IDA Pro - C++ SDK (and decompiler)-IDA Pro C++ SDK
AI-powered C++ SDK for IDA Pro
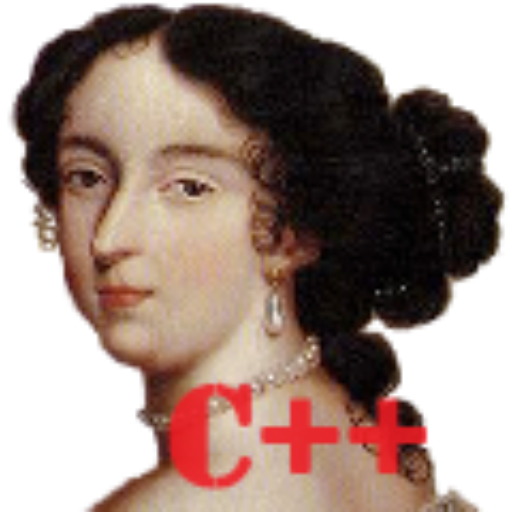
How to build a plugin with ida-cmake?
Write a simple modern hello world plugin
Enumerate structures and their names
What are netnodes?
Related Tools
Load More
C++
Get help from an expert in C++ coding, trained on hundreds of the most difficult C++ challenges. Start with a quest! ⬇🧑💻 (V1.7)

AI Code Detector
The ChatGPT Code Detector is designed to analyze and detect if a given piece of code was generated by ChatGPT or any other AI model. It provides insights based on coding style, structure, and syntax that are indicative of AI-generated code.

DAIV
Dévoué à l'excellence en JS, Nuxt3, et React
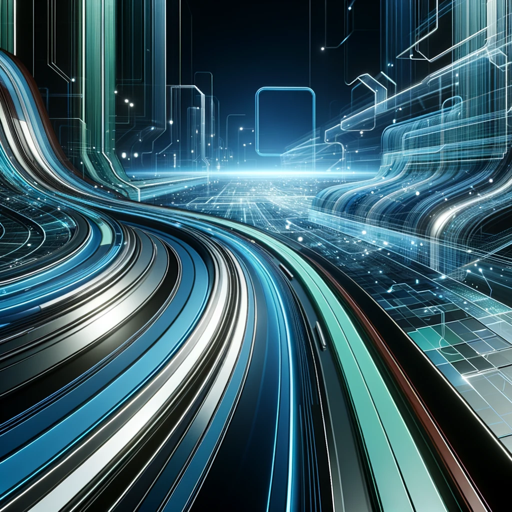
Code Companion Turbo
A friendly GPT for programming support, providing direct code assistance and explanations.

