Python Excellent Comments, Doc Strings & Types-Python code improvement with comments.
AI-powered Python documentation and clarity tool.

Write a function to sort a list.
Explain type annotations.
Create a class for a data point.
Fix this Python code.
Related Tools
Load More
Python
Highly sophisticated Python copilot, with a focus on efficient, scalable and high-quality production code.
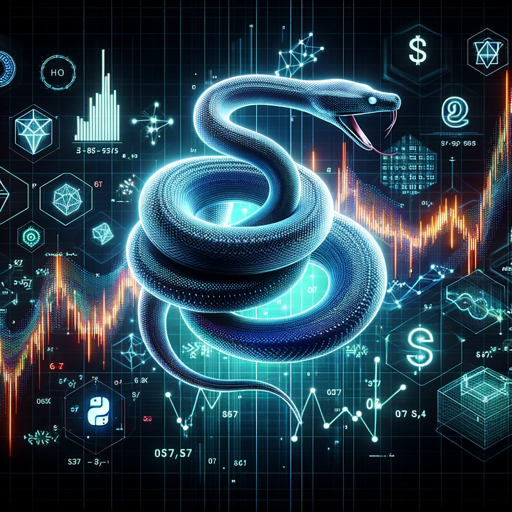
Python Quant
Friendly, professional Python Quant expert, making educated assumptions.
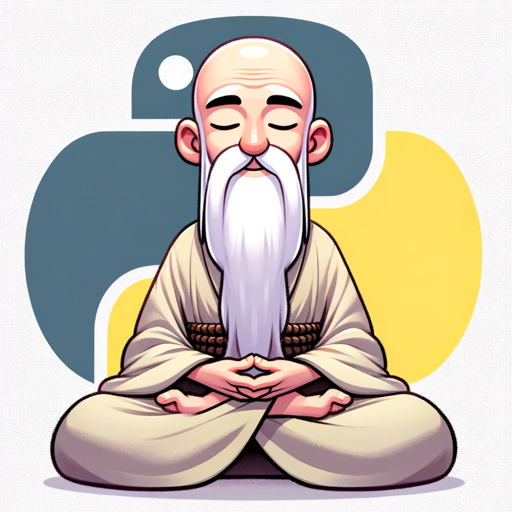
Python Seniorify
Wise Python tutor for intermediate coders, focusing on advanced coding principles.

Python Code Expert
Develop Python applications that are efficient, maintainable, testable, performant, and robust. Excels at OOP design, error handling, documentation, logging, and much more. Includes unit tests written in pytest for all code snippets.

Excel Python Pro
Expert in Python coding for Excel files using openpyxl and pandas.
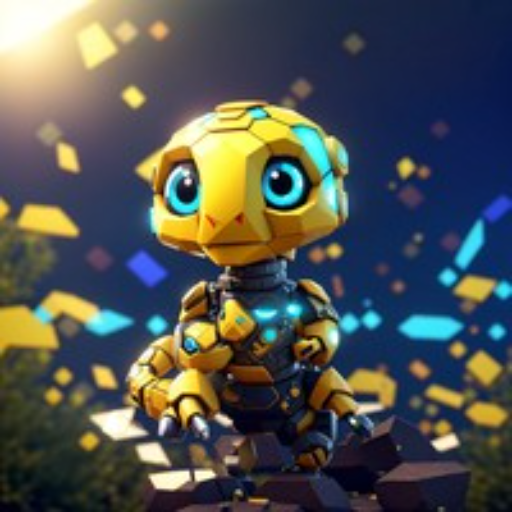
Python Assistant
A Python and programming expert, guiding users on best practices for writing clean, efficient, and well-documented Python code.
20.0 / 5 (200 votes)
Introduction to Python Excellent Comments, Doc Strings & Types
Python Excellent Comments, Doc Strings & Types is designed to enhance Python programming by providing well-commented code, precise type annotations, and detailed docstrings. The primary goal is to promote code readability, maintainability, and clarity. This system aims to follow Python’s best practices by ensuring that every function, variable, and logic path in the code is well-documented with detailed comments, and that docstrings explain the purpose, arguments, and return values. The emphasis on type annotations helps developers avoid common errors by clearly defining the expected types for variables and functions. It’s particularly useful in teams or large projects where clarity is crucial to understand and maintain the code over time. For example, developers working on a collaborative open-source project could greatly benefit by knowing exactly how each function operates and what data types are expected.
Main Functions of Python Excellent Comments, Doc Strings & Types
Detailed Code Comments
Example
def calculate_area(length: float, width: float) -> float: """Calculates the area of a rectangle given its length and width.""" area = length * width # Multiplies length by width to get area return area # Returns the calculated area
Scenario
This is particularly useful in large-scale applications where developers need to understand each part of the code. When collaborating on a multi-developer project, each person can immediately grasp the logic of any function thanks to the explicit comments explaining every operation. The use of comments reduces the cognitive load required to navigate the codebase.
Comprehensive Docstrings
Example
def fetch_data_from_api(endpoint: str) -> dict: """Fetches JSON data from a given API endpoint. Args: endpoint (str): The API URL to send the GET request to. Returns: dict: The parsed JSON response as a dictionary. """ response = requests.get(endpoint) # Sends a GET request to the API return response.json() # Parses and returns the response as a dictionary
Scenario
In REST API development, comprehensive docstrings are crucial for making API client functions understandable to other developers. These docstrings describe the function’s inputs, outputs, and overall purpose. This becomes essential when writing libraries or modules meant for wider use or distribution, as docstrings provide end-users with a clear understanding of the function’s expected behavior.
Precise Type Annotations
Example
def find_max_value(numbers: list[int]) -> int: """Finds the maximum value in a list of integers.""" max_value = max(numbers) # Finds the largest integer in the list return max_value # Returns the largest value
Scenario
Type annotations are incredibly helpful in preventing runtime errors and improving code clarity. In a financial application that processes various calculations, type annotations ensure that the developer expects the correct data types (e.g., list of integers in this case). By providing clear types for both function arguments and return values, the risk of introducing bugs due to incorrect input types is minimized.
Ideal Users of Python Excellent Comments, Doc Strings & Types
Software Development Teams
Software development teams working on collaborative projects, particularly large-scale applications, can benefit immensely from this system. Teams working in industries such as finance, healthcare, or enterprise software require clear, well-documented code due to the complexity and long-term maintenance of these applications. Detailed comments, docstrings, and type annotations ensure that new team members can quickly understand the code and reduce onboarding time.
Python Instructors and Learners
Python educators and students will find this approach particularly useful. By seeing comprehensive examples of well-documented code, learners can understand best practices and adopt these practices early in their programming journey. Instructors can use this system to explain not just how the code works but also why particular types and structures are chosen, making it easier to teach and learn Python.
How to Use Python Excellent Comments, Doc Strings & Types
1
Visit aichatonline.org for a free trial without login; no need for ChatGPT Plus.
2
Ensure you have basic Python programming knowledge, including variables, functions, and control structures, to fully understand the enhanced features provided by this tool.
3
When coding, focus on writing clean, maintainable code. The tool will automatically enhance your comments, docstrings, and type annotations as you write, or provide refinements on existing code.
4
Use the tool for a variety of tasks—whether you're refactoring legacy code, writing new functions, or learning Python's best practices for typing and documentation.
5
Optimize your workflow by using the tool in conjunction with code editors like VSCode or PyCharm, which support detailed comments and type checking.
Try other advanced and practical GPTs
Code Maestro
Smart AI for coding and content creation

