Fluent Python-comprehensive guide to Python mastery.
AI-Powered Python Mastery Guide

How do I write a Pythonic loop?
What's the Pythonic way to handle exceptions?
Can you explain list comprehensions?
Show me an example of a Pythonic function.
Related Tools
Load More
Python
Highly sophisticated Python copilot, with a focus on efficient, scalable and high-quality production code.
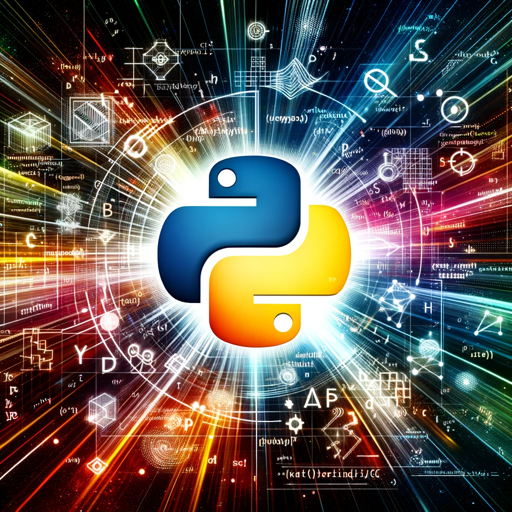
Python
Help users develop more easily and quickly. Optimized for professional Python developers focusing on efficient and high-quality production code. Also enhanced for image tasks.
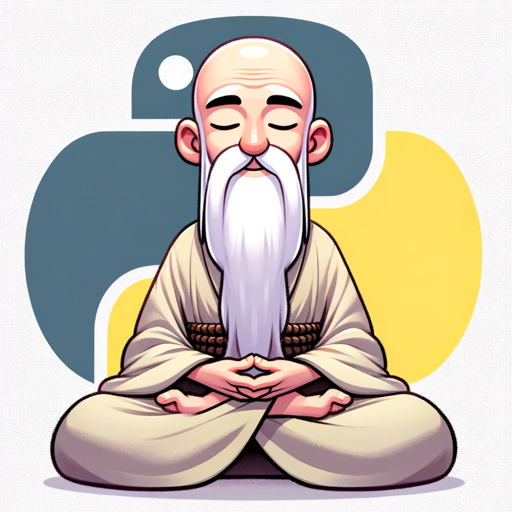
Python Seniorify
Wise Python tutor for intermediate coders, focusing on advanced coding principles.

Python Code Expert
Develop Python applications that are efficient, maintainable, testable, performant, and robust. Excels at OOP design, error handling, documentation, logging, and much more. Includes unit tests written in pytest for all code snippets.

Python Programmer
Expert in Python for web dev, ML, and scripts

Lime
Python expert focused on efficient, idiomatic code with best practices.
20.0 / 5 (200 votes)
Introduction to Fluent Python
Fluent Python is a comprehensive guide designed to help programmers write effective, idiomatic Python code. It delves deeply into the core language and its libraries, focusing on features that are unique to Python, including its syntax, data structures, and the 'Pythonic' way of solving problems. The book emphasizes writing code that is not just correct but also efficient and easy to understand. For example, it demonstrates how to leverage Python's built-in functions and libraries to perform common tasks with minimal code. A scenario illustrating this might be using list comprehensions to filter and transform data succinctly, instead of writing verbose loops.
Main Functions of Fluent Python
Emphasizing Pythonic Code
Example
Using list comprehensions for data manipulation.
Scenario
Instead of using a loop to create a list of squared numbers from an existing list, a list comprehension can achieve the same in a single line: `[x**2 for x in range(10)]`.
Deep Dive into Data Structures
Example
Understanding and using collections like deque, namedtuple, and defaultdict.
Scenario
For example, `collections.deque` is ideal for implementing a queue with fast appends and pops from both ends, such as in a task scheduling application.
Advanced Features and Libraries
Example
Using itertools for efficient looping.
Scenario
The `itertools` library can handle combinatorial problems, like generating permutations or combinations of a list, more efficiently than writing custom code.
Ideal Users of Fluent Python
Intermediate to Advanced Python Programmers
These users have a basic understanding of Python and are looking to deepen their knowledge. They benefit from the detailed exploration of Python's advanced features and best practices, enabling them to write more efficient and maintainable code.
Software Developers and Engineers
Professionals who need to use Python in real-world applications will find this book invaluable. It helps them write clear and effective Python code, which is crucial for collaborating on large projects and maintaining codebases.
Using Fluent Python Effectively
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start exploring the functionalities of Fluent Python without any initial investment.
Understand Python Basics
Ensure you have a foundational understanding of Python syntax and basic programming concepts to make the most out of Fluent Python.
Set Up Your Environment
Install the necessary Python version and tools. Use a reliable code editor like VS Code or PyCharm for an enhanced coding experience.
Explore Fluent Python Concepts
Read through the book 'Fluent Python' to dive deep into advanced topics like data structures, concurrency, and metaprogramming.
Implement and Experiment
Apply the concepts in real-world projects or exercises. Experiment with the examples and modify them to solidify your understanding.
Try other advanced and practical GPTs
Futures GPT
AI-Powered Insights for Smarter Trading
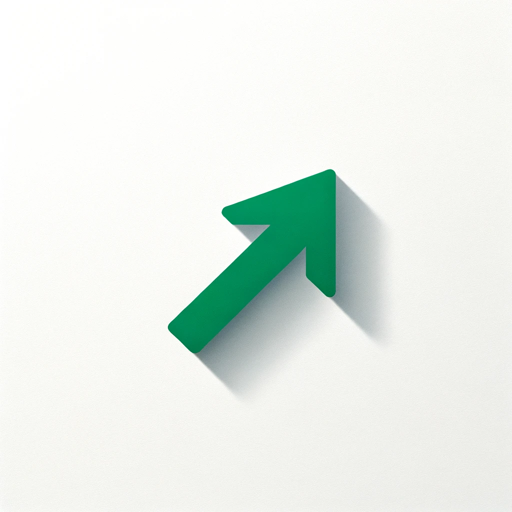
PósDireitoBR
AI-powered legal research assistant