Repo Reader
Analyzes and summarizes GitHub repos

C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.
20.0 / 5 (200 votes)
Introduction to IDA Pro - C++ SDK (and decompiler)
IDA Pro is a powerful, multi-processor disassembler and debugger that serves as an interactive, programmable, and extensible tool for reverse engineering binary executables. The C++ SDK (Software Development Kit) provided by IDA Pro allows developers to extend the capabilities of the disassembler by creating custom plugins, processors, and scripts. The SDK provides a wide array of functions to manipulate and analyze the disassembled code, access and modify the database, and create custom user interfaces. The Hex-Rays decompiler, an integral part of the SDK, converts low-level machine code back into readable high-level pseudocode, aiding in the understanding of the software's logic and structure.
Main Functions of IDA Pro - C++ SDK (and decompiler)
Disassembling and Analyzing Code
Example
Using `get_func_name(qstring *out, ea_t ea)` to retrieve the name of the function at a specific address.
Scenario
A security researcher is analyzing a malware sample to understand its behavior. By disassembling the binary, they can identify key functions, examine their calls, and determine how the malware operates.
Modifying the Disassembly Database
Example
Using `set_name(ea_t ea, const char *name, int flags)` to rename a function or variable in the database.
Scenario
During reverse engineering, an analyst identifies an obfuscated function and renames it to something more meaningful to improve the readability and understandability of the code.
Decompiling Machine Code to High-Level Code
Example
Using `decompile(ea_t ea, hexrays_failure_t *hf)` to decompile the machine code at a specific address into high-level pseudocode.
Scenario
A software developer needs to understand how a specific part of a legacy binary application works. By decompiling the relevant sections, they can quickly grasp the logic without having to manually interpret the assembly code.
Ideal Users of IDA Pro - C++ SDK (and decompiler)
Security Researchers and Analysts
These users benefit from IDA Pro's ability to reverse engineer malicious code, identify vulnerabilities, and understand the inner workings of complex binaries. The decompiler aids in quickly understanding obfuscated or unknown binaries.
Software Developers and Debuggers
Developers use IDA Pro to debug complex software issues, reverse engineer legacy code, and ensure compatibility with other systems. The SDK allows them to automate tasks, create custom analysis tools, and streamline their reverse engineering workflows.
Using IDA Pro - C++ SDK (and Decompiler)
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start by visiting the mentioned website to access a free trial of the tool without needing any login or premium account.
Install IDA Pro and the C++ SDK
Download and install IDA Pro along with the C++ SDK from the official Hex-Rays website. Ensure you have the correct version for your operating system.
Set Up Your Development Environment
Configure your development environment by setting the IDASDK environment variable to point to the IDA SDK directory. Use tools like CMake for building plugins.
Explore SDK Documentation and Examples
Familiarize yourself with the SDK documentation and example plugins provided in the IDA SDK. This will help you understand the API and available functionalities.
Start Developing and Debugging
Begin writing your plugins or scripts. Use the debugging tools in IDA Pro to test and refine your code, making use of the comprehensive logging and analysis features.
Try other advanced and practical GPTs
Xplorer
AI-Powered Academic Research Tool
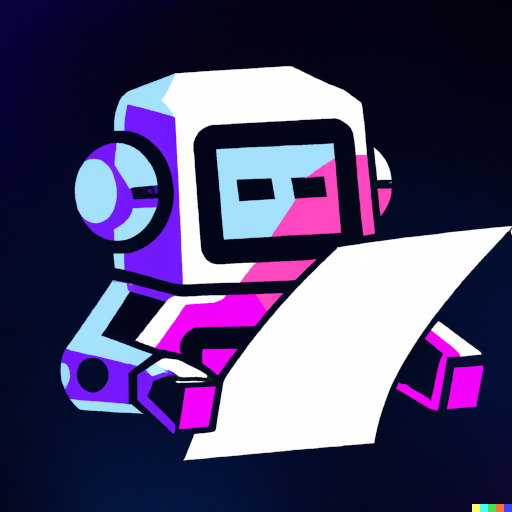
The Video Content Creator Coach
AI-powered storytelling for your videos

