Unity Game Programming Patterns-Unity Game Programming Patterns Guide
AI-powered patterns for game development.

How do I implement the Singleton pattern in Unity?
Can you explain the Observer pattern in Unity game development?
What are the SOLID principles and how do they apply to Unity?
How can I refactor my Unity game code for better scalability?
Related Tools
Load More
Unity Helper
Hi fellow developer! Ready to assist with your Unity coding and game design queries.

Unity GPT
A specialized teacher for Unity Engine queries, providing detailed and practical guidance.

Unity Script Helper
Expert in Unity development, C# scripting - Finetuned with Unity official eBooks, documentation.
Unity C# God
"Unity C# God" is a GPT-based AI tool designed for Unity game development using C#. It offers expert-level assistance in coding, debugging, and optimization, making it an indispensable resource for both novice and experienced Unity C# developers seeking t

Unity Buddy - C# Programmer for Unity 3D
Provides Senior Gameplay Programming support for Unity 3D Game Development

Unity Mentor (Unity Assistant)
Provides assistance to developers, artists, and creators working with Unity, a popular game engine and development environment.
20.0 / 5 (200 votes)
Introduction to Unity Game Programming Patterns
Unity Game Programming Patterns are design approaches tailored to solving common development challenges within the Unity game engine. These patterns help developers write clean, maintainable, and scalable code by adhering to principles like separation of concerns and modularity. They offer standardized solutions to frequent issues like object creation, game state management, and optimizing performance. For example, the 'Singleton' pattern is commonly used to manage global game states, such as a player's score or the game settings, across scenes without duplicating objects. Another pattern, 'Object Pooling,' is ideal in situations where you need to instantiate multiple instances of objects, like bullets in a shooter game. By reusing objects instead of constantly creating and destroying them, the game’s performance can be significantly improved. These patterns enable Unity developers to optimize gameplay experiences while improving the maintainability of the codebase.
Key Functions of Unity Game Programming Patterns
Singleton Pattern
Example
The Singleton pattern ensures a class has only one instance and provides a global point of access to it.
Scenario
In a game, you might need a single instance of a 'GameManager' to manage score, player settings, or game state across multiple scenes. The Singleton pattern makes sure that only one instance of the 'GameManager' exists, preventing conflicts between different parts of the game.
Object Pooling
Example
Object Pooling improves performance by reusing objects rather than constantly instantiating and destroying them.
Scenario
In a first-person shooter game, the bullets shot by the player can be stored in a pool and reused. This avoids frequent memory allocations and deallocations, reducing lag during intensive gameplay sessions.
State Pattern
Example
The State pattern allows an object to alter its behavior when its internal state changes.
Scenario
For a character in a platformer game, the State pattern can be used to handle different states such as 'idle', 'running', 'jumping', or 'attacking'. Each state is encapsulated as a separate class, making the game logic easier to maintain and extend.
Ideal Users of Unity Game Programming Patterns
Professional Game Developers
Professional game developers who work on large-scale Unity projects will benefit from using design patterns to manage complexity, improve code readability, and maintain scalability. These patterns enable developers to optimize performance and ensure consistent behavior across complex game systems, which is essential for AAA or high-budget indie games.
Indie Developers and Hobbyists
Indie developers and hobbyists who may be working solo or with small teams can use Unity Game Programming Patterns to implement robust game systems without reinventing the wheel. By utilizing pre-existing, proven solutions like Singleton or Object Pooling, they can focus on game design and creativity while ensuring their game runs efficiently.
Guidelines for Using Unity Game Programming Patterns
Visit aichatonline.org for a free trial without login, no need for ChatGPT Plus.
You can explore various game programming patterns in Unity at no cost, without requiring additional paid services. The website provides an easy entry point for learning about game programming patterns.
Understand Prerequisites
Before diving into patterns, ensure familiarity with C# programming and the Unity environment. Basic knowledge of object-oriented principles, like inheritance and polymorphism, is key to making the most of programming patterns.
Identify Key Use Cases
Use patterns when managing game states, entities, or implementing reusable behavior. Common scenarios include managing AI, controlling game flow, or optimizing performance for resource-constrained platforms such as mobile.
Apply Patterns Systematically
Use patterns such as Singleton for managing global game states, Object Pool for memory efficiency, and Observer for event handling. Apply these in situations requiring scalable and maintainable code.
Test and Optimize
As with any game development process, use Unity's debugging tools and profilers to ensure the patterns are enhancing performance. Refactor code where necessary to maximize maintainability and performance.
Try other advanced and practical GPTs
Mr. Traductor PDF Pro
AI-powered PDF translations made easy

계약서 작성 법률 검토기
AI-powered legal contract review made easy.

