Rust-Rust learning and development
AI-powered Rust development guide

📡 Help me set up a full REST API in Rust
🧪 Create unit tests for my Rust project
📈 Create a stock market data analysis tool
💡 Teach me a useful skill or trick in Rust
Related Tools
Load More
Rust
Powerful Rust coding assistant. Trained on a vast array of the best up-to-date Rust resources, libraries and frameworks. Start with a quest! 🥷 (V1.9)

Rust Assistant
Your go-to expert in the Rust ecosystem, specializing in precise code interpretation, up-to-date crate version checking, and in-depth source code analysis. I offer accurate, context-aware insights for all your Rust programming questions.

Rust Tutor
An expert in Rust adept at explaining code and teaching you the language.

Rust
A assistant for your Rust code.

Professor Rust
Rust expert, translates queries to English, replies in Russian.

Rust Helper
Efficient Rust programming expert.
20.0 / 5 (200 votes)
Introduction to Rust
Rust is a systems programming language focused on safety, speed, and concurrency. Developed by Mozilla, its design ensures memory safety without a garbage collector, making it suitable for performance-critical applications. Rust’s unique ownership model prevents data races and null pointer dereferences, common issues in other languages like C and C++. The language is designed to be reliable and productive, with a strong emphasis on developer experience and modern tooling. For example, Rust’s compiler provides detailed error messages and suggestions, making it easier to learn and debug. Real-world applications of Rust include web assembly (Wasm) for frontend development, systems programming for operating systems, and backend services that require high performance and low latency.
Main Functions of Rust
Ownership System
Example
In Rust, each value has a single owner, and the ownership can be transferred but not shared. This eliminates issues like dangling pointers and data races.
Scenario
A scenario where the ownership system shines is in systems programming, such as developing an operating system. Memory safety is critical, and Rust’s ownership model ensures that memory is managed safely and efficiently without the need for a garbage collector.
Concurrency
Example
Rust provides threads and async/await for concurrent programming. Its ownership and type system ensure that data races are caught at compile time.
Scenario
Building a high-performance web server can leverage Rust’s concurrency model. By using asynchronous programming, developers can handle many simultaneous connections efficiently, and the compile-time checks ensure thread safety.
Zero-Cost Abstractions
Example
Rust allows developers to write high-level code without incurring runtime overhead. Abstractions are 'zero-cost' as they compile down to the same machine code as hand-written lower-level code.
Scenario
In game development, where performance is crucial, Rust can be used to implement complex game logic with high-level abstractions while maintaining performance comparable to C or C++.
Ideal Users of Rust
Systems Programmers
Developers working on operating systems, embedded systems, or other performance-critical software benefit from Rust’s memory safety and performance. Rust’s low-level control and safety features provide a robust alternative to C and C++.
Web Developers
Rust is ideal for web developers, especially those working with WebAssembly (Wasm). Rust’s performance and safety make it a great choice for writing frontend applications that need to run at near-native speed in the browser.
Detailed Guidelines for Using Rust
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Install Rust using the official installer available at rust-lang.org. This includes the rustup toolchain installer.
Step 3
Set up your development environment by installing an IDE or text editor that supports Rust, such as Visual Studio Code with the Rust Analyzer extension.
Step 4
Familiarize yourself with Cargo, Rust's build system and package manager, by creating a new project with `cargo new project_name` and exploring its structure.
Step 5
Learn Rust syntax and concepts through official documentation, tutorials, and by building small projects to practice and understand best practices and idiomatic Rust code.
Try other advanced and practical GPTs
Just Compare
AI-Powered Product Comparison Made Easy

Explain this Image GPT
AI-powered insights for your images.

Simple Explain
AI-powered simplifications made easy.

