Flutter + Bloc + Firebase-guide to build Flutter apps.
AI-powered Flutter development assistant.

How do I implement Firebase auth in Flutter with Bloc?
What's the best way to manage state in Flutter using Bloc?
Can you help me debug this Flutter widget?
How to optimize a Flutter app for performance?
Related Tools
Load More
Flutter Expert
Expert in Flutter and Dart, providing solutions and best practices.
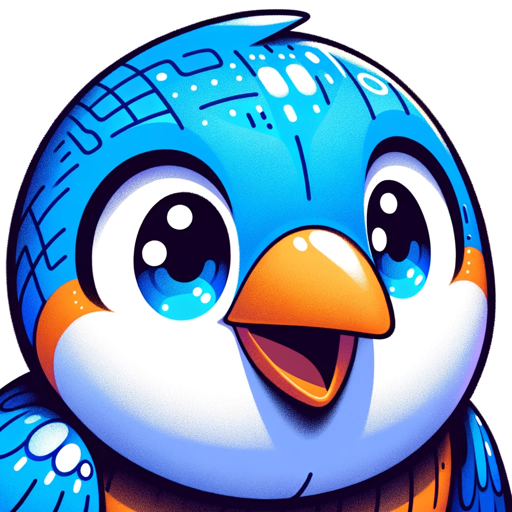
Flutter Pro
Development Co-Pilot.

Flutter Pro
Expert Flutter/Dart guidance with concise, clear advice on best practices. V1.1 05-20-2024

Flutter Expert
Expert in FlutterFlow, Flutter, Firebase, and Dart, offering detailed tech guidance.

Flutter Code Helper
Assists with Flutter coding tasks, providing guidance and code snippets.

Flutter Code Expert
Flutter code formatter and advisor
20.0 / 5 (200 votes)
Understanding Flutter, Bloc, and Firebase
Flutter is an open-source UI toolkit created by Google that enables developers to build natively compiled applications for mobile, web, and desktop from a single codebase. It is known for its fast development cycles, expressive and flexible UI, and native performance. Bloc (Business Logic Component) is a state management library for Flutter that helps manage and separate business logic from the UI. It encourages a clear separation between UI components and business logic, making the code more scalable, maintainable, and testable. Firebase is a comprehensive platform provided by Google that offers a variety of backend services such as authentication, cloud storage, real-time databases, and analytics, which can be integrated seamlessly into Flutter applications. Together, Flutter + Bloc + Firebase offer a powerful combination for developing scalable, responsive, and fully-featured applications. For example, in a real-world scenario like developing a social media application, Flutter would handle the UI/UX, Bloc would manage the state and business logic like user authentication flows, and Firebase would provide backend services such as storing user data, managing authentication, and enabling real-time features like chat.
Core Functions of Flutter + Bloc + Firebase
UI Development with Flutter
Example
Flutter allows developers to create rich, responsive UIs with customizable widgets. It supports both Material Design and Cupertino (iOS) styles.
Scenario
A developer building a cross-platform mobile application for e-commerce uses Flutter to create a seamless shopping experience with consistent design across both iOS and Android platforms. The rich widget catalog enables the creation of complex UI components like product cards, checkout pages, and user profile screens.
State Management with Bloc
Example
Bloc helps manage the state of the application by separating the UI from the business logic. It uses events to trigger changes in the application state and streams to notify the UI of these changes.
Scenario
In a financial tracking app, Bloc manages the state of user accounts, transactions, and budgets. When a user adds a new transaction, an event is triggered, updating the state and reflecting the changes in the UI, such as updating the balance and recent transactions list.
Backend Integration with Firebase
Example
Firebase provides a range of backend services, including real-time databases, cloud storage, and authentication, which are easily integrated with Flutter applications.
Scenario
A messaging app leverages Firebase Authentication for user sign-in, Firestore for storing messages, and Firebase Cloud Messaging for real-time notifications. This setup enables real-time chat functionality, where messages are instantly updated across all user devices.
Target User Groups for Flutter + Bloc + Firebase
Cross-Platform Mobile Developers
Developers who need to build applications for both iOS and Android with a single codebase are ideal users of Flutter. They benefit from Flutter's capability to deliver native-like performance and design consistency across platforms.
Developers Requiring Scalable Backend Solutions
Developers working on applications that require robust, scalable, and real-time backend services find Firebase invaluable. It reduces the need to manage complex backend infrastructure, enabling them to focus on building features and improving the user experience.
Guidelines for Using Flutter + Bloc + Firebase
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start by exploring this platform to understand the basics of AI-driven tools, which can help you grasp the foundational concepts before diving into development.
Set Up Flutter Environment
Install Flutter SDK, set up your preferred IDE (like VS Code or Android Studio), and ensure you have a connected device or emulator ready for testing.
Integrate Firebase with Flutter
Add Firebase to your Flutter project by creating a Firebase project, adding your Android/iOS apps, and configuring it with `google-services.json` or `GoogleService-Info.plist`. Ensure dependencies like `firebase_core`, `firebase_auth`, and `cloud_firestore` are added to your `pubspec.yaml`.
Set Up Bloc State Management
Install the necessary Bloc packages (`flutter_bloc`, `bloc`) and set up your Bloc structure. This involves creating events, states, and Bloc classes to handle business logic efficiently.
Implement Firebase Logic with Bloc
Use your Bloc classes to manage Firebase interactions, such as authentication or database operations. Create Cubit or Bloc instances that listen to Firebase streams and dispatch events to update UI states.
Try other advanced and practical GPTs
Softr Dev Assistant
AI-Powered Custom Code Integration

