C++-trial for C++ guidance
AI-powered C++ Copilot

Hello fellow developer, what can I do for you?
Related Tools
Load More
C++
Get help from an expert in C++ coding, trained on hundreds of the most difficult C++ challenges. Start with a quest! ⬇🧑💻 (V1.7)

C++ (Cpp)
Your personal highly sophisticated C++ (Cpp) copilot, with a focus on efficient, scalable and high-quality production code.

C++
Friendly help with C/C++ coding, debugging, and learning in a professional way.

QT 专家
中国的QT C++顶级程序员,用中文回答问题

C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.

C++ Expert
C++ code expert with strict data privacy
20.0 / 5 (200 votes)
Introduction to C++
C++ is a high-performance, general-purpose programming language developed by Bjarne Stroustrup in 1979 as an extension of the C language. It incorporates object-oriented, generic, and functional programming features while providing facilities for low-level memory manipulation. The primary design purpose of C++ is to enable the development of software that is both efficient and maintainable, making it suitable for systems programming, game development, real-time simulations, and applications requiring high performance. C++ provides a fine balance between high-level abstractions and low-level system access, allowing developers to write both high-level code and hardware-level code in the same program.
Main Functions of C++
Object-Oriented Programming (OOP)
Example
class Animal { public: virtual void sound() = 0; }; class Dog : public Animal { public: void sound() override { cout << "Bark"; } };
Scenario
OOP in C++ is used extensively in scenarios requiring modularity and code reuse. For example, in game development, different game entities like characters, enemies, and items can be represented as classes, allowing for easy extension and maintenance.
Generic Programming
Example
template<typename T> T add(T a, T b) { return a + b; } int main() { cout << add(3, 4); cout << add(3.5, 4.5); }
Scenario
Generic programming in C++ is utilized to write flexible and reusable code. For instance, template functions and classes can handle different data types, such as in a library where sorting algorithms need to work with various data types like integers, floats, or custom objects.
Standard Template Library (STL)
Example
#include <vector> #include <algorithm> std::vector<int> vec = {1, 2, 3, 4}; std::sort(vec.begin(), vec.end());
Scenario
STL provides a collection of template classes and functions for common data structures and algorithms. In financial software development, STL components like vectors, maps, and algorithms can be used to manage and process large datasets efficiently.
Ideal Users of C++
Systems Programmers
Systems programmers benefit from C++'s ability to interact closely with hardware and manage system resources efficiently. They use C++ to develop operating systems, drivers, and embedded systems where performance and resource control are critical.
Game Developers
Game developers prefer C++ due to its performance and ability to handle complex simulations and real-time graphics. C++ is used in game engines like Unreal Engine to create high-performance games that require fast computation and rendering.
Steps to Use C++
1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
2
Install a C++ compiler like GCC, Clang, or MSVC, and an IDE such as Visual Studio, Code::Blocks, or CLion.
3
Familiarize yourself with C++ syntax and concepts by studying resources such as books, online tutorials, and official documentation (https://eel.is/c++draft/).
4
Start writing C++ code by creating basic programs, then gradually move to more complex projects involving data structures, algorithms, and design patterns.
5
Utilize tools and libraries like Boost, C++ STL, and PVS-Studio for code analysis and optimization to ensure code quality and performance.
Try other advanced and practical GPTs
C++
AI-driven C++ programming assistant.