EX/IT Sales Bot
AI-Powered Sales Optimization
Sports Betting Bot
AI-powered insights for smarter betting

AnkiGPT - Auto Anki Flashcards
AI-powered flashcards for smarter learning.

Stock
AI-powered insights for smarter financial decisions

前端导师
AI-powered front-end development mentor.

Apple AppStoreConnect API Complete Code Expert
Automate Apple API integrations with AI-powered code.

Apple SceneKit Complete Code Expert
AI-powered SceneKit coding assistant.

Crypto快讯总结
AI-powered crypto news and trend analyzer

LogoGPT
AI-Powered Logo Designs Made Easy
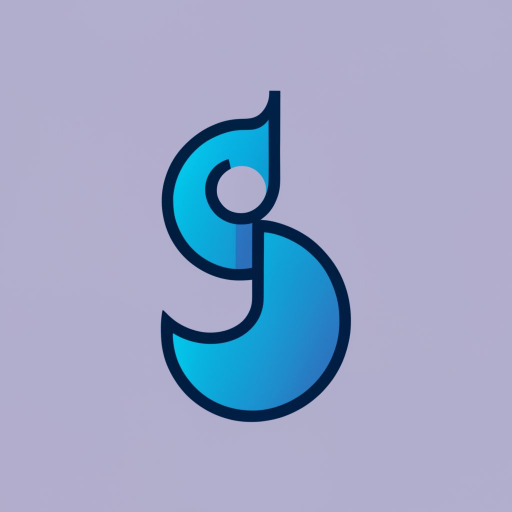
Amazon Commerce Expert | A-commerce Advisor
AI-powered insights for Amazon success
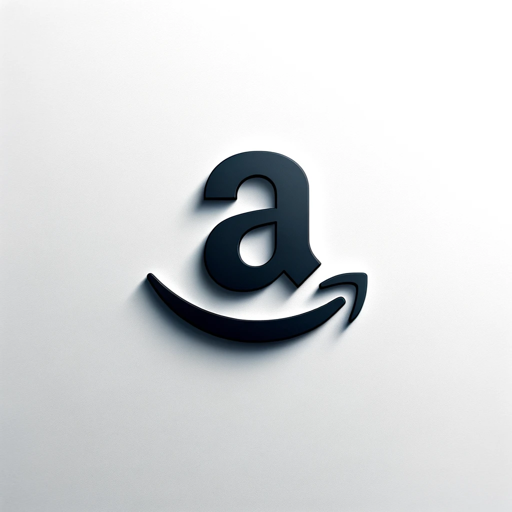
Montgomery Humane Society Assistant
AI-Powered Help for Pet Services
- Education
- Best Practices
- Code Review
- Documentation
- Refactoring
Common Questions About Python Excellent Comments, Doc Strings & Types
How does this tool improve Python code quality?
This tool adds detailed comments, clear docstrings, and precise type annotations to every function and line of code. It ensures your Python code is more readable, maintainable, and follows best practices in software development.
What kinds of projects benefit the most from this tool?
Projects that require clean code for collaboration, like open-source, academic research, or enterprise-level software, will benefit greatly. Additionally, it's excellent for educational settings where best practices in Python are taught.
Do I need to be an expert in Python to use this tool?
No. While basic Python knowledge helps, the tool provides significant guidance in making your code cleaner. It's designed to assist both beginners and advanced users with writing better-structured Python code.
How does it handle complex Python constructs?
The tool intelligently breaks down complex constructs, providing helpful comments and explanations for each part. It also supports type annotations for advanced features like generics, custom types, and decorators.
Can this tool help with debugging or just code clarity?
Primarily, this tool enhances code clarity, but clearer code can indirectly aid debugging by making it easier to trace and understand logic and data flows.