C++-AI-powered coding assistant
AI-driven C++ coding solutions

Describe a competitive programming problem in Markdown format.
I have a CSP problem; I need a C++ solution.
Write a C++ program for this NOI problem description.
Please solve this competitive coding challenge in C++.
Related Tools
Load More
C++
Get help from an expert in C++ coding, trained on hundreds of the most difficult C++ challenges. Start with a quest! ⬇🧑💻 (V1.7)

C++ (Cpp)
Your personal highly sophisticated C++ (Cpp) copilot, with a focus on efficient, scalable and high-quality production code.

C++
Friendly help with C/C++ coding, debugging, and learning in a professional way.

QT 专家
中国的QT C++顶级程序员,用中文回答问题

C++
The first expert in C++. Can utilize Compiler Explorer (godbolt) to compile & run programs, and cppinsights for code transformations.

C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.
20.0 / 5 (200 votes)
Introduction to C++
C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language, or 'C with Classes'. It has imperative, object-oriented, and generic programming features, while also providing facilities for low-level memory manipulation. C++ is designed for systems programming, resource-constrained software, and large systems, with performance, efficiency, and flexibility of use as its design highlights. Its use spans from application software, device drivers, embedded software, high-performance server and client applications, and entertainment software such as video games.
Main Functions of C++
Object-Oriented Programming (OOP)
Example
class Car { public: void start() { cout << 'Car started'; } };
Scenario
This feature allows developers to model real-world entities in their applications. For example, in a vehicle management system, different types of vehicles (cars, trucks, bikes) can be represented as classes, allowing for better code organization and reuse.
Standard Template Library (STL)
Example
#include <vector> #include <algorithm> std::vector<int> numbers = {1, 2, 3, 4, 5}; std::sort(numbers.begin(), numbers.end());
Scenario
STL provides a set of common classes and functions for data structures and algorithms. It includes containers, iterators, and algorithms which make it easier to implement complex data structures like linked lists, stacks, queues, and algorithms like sorting and searching. For example, STL can be used in financial software to manage and process collections of transactions.
Memory Management
Example
int* ptr = new int(10); delete ptr;
Scenario
C++ provides precise control over system resources through dynamic memory allocation and deallocation, which is crucial in developing resource-constrained applications like operating systems, embedded systems, or real-time systems where efficient use of memory and performance are critical.
Ideal Users of C++
Systems Programmers
C++ is highly suitable for systems programmers who develop operating systems, drivers, and other low-level software. Its efficiency and performance, along with low-level memory manipulation capabilities, make it ideal for writing fast and resource-efficient code.
Game Developers
Game developers benefit from C++ due to its high performance and the extensive libraries available for graphics, physics, and real-time processing. Many game engines, such as Unreal Engine, are built using C++ because it allows for high optimization and control over system resources, essential for rendering complex graphics and ensuring smooth gameplay.
How to Use C++
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Ensure you have a C++ development environment set up. This includes installing a compiler like GCC and an IDE like Visual Studio Code or CLion.
Step 3
Write your C++ code in the IDE, utilizing standard libraries and syntax. For example, start with a simple 'Hello World' program to test your setup.
Step 4
Compile your code using the compiler. In most cases, this can be done directly from the IDE or using command line tools.
Step 5
Run the compiled program to see the output. Debug and refine your code as necessary, making use of debugging tools available in your IDE.
Try other advanced and practical GPTs
C# Expert
AI-powered C# development assistance.

Qlik SaaS
AI-powered insights for smarter decisions.

Create Similar Image / Picture with AI
AI-powered Image Duplication Made Easy

Event Finder
AI-powered global music event discovery.

Prompt Crafter
AI-powered precision for crafting perfect prompts

Character Crafter
AI-powered character creation made simple.