Top Voice Badge Advisor
AI-powered recommendations for LinkedIn Top Voices
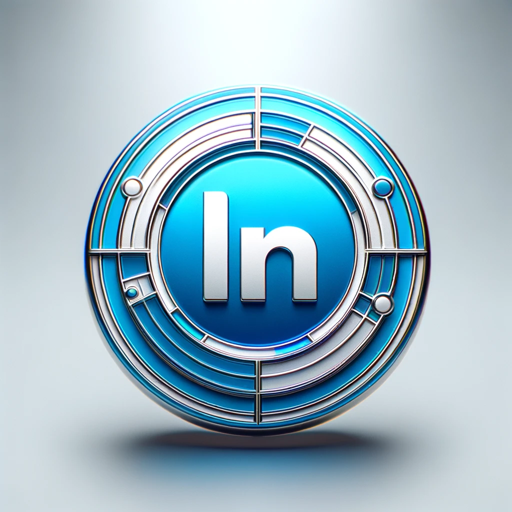
Lama Ivo's corner - Dzogchen and Tibetan Buddhism
AI-powered insights into Dzogchen wisdom
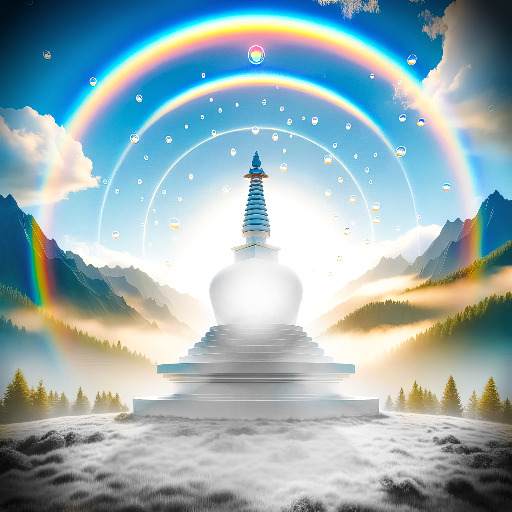
GPT Group Chat
AI-powered facilitator for dynamic chats

💎 Creative Frame Architect 💎
AI-powered chic cartoon portrait creation.

Boolio Global Invest GPT
AI-Powered Investment Analysis and Insights

Franzi's Quest
Embark on an AI-driven pirate adventure

PDF Summary
AI-powered PDF summarization made easy
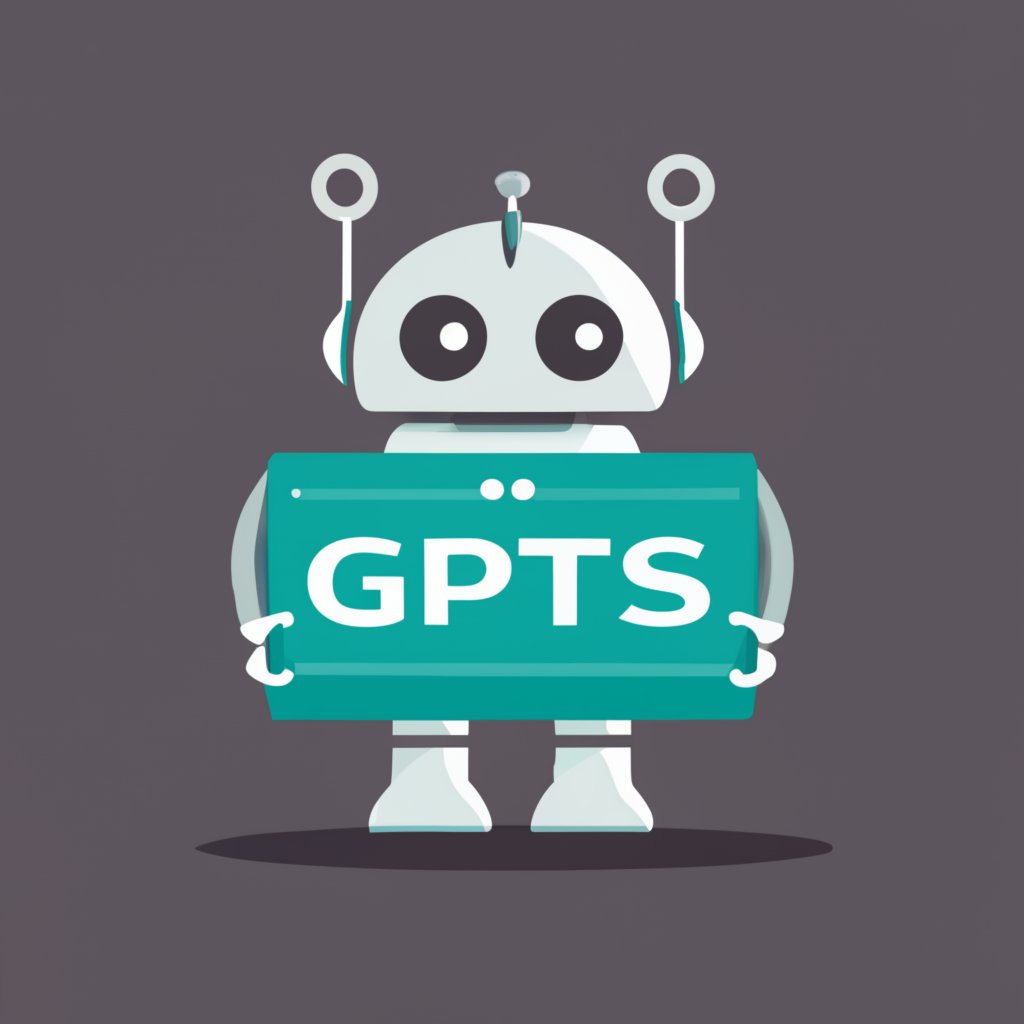
Active Campaign Expert
AI-powered automation for marketing success

