scala-AI-powered Scala guide
AI-powered Scala insights for developers

Related Tools
Load More
Kotlin Expert
Expert in Kotlin programming, offering tailored advice and solutions.

Kotlin
Your personal Kotlin copilot, assistant, and project generator with a focus on responsive, beautiful, and scalable apps. Write clean code and become a much faster developer.

JAVA CODER
Expert in Data Structures and Algorithms in Java

Java Guru
I'm a Java expert and trainer here to help you learn and solve Java problems.

Apple SwiftData Complete Code Expert
A detailed expert trained on all 1,401 pages of Apple SwiftData, offering complete coding solutions. Saving time? https://www.buymeacoffee.com/parkerrex ☕️❤️

Javafx master
Friendly JavaFX expert for all-around support.
20.0 / 5 (200 votes)
Introduction to Scala
Scala is a high-level programming language that combines object-oriented and functional programming paradigms. Designed by Martin Odersky, Scala was created to address some of the complexities and limitations of Java while providing a more concise, elegant syntax. It runs on the Java Virtual Machine (JVM), ensuring interoperability with Java. Scala's design purpose is to offer a language that supports a wide variety of programming styles and provides robust features for building scalable, maintainable applications. Scala is known for its concise syntax, powerful type inference, and support for immutability and higher-order functions. These features make it an ideal choice for tasks ranging from simple scripts to complex distributed systems. For example, Scala's case classes and pattern matching simplify the handling of immutable data structures, making code easier to read and maintain.
Main Functions of Scala
Pattern Matching
Example
```scala sealed trait Animal case class Dog(name: String) extends Animal case class Cat(name: String) extends Animal val animal: Animal = Dog("Buddy") animal match { case Dog(name) => println(s"This is a dog named $name") case Cat(name) => println(s"This is a cat named $name") } ```
Scenario
Pattern matching is used in scenarios where you need to perform different actions based on the structure of an object. For instance, in a system that processes different types of user commands, pattern matching can be used to handle each command type appropriately.
Immutability
Example
```scala val numbers = List(1, 2, 3) val newNumbers = 0 :: numbers println(newNumbers) // Output: List(0, 1, 2, 3) ```
Scenario
Immutability is crucial in multi-threaded environments where shared state can lead to concurrency issues. By ensuring that data structures cannot be modified after they are created, Scala helps prevent such problems, making it easier to reason about program behavior.
Higher-Order Functions
Example
```scala val numbers = List(1, 2, 3, 4, 5) val doubled = numbers.map(_ * 2) println(doubled) // Output: List(2, 4, 6, 8, 10) ```
Scenario
Higher-order functions are functions that take other functions as parameters or return them as results. They are particularly useful in scenarios involving data processing pipelines, such as transforming a list of numbers, filtering elements, or combining data from multiple sources.
Ideal Users of Scala
Data Engineers
Data engineers benefit from Scala's strong support for functional programming and its seamless integration with big data tools like Apache Spark. Scala's powerful type system and concise syntax make it easier to write and maintain complex data processing pipelines.
Backend Developers