C
AI-powered C programming made simple
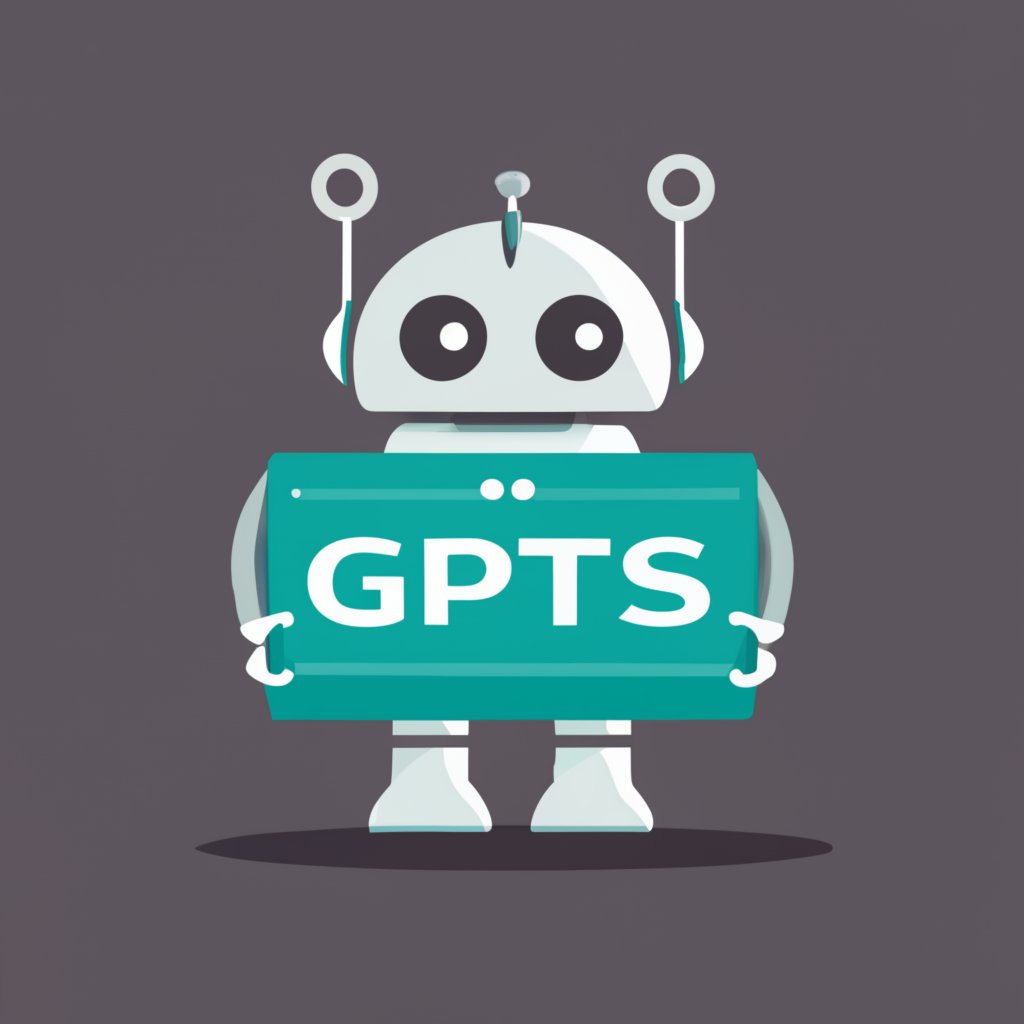
C++
AI-driven C++ coding solutions

C# Expert
AI-powered C# development assistance.

Qlik SaaS
AI-powered insights for smarter decisions.