C
AI-powered C programming made simple
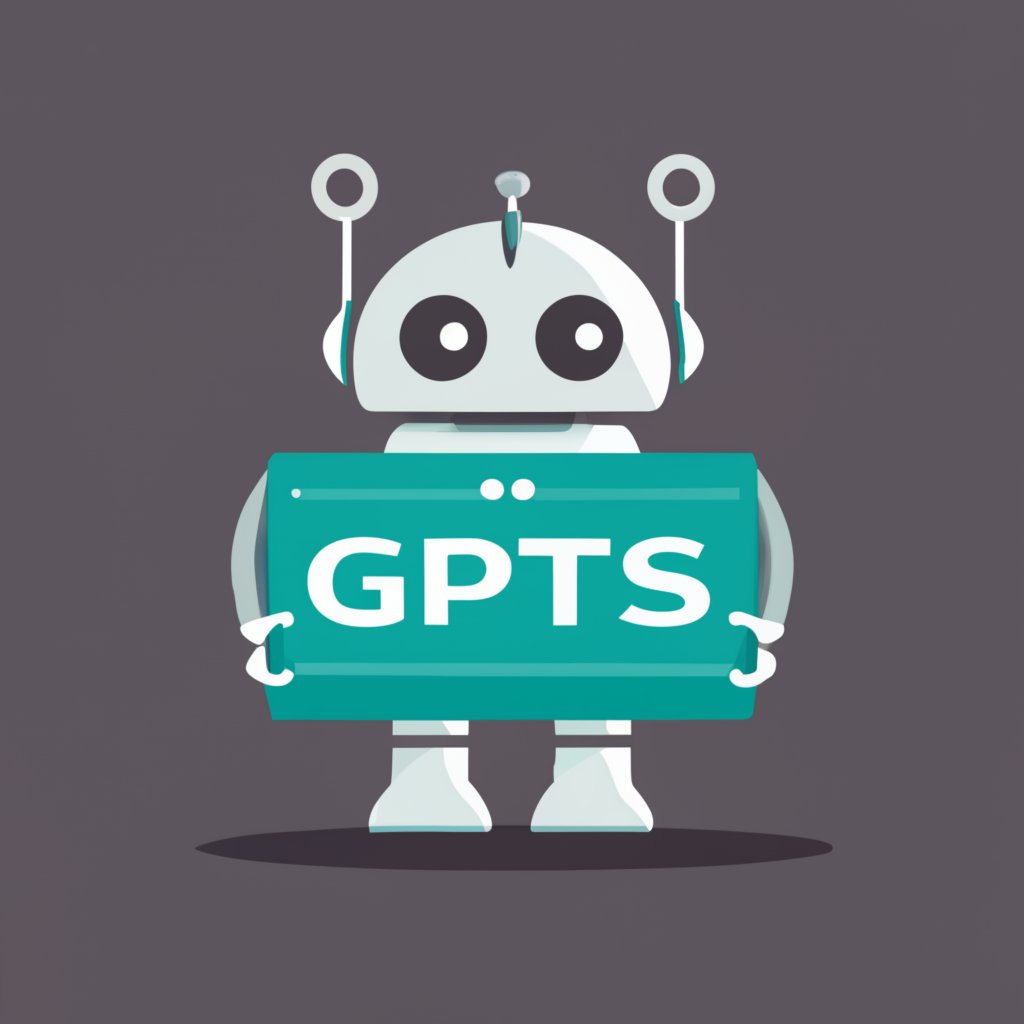
C++
AI-driven C++ programming assistant.