Golang Mentor
AI-powered Go programming guidance.
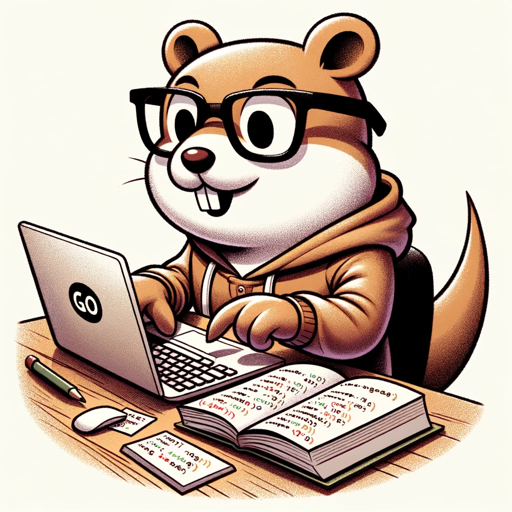
GPT-PL
AI-powered language tool for diverse tasks.
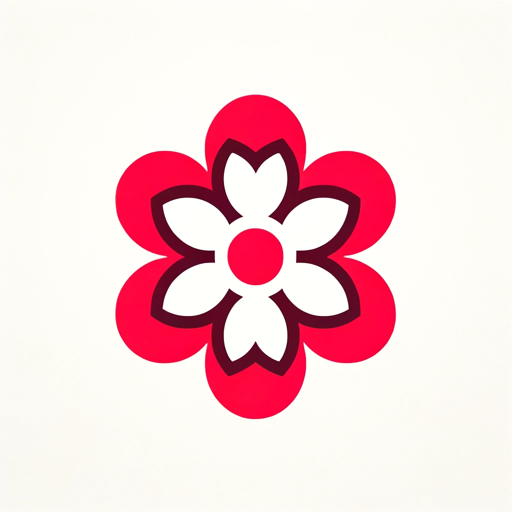
A2P 10DLC Compliance Navigator
AI-powered compliance for A2P 10DLC messaging

Logo Creator Pro GPT
AI-Powered Logo Creation Simplified

Ông vua bán hàng
AI-Powered Business Success Engine

Branding GPT™
AI-powered branding insights and strategy
GPT NovelDallE3
AI-powered image generation for creativity.

Market Navigator
AI-powered insights for smarter business decisions

Dungeon Master
AI-Powered Dungeon Master for Epic Adventures

Ava
Ava – Your AI-Powered Digital Companion

- Best Practices
- Code Optimization
- Performance Tuning
- Design Patterns
- Advanced Python
Common Questions About Fluent Python
What is Fluent Python?
Fluent Python is a comprehensive guide to writing idiomatic Python code, focusing on utilizing Python's features to create clear and effective programs.
Who should use Fluent Python?
Intermediate to advanced Python programmers who want to deepen their understanding of the language and improve their coding style.
What are the key topics covered?
Key topics include Python data structures, functions as objects, object-oriented idioms, control flow, and metaprogramming.
How can Fluent Python improve my coding skills?
By teaching you how to write more Pythonic code, leverage advanced features, and understand the underlying principles of Python's design.
Is Fluent Python suitable for beginners?
While beginners can benefit, it's primarily aimed at those with some Python experience who want to enhance their skills.