C++-AI-powered C++ guidance
AI-powered C++ assistance for developers

Optimize this C++ code:
Explain this C++ error message:
Best practice for this C++ scenario:
Help me with this advanced C++ concept:
Related Tools
Load More
C++ (Cpp)
Your personal highly sophisticated C++ (Cpp) copilot, with a focus on efficient, scalable and high-quality production code.

C++
Friendly help with C/C++ coding, debugging, and learning in a professional way.

QT 专家
中国的QT C++顶级程序员,用中文回答问题

C++
The first expert in C++. Can utilize Compiler Explorer (godbolt) to compile & run programs, and cppinsights for code transformations.

C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.

C++ Expert
C++ code expert with strict data privacy
20.0 / 5 (200 votes)
Introduction to C++
C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language. It offers a blend of high-level and low-level programming features, making it suitable for system programming, game development, real-time simulations, and performance-critical applications. C++ supports various programming paradigms, including procedural, object-oriented, and generic programming. Its design emphasizes performance, efficiency, and flexibility in resource management. For example, C++ is commonly used in developing operating systems like Windows, game engines such as Unreal Engine, and high-frequency trading systems due to its ability to directly manipulate hardware and memory.
Main Functions of C++
Object-Oriented Programming
Example
C++ allows defining classes, creating objects, and implementing inheritance, polymorphism, encapsulation, and abstraction. For instance, a 'Car' class can have attributes like color, model, and methods like start(), accelerate(). Derived classes like 'ElectricCar' can inherit from 'Car' and extend functionalities.
Scenario
Used in developing complex software systems such as GUI applications, real-time simulations, and game development where modeling real-world entities and their interactions is crucial.
Generic Programming
Example
C++ templates enable writing generic and reusable code. For example, a template function for sorting can work with any data type (int, float, custom objects) without code duplication.
Scenario
Extensively used in the Standard Template Library (STL) which provides generic classes and functions like vectors, lists, and algorithms. This promotes code reuse and type safety in applications like data processing and manipulation.
Low-Level Memory Manipulation
Example
C++ provides direct access to memory through pointers, enabling manual memory management. For instance, dynamic memory allocation using new and delete operators.
Scenario
Critical in system programming, game development, and applications requiring fine-grained control over hardware resources, such as real-time simulations and embedded systems.
Ideal Users of C++
System and Application Programmers
Developers working on operating systems, drivers, and performance-critical applications benefit from C++'s efficiency and control over system resources. Its low-level capabilities are essential for tasks requiring direct hardware manipulation and fine-tuned performance optimization.
Game Developers and Simulation Engineers
C++ is favored in game development and simulations due to its performance and real-time processing capabilities. Game engines like Unreal Engine and graphics libraries such as OpenGL and DirectX heavily rely on C++ for their core functionalities, enabling developers to create high-performance, resource-intensive applications.
Guidelines for Using C++
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Access resources and tools to begin your C++ journey.
Install a C++ compiler and IDE
Download and install a C++ compiler (e.g., GCC, Clang) and an integrated development environment (IDE) like Visual Studio or Code::Blocks.
Write your first program
Start with a simple 'Hello, World!' program to familiarize yourself with the syntax and structure of C++ programs.
Explore C++ libraries and frameworks
Learn about and use the extensive libraries and frameworks available for C++ to extend your program's functionality.
Debug and optimize your code
Utilize debugging tools and techniques to find and fix errors, and optimize your code for better performance.
Try other advanced and practical GPTs
OKR Coach
AI-powered OKR creation and management
Witty Wordsmith
AI-powered writing refinement tool
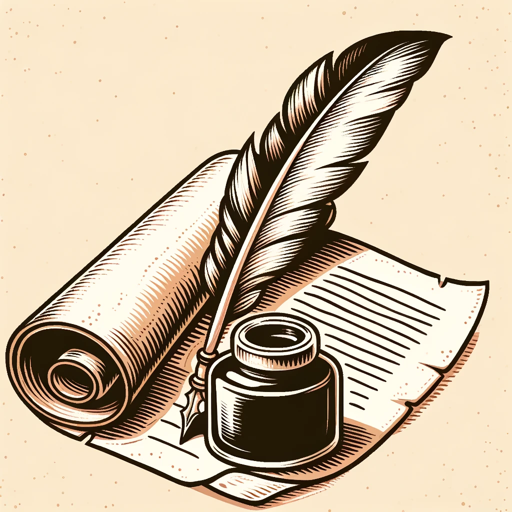
Kemi - Research & Creative Assistant
AI-driven research and visual creativity