C++
AI-powered C++ Copilot

C HELPER
AI-powered C programming assistant.
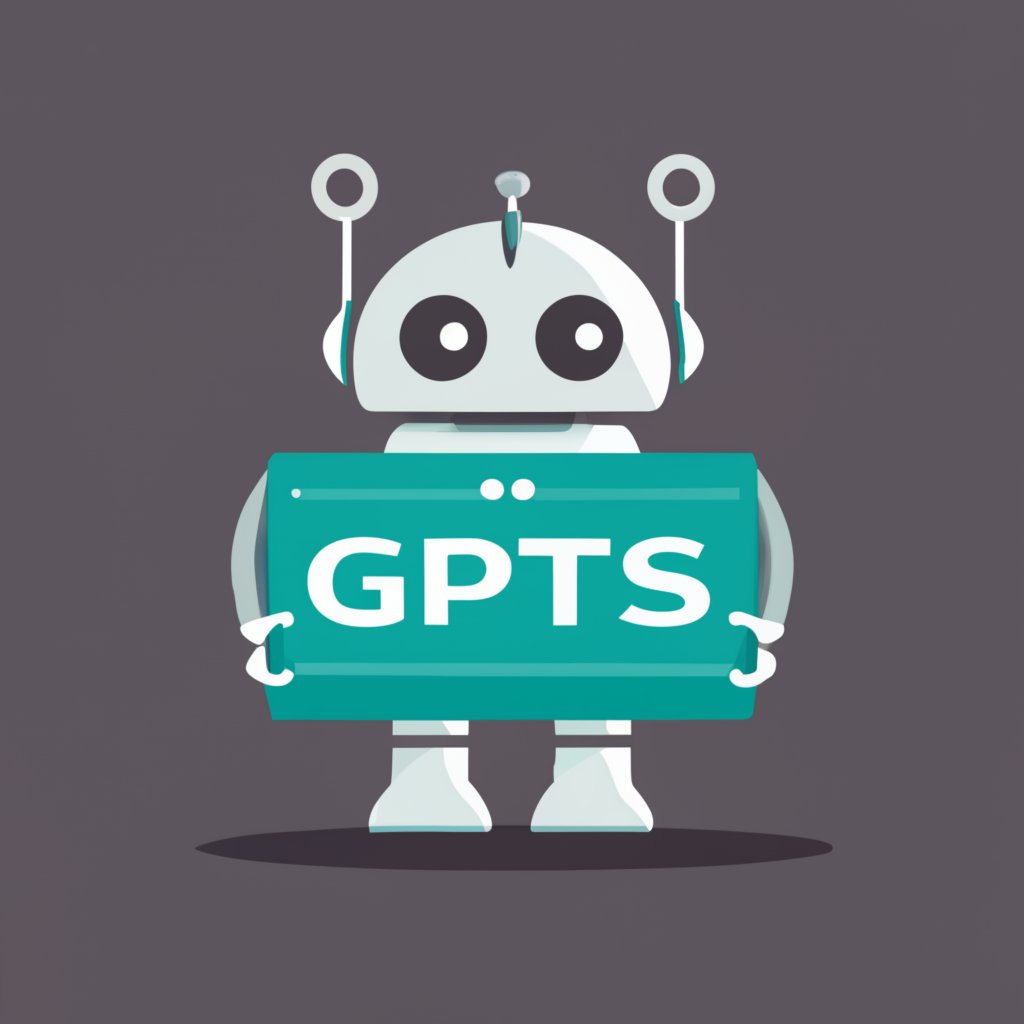
C# Sage
AI-Powered C# Coding Assistant

Leet Code(C++ Version)💻
AI-powered C++ problem solver.

- Game Development
- Software Engineering
- Embedded Systems
- System Programming
- Competitive Programming
C++ Q&A
What is C++?
C++ is a high-level programming language that supports procedural, object-oriented, and generic programming features. It is widely used for system/software development and game programming.
How do I compile a C++ program?
To compile a C++ program, you need a C++ compiler like GCC. In the terminal, navigate to the directory containing your C++ file and use a command like 'g++ -o outputfile sourcefile.cpp'. This will generate an executable file.
What are the key features of C++?
C++ features include classes and objects, inheritance, polymorphism, templates, and the Standard Template Library (STL). These features support various programming paradigms and efficient code execution.
Can I use C++ for web development?
While C++ is not commonly used for front-end web development, it can be used for back-end development and for creating web servers, especially in performance-critical applications.
How does C++ handle memory management?
C++ provides both manual and automatic memory management. Developers can allocate and deallocate memory using 'new' and 'delete' keywords, and the Standard Library offers smart pointers for automatic memory management.