Rust-AI-powered Rust assistant
AI-powered Rust guidance

How do I use Rust's ownership model?
Can you explain Rust's error handling?
Tips for optimizing Rust code?
Help with Rust and C interoperability?
Related Tools
Load More
Rust
Your personal Rust assistant and project generator with a focus on responsive, efficient, and scalable code. Write clean code and become a much faster developer.

Rust
Powerful Rust coding assistant. Trained on a vast array of the best up-to-date Rust resources, libraries and frameworks. Start with a quest! 🥷 (V1.9)

Rust Assistant
Your go-to expert in the Rust ecosystem, specializing in precise code interpretation, up-to-date crate version checking, and in-depth source code analysis. I offer accurate, context-aware insights for all your Rust programming questions.

Rust Tutor
An expert in Rust adept at explaining code and teaching you the language.

Professor Rust
Rust expert, translates queries to English, replies in Russian.

Rust Helper
Efficient Rust programming expert.
20.0 / 5 (200 votes)
Introduction to Rust
Rust is a systems programming language focused on safety, speed, and concurrency. It was created by Mozilla Research and has gained popularity due to its unique approach to memory safety without using a garbage collector. Rust's design purpose is to enable the development of reliable and efficient software by providing powerful abstractions and low-level control. A key feature of Rust is its ownership system, which ensures memory safety by enforcing strict rules on how memory is accessed and managed. For instance, in traditional languages like C or C++, developers often face issues such as null pointer dereferencing or buffer overflows. Rust mitigates these issues through its ownership, borrowing, and lifetimes concepts, ensuring that such problems are caught at compile time.
Main Functions of Rust
Ownership and Borrowing
Example
Rust enforces ownership rules through its borrowing mechanism. For example, a variable in Rust can only have one owner at a time, and it can be borrowed immutably multiple times or mutably once.
Scenario
Consider a scenario where you need to manage memory manually in a high-performance application. Using Rust, you can ensure that your application does not have dangling pointers or memory leaks, as the compiler enforces rules that prevent such issues.
Concurrency
Example
Rust provides lightweight, non-blocking concurrency using its async/await syntax. For instance, the 'tokio' library allows you to write asynchronous code that is both efficient and easy to read.
Scenario
In a web server application, handling multiple client requests concurrently without blocking the main thread is crucial. Rust's async/await feature allows you to handle numerous requests efficiently, ensuring high performance and responsiveness.
Pattern Matching
Example
Rust's pattern matching is powerful and expressive. For instance, the 'match' statement can be used to destructure enums and handle various cases effectively.
Scenario
In a compiler, pattern matching can be used to handle different syntax tree nodes. This makes the code more readable and maintainable, as each case of the 'match' statement can directly correspond to different types of nodes.
Ideal Users of Rust
Systems Programmers
Systems programmers who need low-level control over hardware and memory will benefit from Rust. Rust provides the performance of C/C++ while ensuring safety through its strict compile-time checks, making it ideal for developing operating systems, embedded systems, and other performance-critical applications.
Web Developers
Web developers looking for high performance and safety in their web applications can benefit from using Rust. With frameworks like 'Actix' and 'Rocket', Rust allows developers to build fast and secure web applications. Its concurrency model and memory safety features help in building robust server-side applications that can handle a large number of concurrent connections.
How to Use Rust
Visit aichatonline.org
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Install Rust
Install Rust by following the official installation guide at rust-lang.org, which will guide you through downloading Rustup, the Rust toolchain installer.
Set Up Your Environment
Configure your development environment with your favorite IDE or text editor. Common choices include VSCode, IntelliJ IDEA, or CLion, all of which have good Rust support.
Learn Rust Syntax
Start with the Rust Book, an excellent resource for learning Rust from scratch. It covers basic syntax, ownership, borrowing, and more advanced topics.
Build and Run Projects
Create new projects using Cargo, Rust's build system and package manager. Build, run, and test your projects locally to see your progress in action.
Try other advanced and practical GPTs
Ai Swarm Linux
Empower AI with Linux swarms

.GPT NEO
AI-Powered Content & Analysis Tool

Prompt Wizard
AI-powered tool to enhance prompts

Luna
AI-driven emotional support and companionship.

