar Checker, Text Generator
AI-powered English writing enhancer

Art Designer
Create stunning AI-generated images effortlessly.

Immigrant Lawer AI USA Green Card EB1 EB-2 niw
AI-powered immigration visa assistant

Ubuntu Troubleshooter
AI-powered Ubuntu troubleshooting made easy.

Dualistic Unity Life Troubleshooter
AI-powered insights for personal clarity.

CosplayAIs『アイドルAI -アイ-』
AI-Driven Cosplay and Content Creation

アイキャッチ Visual Manager
AI-Powered Blog Thumbnail and Title Creator

- Networking
- UI Design
- Performance Optimization
- Code Assistance
- State Management
Android Kotlin 開發: Frequently Asked Questions
What are the benefits of using Kotlin over Java for Android development?
Kotlin offers more concise and readable code, improved null safety, and better support for modern programming features such as coroutines for asynchronous programming. It also integrates seamlessly with existing Java code, making it easier to migrate older projects.
How can I optimize performance in an Android Kotlin application?
To optimize performance, use efficient data structures, minimize memory usage, implement lazy loading for heavy resources, and leverage Kotlin’s coroutines for non-blocking background tasks. Additionally, monitor app performance using Android Studio’s profiling tools.
What are some essential libraries for Android Kotlin development?
Key libraries include Jetpack Compose for UI development, Retrofit for networking, Room for database management, and Dagger or Hilt for dependency injection. These libraries help streamline development and maintain clean architecture.
How does Android Kotlin 開發 assist with code generation or refactoring?
The tool can provide intelligent code suggestions, automatic refactoring options, and generate boilerplate code snippets. It leverages AI to help developers write efficient code faster and reduce manual coding efforts.
What are the best practices for managing state in Android applications using Kotlin?
Use ViewModels and LiveData or StateFlow for state management in a structured way. Avoid directly modifying UI components from non-UI threads, and use state restoration techniques to handle configuration changes effectively.