jQuery-jQuery guide and tools
Enhance web projects with jQuery's power

What are the latest features in jQuery?
How can I optimize my web development using jQuery?
Can you explain jQuery's AJAX methods?
What are some best practices for using jQuery in web design?
Related Tools
Load More
Javascript
Your personal Javascript assistant and project generator with a focus on responsive, beautiful, and scalable code. Write clean code and become a much faster developer.

shadcn/ui
Senior UI/UX Engineer expert in shadcn/ui, Next JS & React JS

Daisy UI
UI/UX specialist in DaisyUI with frontend and backend insights

Javascript God
Embodies pinnacle of Javascript expertise.

JavaScript Developer
This GPT model is tailored to teach and assist with JavaScript programming.

JavaScript expert
Transform your JavaScript code with expert refactoring tips and tricks!
20.0 / 5 (200 votes)
Introduction to jQuery
jQuery is a fast, small, and feature-rich JavaScript library designed to simplify the client-side scripting of HTML. It was created by John Resig in 2006 with the aim to write less code while accomplishing more. jQuery makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. The core idea behind jQuery is to make it easier for developers to navigate a document, select DOM elements, create animations, handle events, and develop Ajax applications. For instance, jQuery allows you to select elements with CSS-style selectors and perform actions on them with ease. Consider a scenario where you want to hide all paragraphs on a page; with jQuery, you can do this with a single line of code: $('p').hide();.
Main Functions of jQuery
DOM Manipulation
Example
$('#content').html('Hello, world!');
Scenario
Use this to dynamically change the content of a webpage. For instance, updating a user profile section with new information fetched from a server.
Event Handling
Example
$('button').on('click', function() { alert('Button clicked!'); });
Scenario
Use this to bind events to elements, such as showing an alert when a button is clicked, improving interactivity on web pages.
Ajax Requests
Example
$.ajax({ url: 'data.json', method: 'GET', success: function(data) { console.log(data); } });
Scenario
Use this to load data from the server without refreshing the page. For example, fetching user data to populate a profile section dynamically.
Ideal Users of jQuery
Web Developers
Web developers, ranging from beginners to advanced, can benefit greatly from jQuery. It simplifies many common tasks in JavaScript, allowing developers to write less code while achieving more functionality. Its ease of use and extensive documentation make it a valuable tool for speeding up development processes.
Front-end Designers
Front-end designers who may not be deeply versed in JavaScript but need to add interactivity to their designs can use jQuery. It provides a straightforward way to handle animations, DOM manipulation, and event handling, thus enhancing the user interface without requiring deep programming knowledge.
How to Use jQuery
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
This platform provides an easy way to get started with jQuery and other web development tools.
Include the jQuery library in your HTML.
Use a Content Delivery Network (CDN) to include the latest version of jQuery in your HTML file: `<script src='https://code.jquery.com/jquery-3.6.0.min.js'></script>`.
Understand jQuery syntax and selectors.
jQuery uses CSS-like selectors to select elements and perform actions on them. Example: `$('p').hide();` hides all `<p>` elements.
Practice common jQuery tasks.
Get familiar with tasks like event handling (`$('button').click(function() { alert('Button clicked!'); });`), DOM manipulation, and AJAX requests.
Explore jQuery plugins and UI.
jQuery has a vast ecosystem of plugins and UI components. Explore and integrate them into your projects to extend functionality.
Try other advanced and practical GPTs
POSTGRES GPT
AI-Powered PostgreSQL Assistance

Word Tuner (for Book Writers) 📚
AI-powered tool for age-appropriate writing.

NVivo Data Analysis
AI-powered insights for qualitative data.

Code & Data Sage
AI-driven solutions for coding and data challenges.

HD Image Converter
AI-powered image enhancement made easy

AI girlfriend
AI-powered virtual companion for personalized interactions.
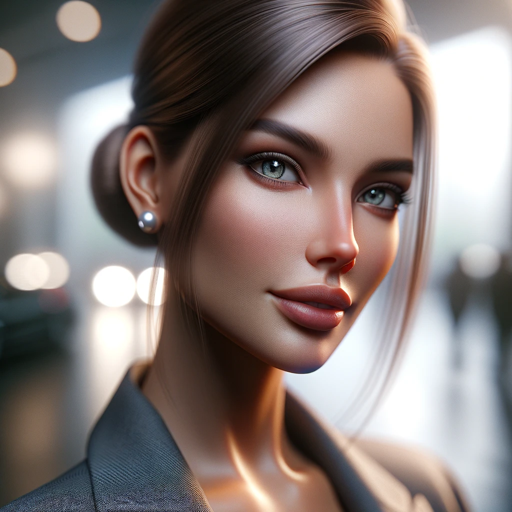
AA 05
AI-driven insights at your fingertips.

InfoProductor GPT
AI-powered tool for engaging Instagram stories.

Crayon Delight 🎨️
AI-powered charming crayon creations

神经导航
AI-powered insights for neuroimaging professionals

Native Speaker Polisher
AI-driven language enhancement for professionals.

Weibo小助手 v1.5
AI-powered Weibo content creation made easy.

- Web Development
- Event Handling
- Plugins
- DOM Manipulation
- AJAX Requests
jQuery Q&A
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, and animation.
How can I include jQuery in my project?
You can include jQuery by linking to a CDN in your HTML file: `<script src='https://code.jquery.com/jquery-3.6.0.min.js'></script>`.
What are jQuery selectors?
jQuery selectors are used to select and manipulate HTML elements. They use CSS-like syntax. Example: `$('#id')`, `.class`, `$('tag')`.
How do I make an AJAX request with jQuery?
Use jQuery's `$.ajax` method. Example: `$.ajax({url: 'example.com/api', method: 'GET', success: function(data) { console.log(data); }});`.
Can I use jQuery with other JavaScript frameworks?
Yes, jQuery can be used alongside other frameworks like Angular, React, and Vue. Ensure there are no conflicts by using jQuery in noConflict mode if needed.