AI Tool Finder
Discover the Best AI Tools Effortlessly

Dungeon Adventure Engine RPG
AI-Powered Adventures Await

Tweet Analyzer
Unleash AI to Decode Tweets

GPT TUBE
AI-powered YouTube insights at your fingertips.
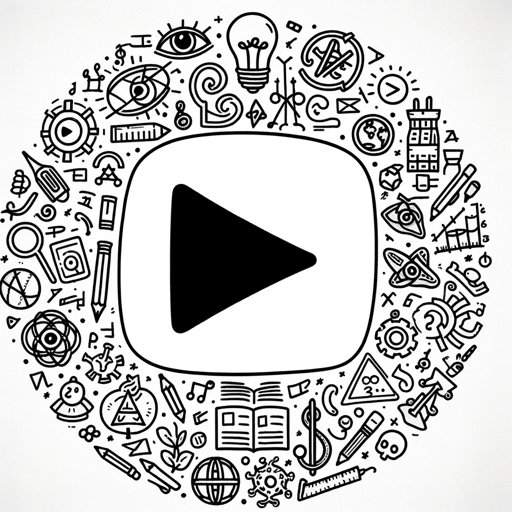
英語→日本語翻訳くん
AI-powered, seamless English to Japanese translation.
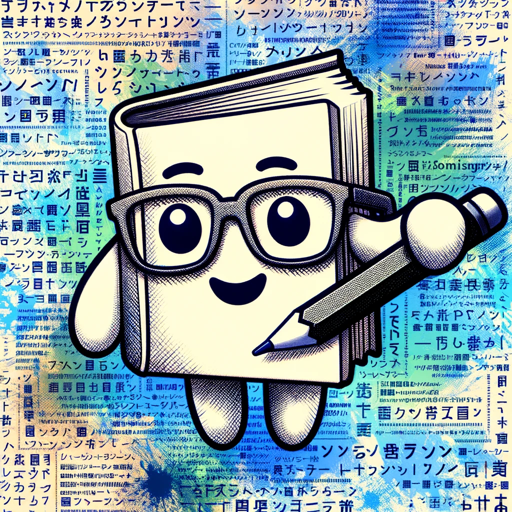
Arabizi
Bridge Arabic and English seamlessly.

Documentation Mentor
AI-powered support for all your documentation needs

한국어/일본어 번역기 | 日本語/韓国語翻訳機
AI-powered Korean-Japanese Translation
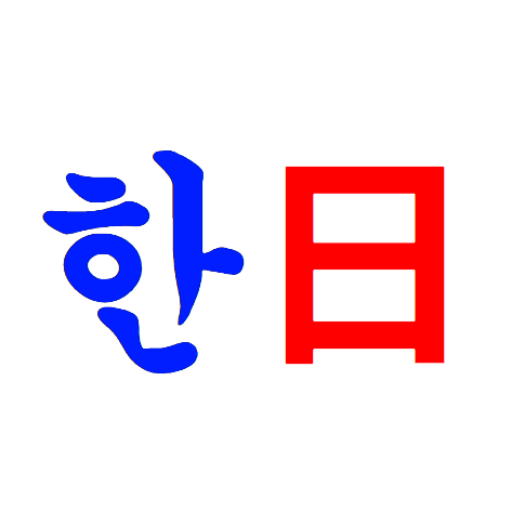
Performance Evaluation Assistant
AI-Powered Evaluations for Growth
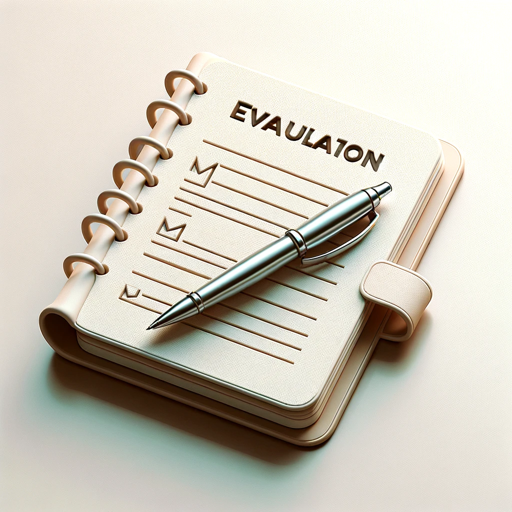
- Game Development
- System Programming
- Scientific Computing
- GUI Development
- Real-time Systems
Common C++ Questions and Answers
What are the key features of C++?
C++ offers object-oriented programming, strong type checking, flexibility with low-level manipulation, and a rich library ecosystem.
How do you manage memory in C++?
Memory management in C++ involves using new and delete operators for dynamic memory allocation and deallocation, along with smart pointers like std::unique_ptr and std::shared_ptr for automatic resource management.
What is the difference between C++98, C++11, and C++14?
C++98 is the original standard, C++11 introduced major features like auto keyword, lambda expressions, and smart pointers, while C++14 refined and extended features introduced in C++11, such as generic lambdas and improved constexpr support.
How do you handle errors in C++?
Error handling in C++ is primarily done using exceptions, which allow you to catch and handle errors gracefully using try, catch, and throw keywords.
What are some best practices for writing efficient C++ code?
Some best practices include using RAII (Resource Acquisition Is Initialization) for resource management, preferring standard library algorithms and containers, minimizing the use of global variables, and profiling your code to find and optimize performance bottlenecks.