Create Similar Image / Picture with AI
AI-powered Image Duplication Made Easy

C HELPER
AI-powered C programming assistant.
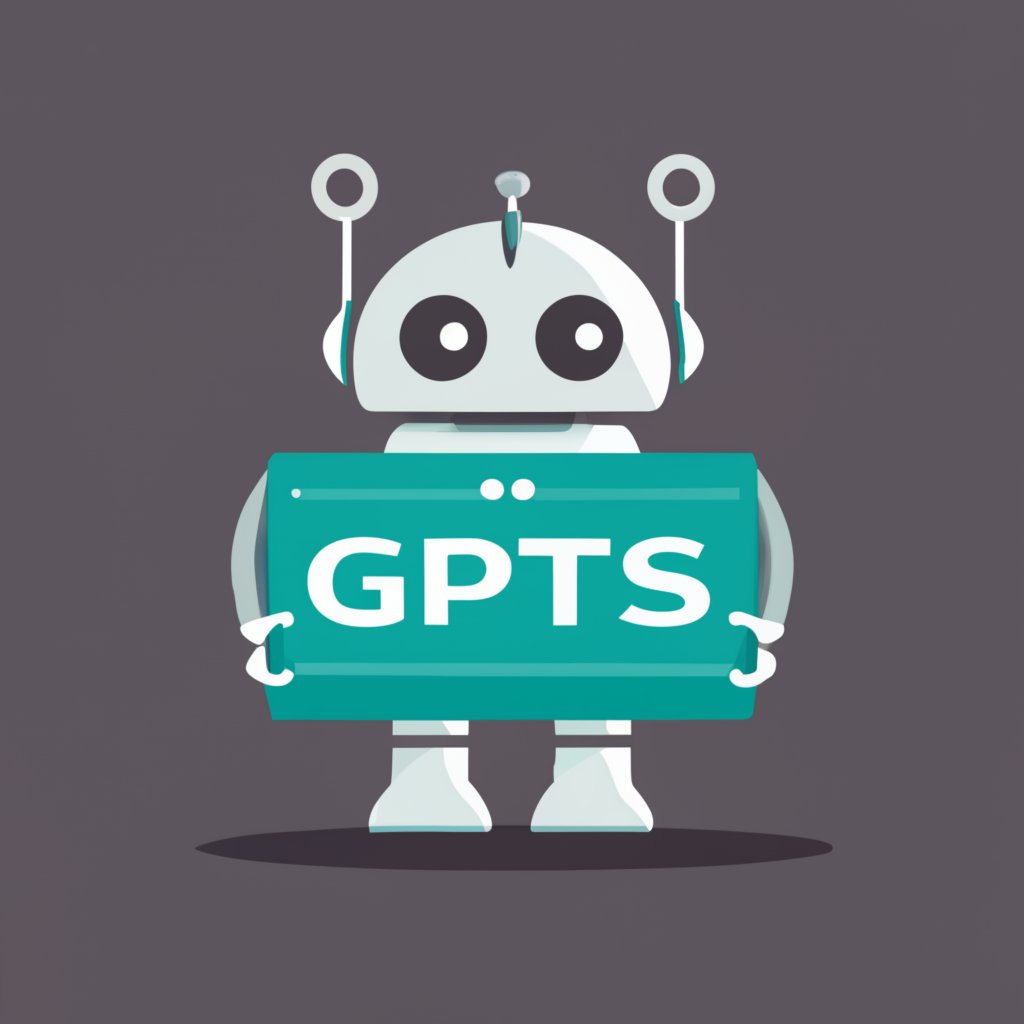
C# Sage
AI-Powered C# Coding Assistant

Leet Code(C++ Version)💻
AI-powered C++ problem solver.

Peanutize Me
Transform your photos into Peanuts characters with AI.

Simpsonize Me
Transform Your Text with AI Humor
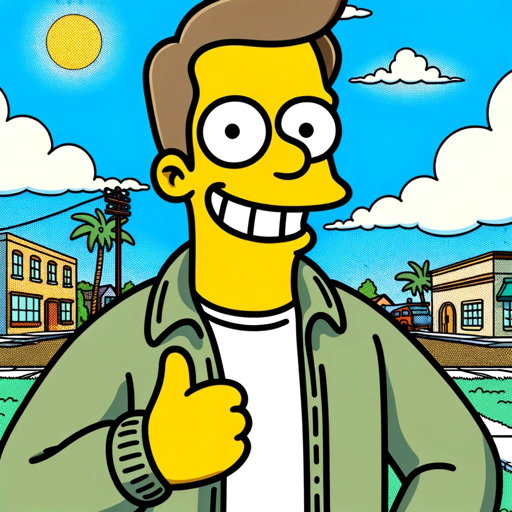
Image to Cartoon
AI-powered photo to cartoon tool.

- Game Development
- High-Performance
- System Software
- Financial Systems
- Real-time Simulation
C++ Q&A
What is C++?
C++ is a powerful general-purpose programming language developed by Bjarne Stroustrup. It supports procedural, object-oriented, and generic programming paradigms, making it suitable for a wide range of applications from system software to game development.
How can I handle memory management in C++?
In C++, memory management can be handled manually using operators like `new` and `delete`, or automatically using smart pointers provided by the Standard Library (e.g., `std::unique_ptr`, `std::shared_ptr`). Smart pointers help prevent memory leaks and dangling pointers.
What are some common uses of C++?
C++ is commonly used in system/software development, game development, real-time simulation, financial systems, and high-performance applications. Its efficiency and control over hardware resources make it ideal for these areas.
What are the key features introduced in C++20?
C++20 introduced several significant features, including concepts, ranges, coroutines, modules, the spaceship operator (`<=>`), and enhanced constexpr capabilities. These features improve code readability, efficiency, and metaprogramming capabilities.
How can I ensure my C++ code is secure and high-quality?
To ensure code quality and security in C++, follow best practices such as adhering to the C++ Core Guidelines (https://isocpp.github.io/CppCoreGuidelines/CppCoreGuidelines), performing code reviews, using static analysis tools like PVS-Studio, and writing comprehensive unit tests.