Ask Me Anything
AI-powered answers to any question.

Handwriting to Text GPT
AI-Powered Handwriting to Text Conversion

PhD Reviewer
AI-Powered Research Insight and Analysis
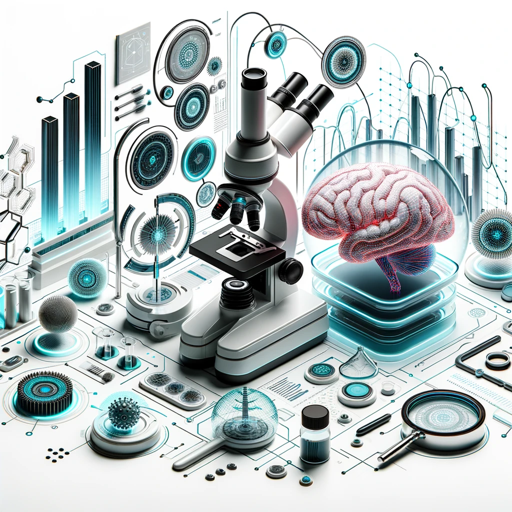
Intrapreneur
AI-powered tool to boost innovation.

API
AI-powered API integration tool
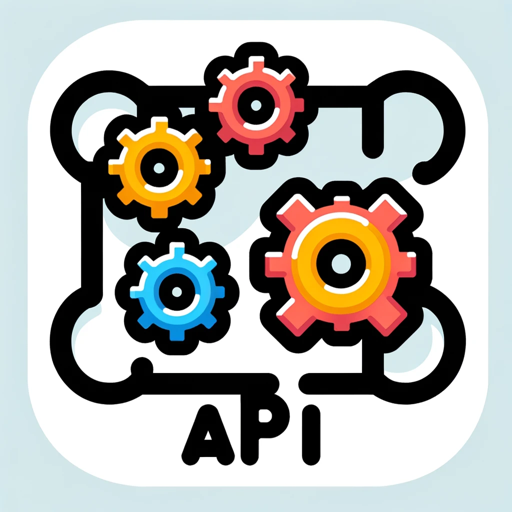
ThorVGPT
AI-powered guide for ThorVG
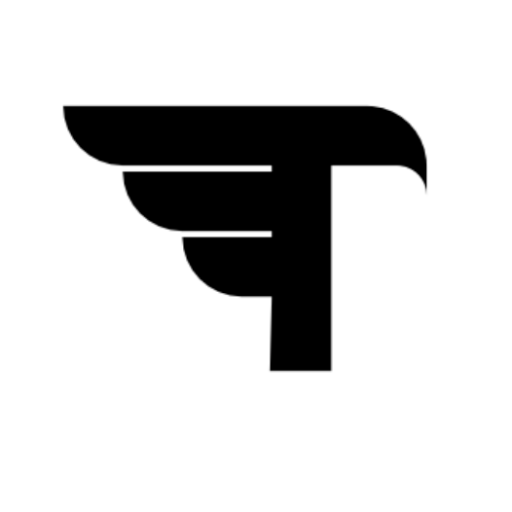
Project Management Hub
AI-powered project management made simple.

PrimeLoupe
AI-powered tool for Amazon product review analysis

- Networking
- Game Development
- Blockchain
- System Programming
- Web Assembly
Detailed Q&A About Rust
What is Rust?
Rust is a systems programming language focused on safety, speed, and concurrency. It eliminates common bugs associated with memory management by using a strict and unique ownership system.
Why choose Rust over other programming languages?
Rust offers memory safety without a garbage collector, concurrency without data races, and a powerful type system. These features make it an excellent choice for systems programming, web assembly, and embedded systems.
How does Rust handle memory management?
Rust uses a unique ownership model with rules that the compiler checks at compile time. This ensures memory safety and prevents data races, null pointer dereferencing, and buffer overflows.
What tools are available for Rust development?
Rust development is supported by various tools including Cargo for package management and building, rustfmt for code formatting, Clippy for linting, and IDE plugins for code editing and debugging.
What are some common use cases for Rust?
Rust is commonly used in system-level programming, web assembly, game development, blockchain, and networking applications due to its performance and safety guarantees.