Backend developers use Scala to build scalable, high-performance web services and APIs. Scala's interoperability with Java allows developers to leverage existing Java libraries while benefiting from Scala's advanced language features, such as pattern matching and immutability.
How to Use Scala
Visit aichatonline.org for a free trial without login, no need for ChatGPT Plus.
Access the website to start using the Scala tool without any registration or subscription requirements.
Install the Scala SDK
Download and install the Scala Software Development Kit (SDK) from the official Scala website. Ensure that Java is also installed as Scala runs on the Java Virtual Machine (JVM).
Set Up Your Development Environment
Choose an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse, and configure it for Scala development by installing necessary plugins.
Create a New Scala Project
Start a new project in your chosen IDE, set up the project structure, and begin writing Scala code. Familiarize yourself with Scala syntax and functional programming concepts.
Run and Test Your Scala Application
Compile and run your Scala application using the IDE. Test your code to ensure it behaves as expected. Utilize Scala’s testing frameworks such as ScalaTest or Specs2 for robust testing.
Try other advanced and practical GPTs
Music Composer Analysis작사 작곡
AI-powered music composition and analysis.

Activity Creation | QA Engineering | EnglishCode
AI-powered QA training activities for professionals
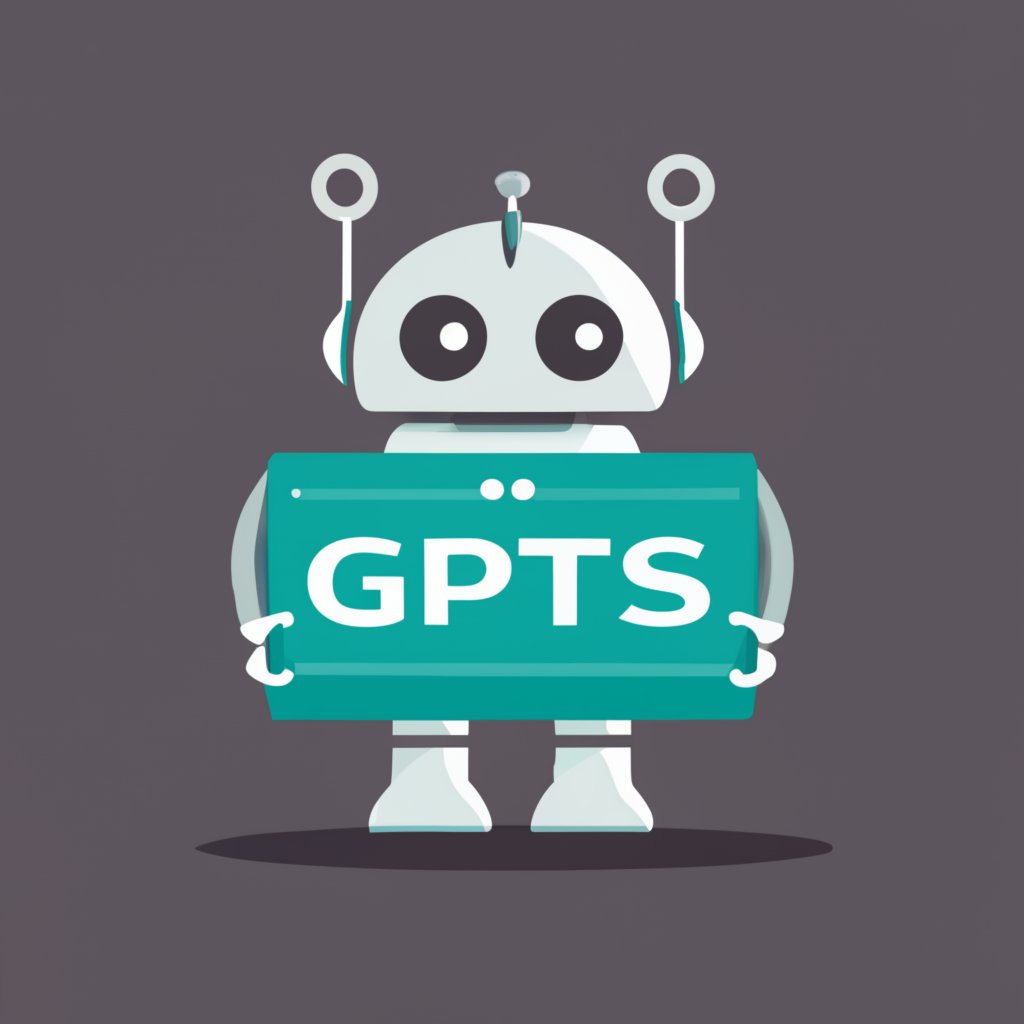
Yukkuri台本クリエイター
AI-powered Yukkuri script generator for engaging content.

Teachers of English Language Learners
AI-powered English learning for all levels

Sales Follow-Up Genius
AI-powered personalized sales follow-up.

Flutter Helper
AI-powered solutions for Flutter development

Master of Mastery
AI-powered tool for deep learning.

New GPT
AI-powered tool for all your tasks.

US General Aviation Expert
AI-powered aviation insights and support.

US Cultural Navigator
AI-powered tool for understanding American culture and language nuances

US GAAP Advisor
AI-powered US GAAP accounting advisor

Anti AI Detector Tool
Transform AI Text to Human-Like Content

- Web Development
- Data Processing
- Big Data
- Functional Programming
- Concurrent Programming
Scala Q&A
What is Scala?
Scala is a high-level programming language that combines object-oriented and functional programming paradigms. It is designed to be concise, elegant, and scalable, making it suitable for a wide range of applications.
Why should I use Scala?
Scala provides powerful features like immutability, type inference, and pattern matching, which enable developers to write clean, efficient, and maintainable code. It is also interoperable with Java, allowing the use of existing Java libraries.
How does Scala handle concurrency?
Scala uses the Actor model, particularly through the Akka framework, to manage concurrency. This approach allows for scalable and fault-tolerant systems by modeling concurrent processes as actors that communicate through message passing.
Can I use Scala for web development?
Yes, Scala is well-suited for web development. Frameworks like Play and Lift provide robust tools for building scalable and reactive web applications, leveraging Scala’s functional programming features.
How does Scala compare to other functional programming languages?
Scala stands out for its blend of object-oriented and functional programming, its seamless Java interoperability, and its rich type system. While languages like Haskell focus more on pure functional programming, Scala offers a practical balance that appeals to both functional and object-oriented programmers.