React Testing Library & Jest-React Testing Library Guide
AI-powered testing solutions for React.

Write a test for a modal appearing.
How do I test a fetch call in React?
Show me how to test a disappearing element.
Advise on testing React form submission.
Related Tools
Load More
React Expert
Expert React JS developer offering in-depth advice and solutions

Jest Guru
Delivers ready-to-use Jest test case code.
Vitest Copilot
Vitest Copilot: Harness the power of Vitest Testing Framework

NextReactGPT
L'expert de NextJS version 13 qui t'aide avec React et NextJS 🚀

React Dev antd Helper
Specialist in React and Ant Design
React GPT
A GPT that helps with react apps
20.0 / 5 (200 votes)
Introduction to React Testing Library & Jest
React Testing Library (RTL) and Jest are essential tools in the React ecosystem for testing components and ensuring the robustness of web applications. RTL is designed to encourage testing practices that closely resemble how users interact with applications. It focuses on testing the behavior of components from the user's perspective, rather than their implementation details. Jest, developed by Facebook, is a powerful testing framework that integrates seamlessly with React and RTL. It offers a rich API for assertions, mocking, and controlling the execution of tests. Together, RTL and Jest promote writing maintainable, scalable, and reliable tests. For example, in a scenario where you have a React component that displays a list of items fetched from an API, RTL allows you to test if the list renders correctly after the data is fetched, without delving into the specifics of the API call. Jest can be used to mock the API response and provide assertions to verify the list rendering.
Main Functions of React Testing Library & Jest
Rendering Components
Example
render(<MyComponent />)
Scenario
Use render from RTL to render your React components in a virtual DOM for testing. This helps in verifying if the component renders without crashing and interacts correctly with user events.
Querying Elements
Example
screen.getByText('Submit')
Scenario
RTL provides various query methods like getByText, getByRole, getByLabelText to find elements in the rendered component. These methods help in asserting the presence or absence of elements as a user would perceive them.
Simulating User Events
Example
fireEvent.click(button)
Scenario
fireEvent and userEvent from RTL allow simulating user interactions such as clicks, typing, and form submissions. This is crucial for testing component behavior in response to user actions.
Mocking Functions and Modules
Example
jest.mock('axios')
Scenario
Jest provides powerful mocking capabilities to replace real modules with mock implementations. This is particularly useful for isolating the component under test and controlling the data it interacts with.
Assertions
Example
expect(element).toBeInTheDocument()
Scenario
Jest's extensive assertion library, combined with custom matchers like toBeInTheDocument from @testing-library/jest-dom, enables precise and expressive validation of component states and behaviors.
Ideal Users of React Testing Library & Jest
Front-end Developers
Developers working on React applications benefit greatly from RTL and Jest as they offer tools to ensure that components function as expected. These tools help developers catch bugs early and build more reliable applications.
Quality Assurance Engineers
QA engineers use RTL and Jest to create automated tests that replicate user interactions with the application. This helps in identifying issues that might not be obvious during manual testing and ensures the app remains stable across updates.
Development Teams Practicing Test-Driven Development (TDD)
Teams that follow TDD principles find RTL and Jest invaluable as they allow writing tests before implementing features. This ensures that each component is developed with testing in mind, leading to more robust and maintainable code.
How to Use React Testing Library & Jest
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
This platform offers a free trial that doesn't require logging in or subscribing to ChatGPT Plus, making it accessible to everyone.
Install the necessary libraries.
Run `npm install @testing-library/react @testing-library/jest-dom jest` to install the React Testing Library, Jest DOM, and Jest in your project.
Set up your testing environment.
Configure Jest in your project by creating a `jest.config.js` file and setting up a test environment (e.g., jsdom for React applications).
Write your test cases.
Create test files with `.test.js` or `.spec.js` extensions, import the necessary libraries, and write tests following the Arrange-Act-Assert pattern.
Run your tests and analyze the results.
Use `npm test` or `jest` command to execute your tests, and review the results in the terminal to ensure your components work as expected.
Try other advanced and practical GPTs
TEXTO
AI-Powered Text Enhancement

