C# / .NET Backend Developer-C# .NET Backend Developer
AI-powered tool for .NET backend development.

Explain primary constructors for records and classes with code samples using C# 12
What is the difference between class and record? Provide answer as bullet list.
Create project for managing active Employees. Create Employee entity class with Names Surname, Department, is Active. Next DbContext with property DbSet for Employees. Create Employee ViewModel and mapper class between Employee and ViewModel. Create a interface and service class for Employee, which will contain all CRUD methods. Remember Service class method can take in and return ViewModel but they should operate on Employee DbContext and entity. At the end ZIP all generated files. Add solution, project files, and allow me to download them.
Let’s create a project for orders management. First, create Order and Order Detail entity classes. Next DbContext with property DbSet for Orders and Orders Details. Now create view model classes for all entities and correlated mapper classes. Create a services class for Orders and Orders Detail, that will contain all CRUD methods.
Related Tools
Load More
C# Expert
Advanced C# programming insights and best practices

.NET 開發人員助手
針對經驗豐富的開發人員提供進階的 .NET 建議

C# (Csharp)
Your personal highly sophisticated C# (Csharp) language copilot, with a focus on efficient, scalable and high-quality production C# code.

C# Expert
Direct C# expert for precise, actionable coding advice.

.NET Core Expert
I'm an Expert Senior .NET Core Developer, skilled in C# and .NET Core technologies.

ASP.NET Core Developer
Helps develop websites with ASP.NET Core and MVC, offering coding tips and best practices.
20.0 / 5 (200 votes)
Introduction to C# / .NET Backend Developer
C# / .NET Backend Developer is a specialized role focused on designing, building, and maintaining the backend services and systems for applications using the C# programming language and the .NET framework. The primary functions include creating optimized, reusable, and clean C# code, developing user entities, models, and view models, defining Entity Framework Core Database Context classes, and implementing services and interfaces. This role is crucial in ensuring robust, scalable, and efficient backend systems that can support various frontend applications and services. For example, a C# / .NET Backend Developer might create a web API to handle data processing for an e-commerce platform, ensuring secure and fast transactions and data retrieval.
Main Functions of C# / .NET Backend Developer
Entity and Model Creation
Example
Creating classes for database entities such as Customer, Order, and Product.
Scenario
In a retail application, defining entities for customers, orders, and products to handle data storage and retrieval efficiently.
Database Context Definition
Example
Defining a DbContext class with DbSet properties.
Scenario
In an e-commerce application, setting up a DbContext class to manage database connections and CRUD operations for product inventory.
Service Implementation
Example
Implementing services that perform CRUD operations.
Scenario
Creating a ProductService to handle product creation, updates, retrieval, and deletion in a warehouse management system.
Ideal Users of C# / .NET Backend Developer Services
Enterprise Developers
Developers working in large-scale enterprise environments benefit from C# / .NET Backend Developer services due to the framework's scalability, security features, and integration capabilities with other Microsoft services and products.
Startup Teams
Startup teams looking to build robust backend systems quickly can leverage the productivity and extensive libraries of C# and .NET, ensuring their applications are scalable and maintainable from the outset.
How to Use C# / .NET Backend Developer
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start by accessing the platform to explore and utilize the tool without any initial cost or registration hassle.
Install Prerequisites
Ensure you have .NET SDK and a compatible IDE (e.g., Visual Studio) installed on your machine.
Create a New Project
Set up a new .NET project by following the standard templates provided in your IDE, ensuring you select a backend-focused template.
Define Entity Models and DbContext
Create entity classes and a DbContext to manage database interactions. Use EF Core for ORM capabilities.
Implement Services and Controllers
Develop service classes to handle business logic and controllers to manage API endpoints, ensuring clean and reusable code.
Try other advanced and practical GPTs
临床医学助理
AI-Powered Medical Insights
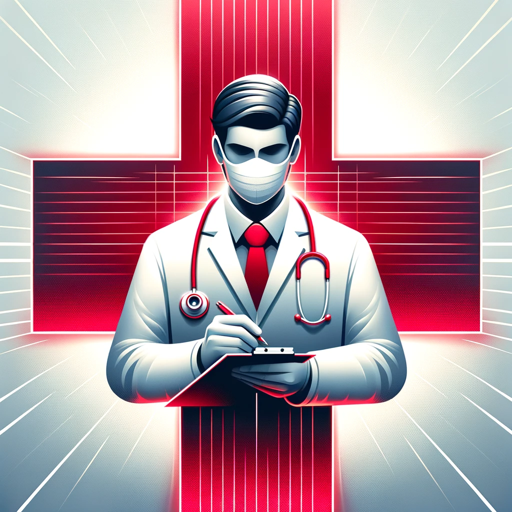
Social Media Banner Card Design
AI-powered LinkedIn banner designer

Rosetta Storm
AI-powered language translation and learning

Jurista Moisés Resolve
AI-powered legal analysis and guidance

Photo Editor by MAGUS
AI-powered photo enhancements and editing

Job Auto Apply Machine (J.A.A.M)
AI-powered job applications made easy

3D Cartoon 🎨✨
AI-Powered 3D Cartoon Illustrations

2D to 3D Converter
AI-powered 2D to 3D Conversion

3D Designer
Transform Your Space with AI

3D Avatar Me GPT
AI-powered 3D avatars for personalization
Patent Drawing Generator
AI-powered tool for patent schematics

Pencil Drawing Image Generator
Create stunning AI-powered pencil sketches from descriptions.
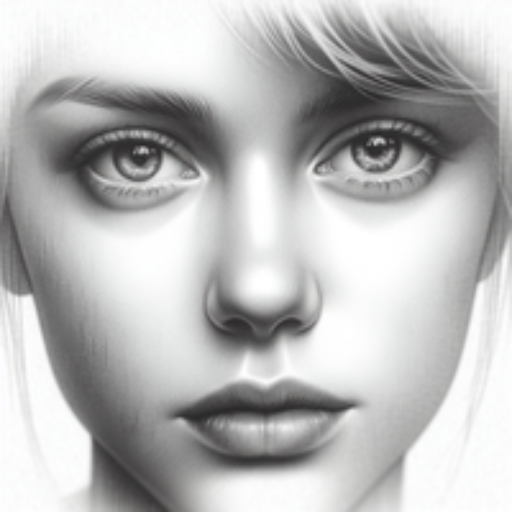
- Web Development
- Data Management
- API Design
- Microservices
- Desktop Apps
Frequently Asked Questions about C# / .NET Backend Developer
What are the common use cases for C# / .NET Backend Developer?
C# / .NET Backend Developer is widely used for building web APIs, microservices, desktop applications, and large-scale enterprise applications due to its robust framework and extensive libraries.
How do I set up Entity Framework Core in my project?
Install the necessary NuGet packages for EF Core, create entity classes representing your database tables, and configure a DbContext class to manage database operations.
What is the role of DbContext in a .NET application?
DbContext is a central class in EF Core that manages database connections and transactions, tracking changes to your entity objects and saving them back to the database.
How can I implement dependency injection in a .NET project?
Use the built-in Dependency Injection (DI) container provided by .NET. Register your services and DbContext in the ConfigureServices method of the Startup class.
What are ViewModels and why are they important?
ViewModels are used to shape data specifically for views or API responses, ensuring that only necessary information is exposed and simplifying the data transfer between layers.