Stock Photo Friend
AI-powered image descriptions and SEO.

Review My Airbnb Listing
AI-powered insights for better listings.

Master Listing Optimizer
AI-powered Amazon product listing enhancement.

네이버 블로그 글쓰기 도우미 : NAVER 특화
AI-powered assistant for Naver blogging

deep web search
Unleashing AI-Powered Deep Web Insights.

IT Mentor - Ausbildungsbegleiter Fachinformatiker
AI-powered assistant for IT training

Calculus Solver
AI-Powered Calculus Problem Solver

Calculus Solver
AI-Powered Calculus Solutions

Multivariable Calculus Tutor
AI-Powered Assistance for Multivariable Calculus Mastery

Calculus Companion
AI-powered calculus help at your fingertips.

Calculus Professor
AI-powered solutions for calculus mastery.

- Real-time Data
- State Management
- Authentication
- Cloud Functions
- Scalable Apps
Common Q&A on Flutter + Bloc + Firebase
How do I handle Firebase authentication with Bloc in Flutter?
First, create an AuthBloc that manages authentication events (login, logout, sign-up). Then, within the Bloc, use Firebase's `firebase_auth` package to handle authentication logic. Emit corresponding states (Authenticated, Unauthenticated) based on the success or failure of these operations.
Can I use multiple Firebase services in one Bloc?
Yes, a single Bloc can handle multiple Firebase services. For instance, you can create a `UserBloc` that manages both authentication (`firebase_auth`) and user data (`cloud_firestore`). Ensure your Bloc properly manages state transitions for each Firebase interaction.
How does Bloc simplify state management in a Flutter app with Firebase?
Bloc separates business logic from the UI, making the codebase more maintainable and testable. When working with Firebase, Bloc allows you to cleanly handle asynchronous operations like fetching data, managing authentication states, and responding to real-time updates.
What are the benefits of using Firebase with Bloc?
Combining Firebase with Bloc provides a structured approach to managing app states, real-time data updates, and asynchronous operations. This results in a scalable, maintainable, and responsive application that efficiently handles Firebase services.
How can I debug issues when integrating Firebase with Bloc?
Utilize Flutter's DevTools for inspecting states and transitions in your Bloc. Additionally, Firebase provides robust logging tools that can be integrated with your Bloc to diagnose issues related to authentication, database operations, and cloud functions.