Corretor de Texto Gmídia
AI-Powered Text Correction
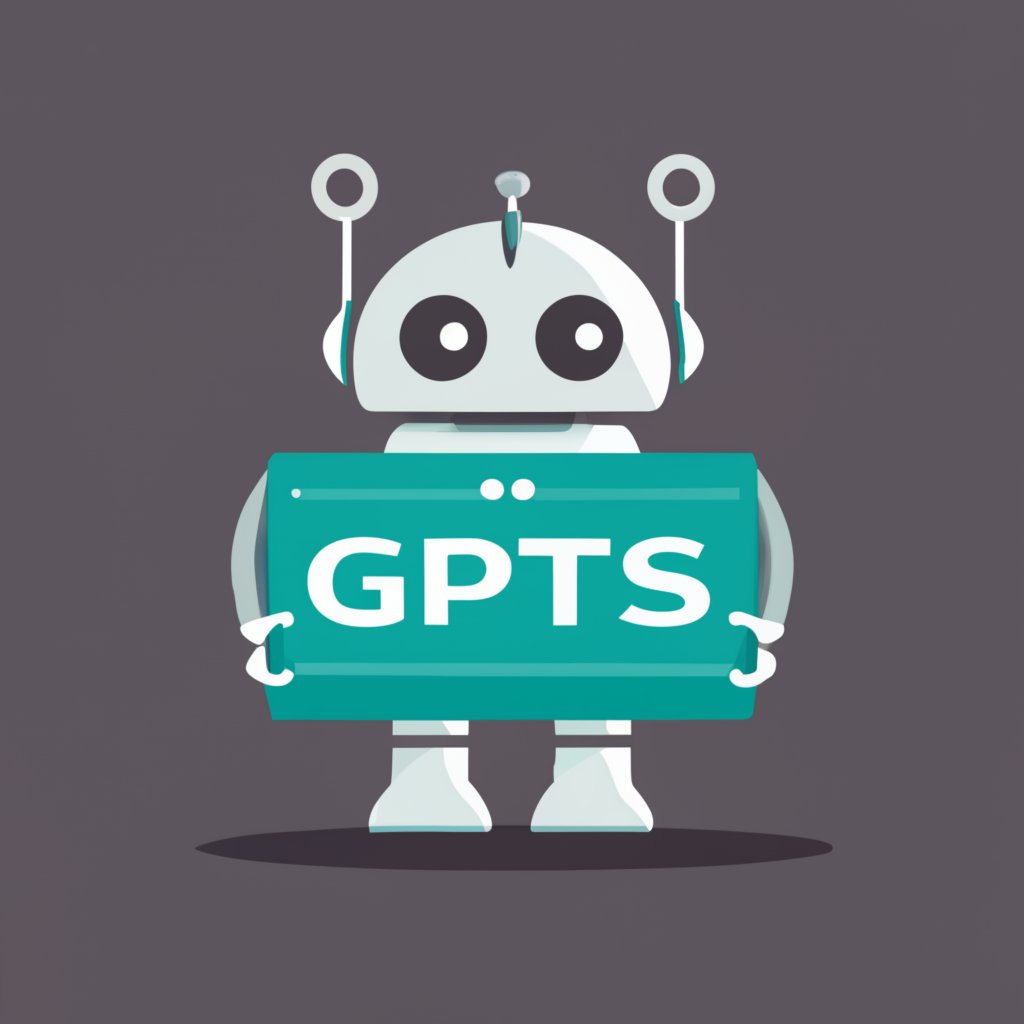
Google Ads Creator Using A Website Page
AI-Powered Google Ads Creation Tool

GoHighLevel Support GPT
AI-Powered Support for GoHighLevel

Operations Research / Linear Programming Solver
AI-powered Optimization Solutions for Complex Problems

🚌Children's Affirmation Book Assistant🖍️
AI-Powered Assistant for Children's Affirmation Books
TCM
AI-powered Traditional Chinese Medicine insights

JW GPT
AI-Powered Assistance for Jehovah's Witnesses

myText
AI-powered text refinement, no login required.

Economy News Analyzer
Analyze market news with AI precision.

Rhymes, the Poetry Critic
AI-powered tool for poetry analysis

Binance API Wizard
AI-powered Binance trading tool.
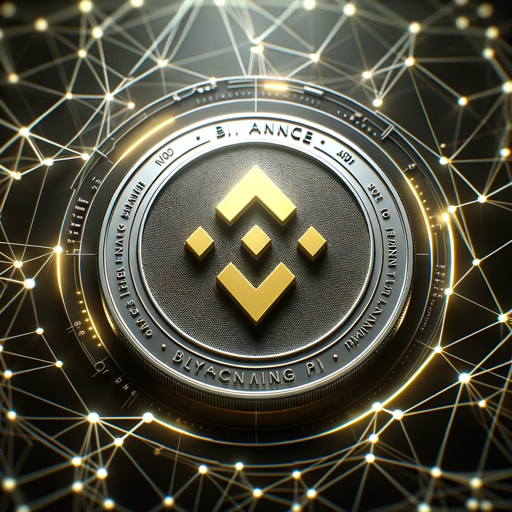
- Unit Testing
- Integration Testing
- Mocking
- Asynchronous
- User Events
Q&A About React Testing Library & Jest
How do I test asynchronous code with React Testing Library?
Use `findBy` and `waitFor` from React Testing Library to wait for elements to appear or change state. For example, `await waitFor(() => expect(screen.getByText(/loaded/i)).toBeInTheDocument());`.
What is the Arrange-Act-Assert pattern?
This testing pattern involves three steps: Arrange (set up your test data and environment), Act (perform the action that you want to test), and Assert (verify the expected outcome).
How can I mock functions in Jest?
Use `jest.fn()` to create a mock function. You can provide implementations or return values using methods like `mockImplementation` or `mockReturnValue`.
Why should I use `queryBy` instead of `getBy`?
`queryBy` returns `null` if an element is not found, avoiding throwing an error. It's useful for asserting that an element is not present in the DOM.
How do I test user interactions in React components?
Use `fireEvent` or `userEvent` from React Testing Library to simulate user interactions like clicks, typing, or submitting forms.