C++-AI-powered code assistant
AI-Powered C++ Code Assistant

Help me write code in C++
Optimize my C++ code
Help me fix this C++ error
Explain how pointers work in C++
Related Tools
Load More
C++
Get help from an expert in C++ coding, trained on hundreds of the most difficult C++ challenges. Start with a quest! ⬇🧑💻 (V1.7)

C++ (Cpp)
Your personal highly sophisticated C++ (Cpp) copilot, with a focus on efficient, scalable and high-quality production code.

QT 专家
中国的QT C++顶级程序员,用中文回答问题

C++
The first expert in C++. Can utilize Compiler Explorer (godbolt) to compile & run programs, and cppinsights for code transformations.

C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.

C++ Expert
C++ code expert with strict data privacy
20.0 / 5 (200 votes)
Introduction to C++
C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language, or 'C with Classes'. It is designed to provide both high-level abstractions for easy programming and low-level memory manipulation features for performance-critical applications. C++ supports object-oriented, procedural, and generic programming paradigms, making it a versatile language for a wide range of software development needs. Key features include strong type checking, resource management, and direct access to hardware via low-level constructs. Example: In game development, C++ allows developers to write high-performance code for real-time simulations and graphics rendering, ensuring smooth gameplay experiences.
Main Functions of C++
Object-Oriented Programming (OOP)
Example
Defining classes and objects to model real-world entities.
Scenario
In a banking system, classes such as `Account`, `Customer`, and `Transaction` can encapsulate data and operations, providing a clear structure and reusability.
Standard Template Library (STL)
Example
Using STL containers like vectors, maps, and sets for efficient data management.
Scenario
In a text editor, a `std::vector` can dynamically manage a list of strings representing lines of text, providing efficient insertion, deletion, and access operations.
Low-Level Memory Manipulation
Example
Using pointers and manual memory allocation with `new` and `delete`.
Scenario
In a memory-intensive application such as a database engine, direct memory management allows for fine-tuned control over performance and resource usage.
Ideal Users of C++
System Programmers
Developers who need to write low-level code for operating systems, drivers, and embedded systems. They benefit from C++'s ability to manipulate hardware directly and manage resources efficiently.
Game Developers
Professionals creating high-performance games and real-time simulations. C++ provides the necessary speed and control over system resources to ensure optimal game performance and responsiveness.
How to Use C++
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Install a C++ compiler like GCC or Clang, or use an IDE such as Visual Studio or Code::Blocks that includes a compiler.
Step 3
Write your C++ code in a text editor or IDE. Start with basic programs to understand syntax and structure.
Step 4
Compile your code using the installed compiler to check for errors and generate an executable file.
Step 5
Run the executable file to see the output of your program. Debug and refine your code as necessary.
Try other advanced and practical GPTs
샘호트만's 초보자들을 위한 데이터 분석 서포터
AI-powered data analysis for beginners.

TALE-C
AI-Powered Dynamic Storytelling
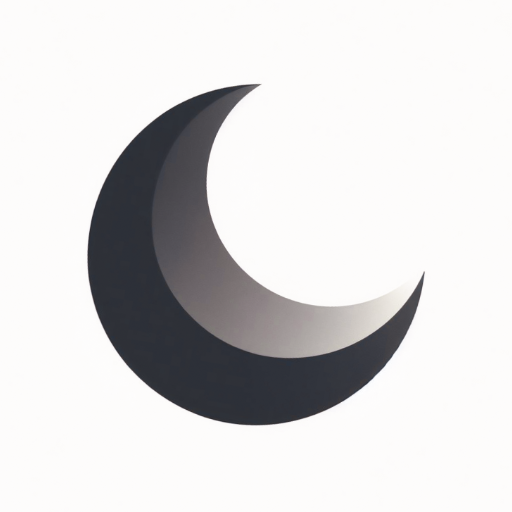
AnkiGPT
AI-powered flashcard creation for efficient learning.

ArcPy Expert
AI-Powered GIS Solutions at Your Fingertips

IBgrad
AI-powered support for IB success

ChatYouTube
AI-Powered YouTube Content Analyzer

Resumo de livros
AI-powered book summaries for everyone.

Convert to Word with OCR
AI-powered OCR for seamless document conversion

KOR GPT
AI-powered Korean language assistant

마케팅왕 - SEO 특화 글쓰기 도움 (마케팅/마케터)
AI-powered SEO content optimization

Expert Crypto Trade
AI-powered crypto trading insights

The Assignment Solver
AI-Powered Solutions for Every Assignment

- Game Development
- Performance Optimization
- Software Development
- System Programming
- Real-time Simulations
C++ Q&A
What is C++ primarily used for?
C++ is used for system/software development, game development, real-time simulations, and performance-critical applications.
How does C++ differ from C?
C++ is an extension of C with object-oriented features, offering classes, inheritance, polymorphism, and more robust type checking.
What are some key features of C++?
Key features include object-oriented programming, templates, the Standard Template Library (STL), and multi-paradigm programming capabilities.
Why is C++ preferred for game development?
C++ provides high performance, direct memory access, and fine-grained control over system resources, making it ideal for the performance-intensive needs of game development.
Can I use C++ for web development?
While not common, C++ can be used for backend development with frameworks like CppCMS or integrated into web applications for performance-critical tasks.