Anaconda and Python Guide
AI-powered guide for Python and Anaconda

Translate German to English
AI-powered German to English Translation
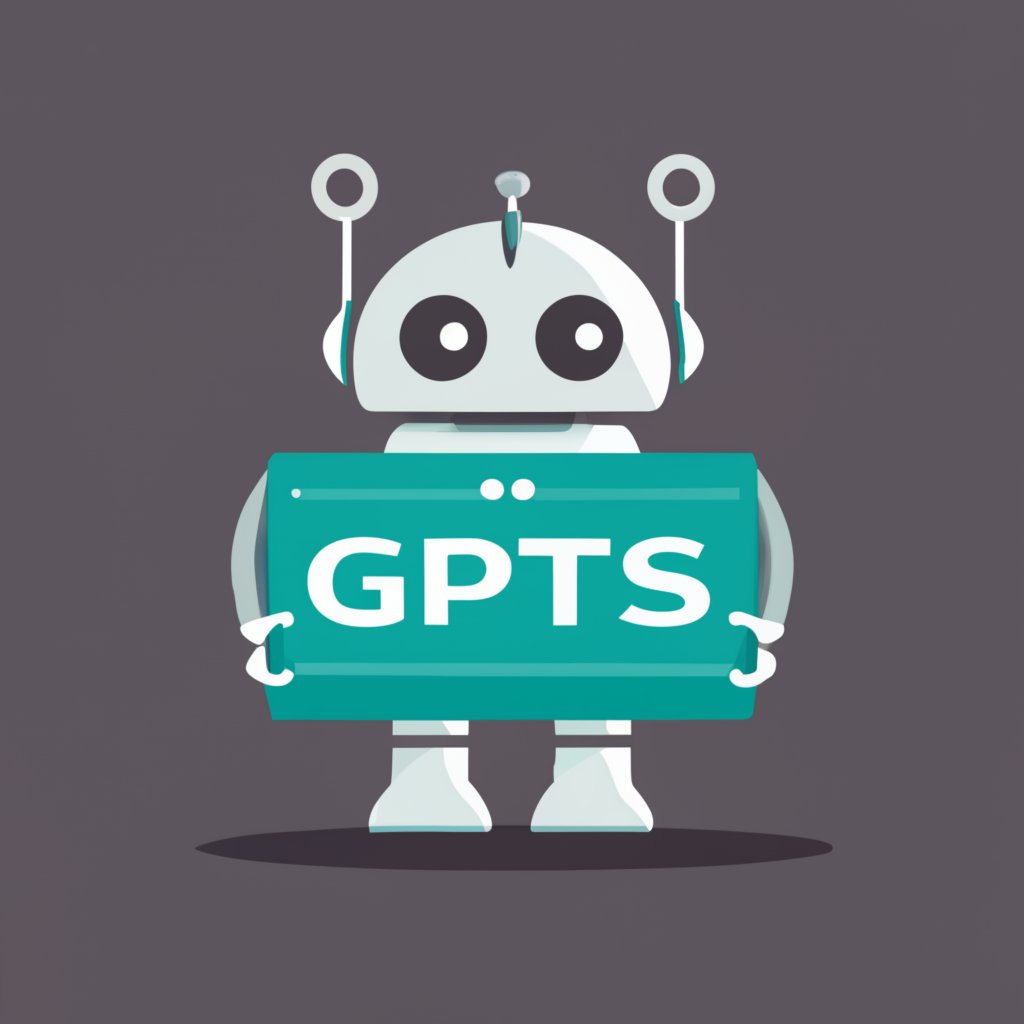
- Performance Optimization
- Event Handling
- Game States
- Object Pooling
- AI Control
Common Q&A on Unity Game Programming Patterns
What are Unity Game Programming Patterns?
These are pre-established design patterns applied in Unity development to solve recurring problems in game architecture, such as managing object creation, game states, and event handling.
When should I use the Singleton pattern in Unity?
Use Singleton when you need a class to have only one instance across the game, such as managing global game states or service managers like AudioManager.
How can I optimize performance with Object Pooling?
Object Pooling reduces memory allocation overhead by reusing objects rather than destroying and recreating them. It's particularly effective in games with many recurring objects, like projectiles or enemies.
Can I combine multiple patterns in a single Unity project?
Yes, patterns can be combined. For example, you can use the Observer pattern for event communication between systems, and the Factory pattern for object creation, ensuring flexibility and scalability.
How do I implement the Observer pattern in Unity?
You can implement the Observer pattern using C# events or Unity's event system. It allows objects (Observers) to subscribe to changes or events in another object (Subject), decoupling the code and making it more modular.