Summarise & Explain
AI-Powered Summarization and Explanation
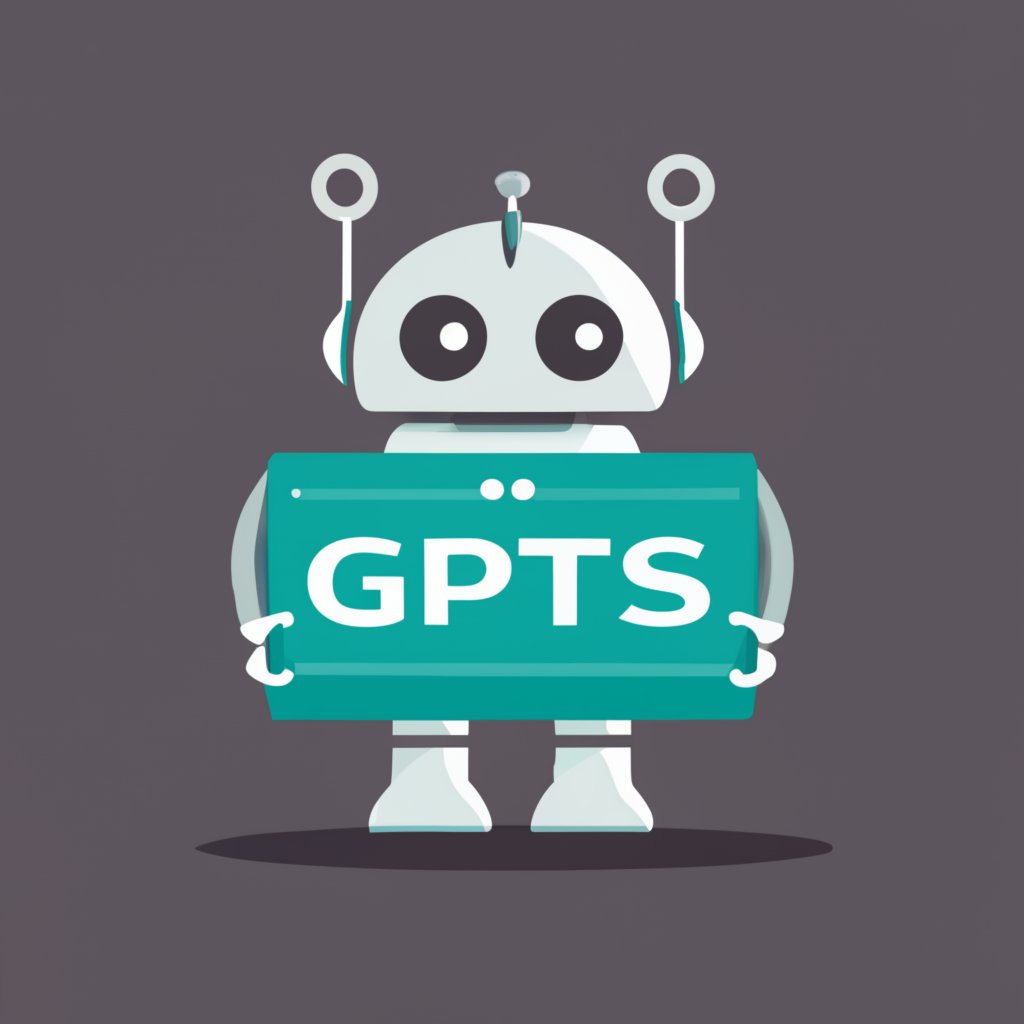
Proposal Pro
AI-powered freelance proposal generator.

Proposal Pro
AI-powered proposal enhancement for professionals

Info Seeker - AI and ML Expert
AI-powered tool for advanced learning and coding
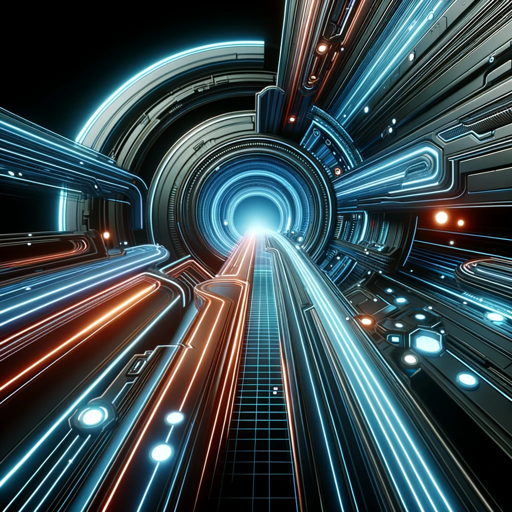
Wisdom Seeker Rooted In Theosophy And Esotericism
AI-powered guidance for spiritual growth

Epigenetic and Genetic Research to Cure Autism
AI-powered genetic insights for autism.

Web Designer
Design stunning web pages with AI power.

Slideshow Designer
AI-powered slideshow creation tool

Designer
AI-powered digital design made easy

- Web Development
- Game Development
- Embedded Systems
- Concurrency
- Systems Programming
Five Detailed Q&A About Rust
What is Rust used for?
Rust is used for systems programming, web development, game development, and embedded systems. Its performance and safety features make it ideal for high-performance applications.
How does Rust ensure memory safety?
Rust ensures memory safety through its ownership model, which enforces strict rules on how memory is accessed and managed. This eliminates common bugs such as null pointer dereferencing and data races.
What are Rust's main advantages over other languages?
Rust offers memory safety without a garbage collector, concurrency without data races, and a robust type system. These features lead to safer and more efficient code compared to languages like C++ and Python.
How can I manage dependencies in Rust?
Dependencies in Rust are managed using Cargo. You can specify dependencies in the `Cargo.toml` file and use `cargo build` to download and compile them automatically.
What tools are available for testing in Rust?
Rust includes built-in support for unit and integration testing. The `#[test]` attribute marks functions as test cases, and `cargo test` runs all tests in the project.