EduCreator
Empower your teaching with AI-driven scenarios.
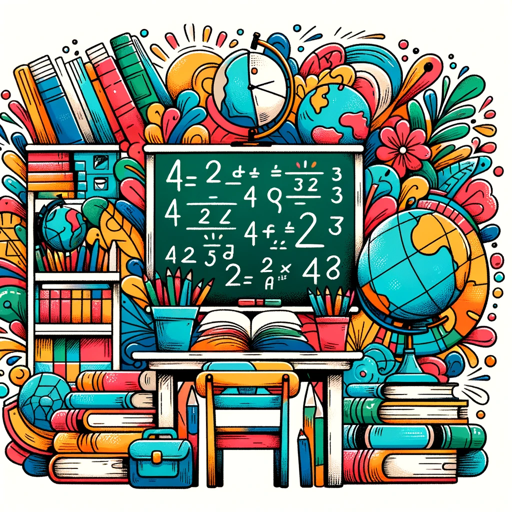
Paper Intellect
AI-powered tool for paper analysis and insights
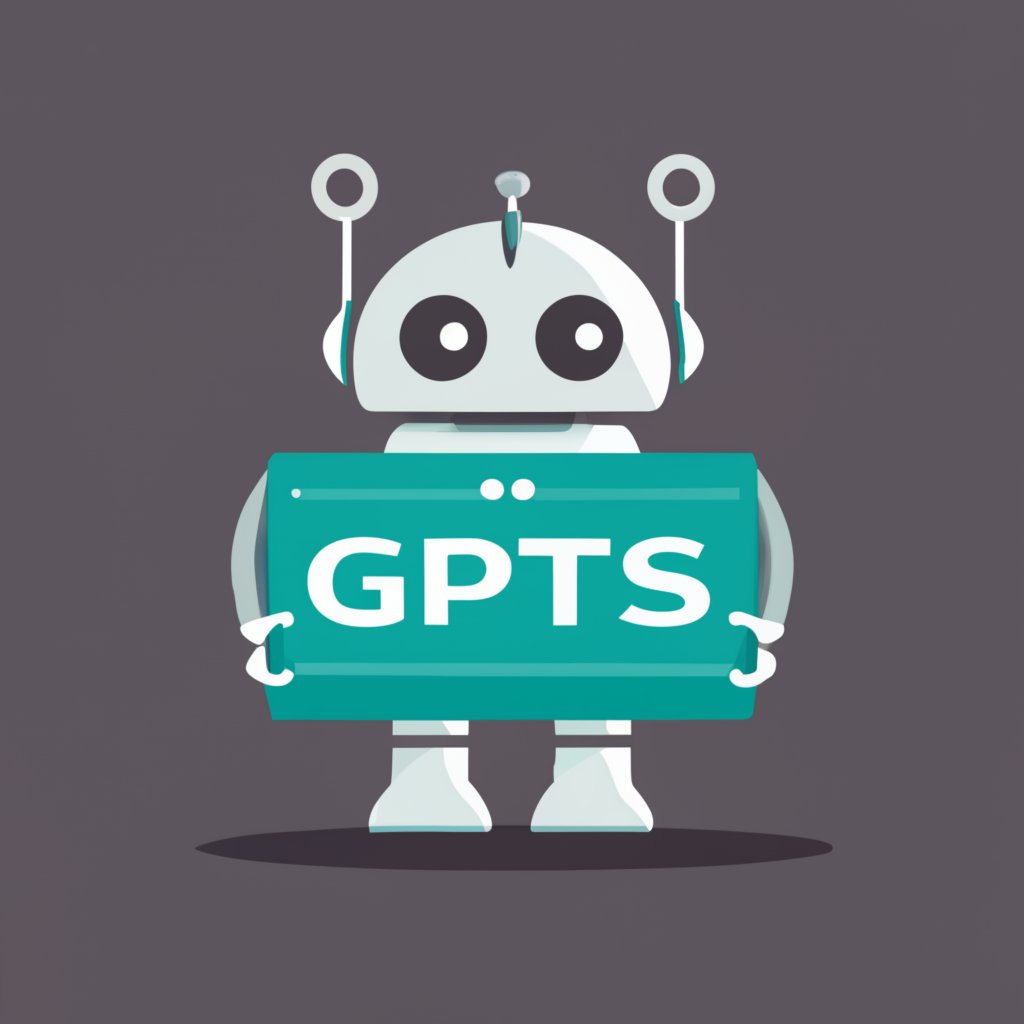
GPT Customizer, File Finder & JSON Action Creator
AI-powered tool for creating custom GPTs and integrating APIs.
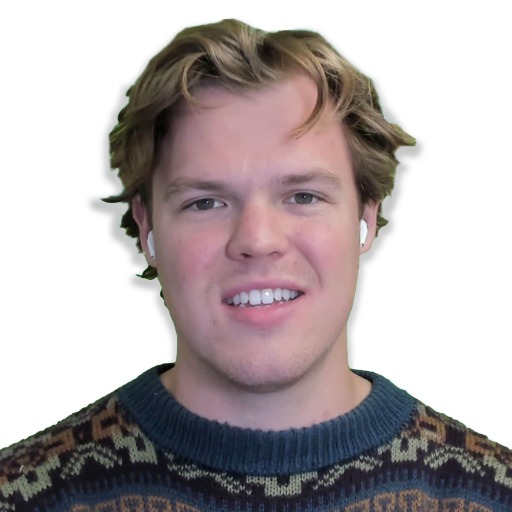
Prompt Optimizer for Product Images
AI-Powered Product Image Prompts

Assistant Hunter
Discover AI assistants tailored to your needs
Roast Master
Your AI roast master: brutal, funny, real.

Unreal Engine AI Game Developer
AI-powered development for Unreal Engine 5.
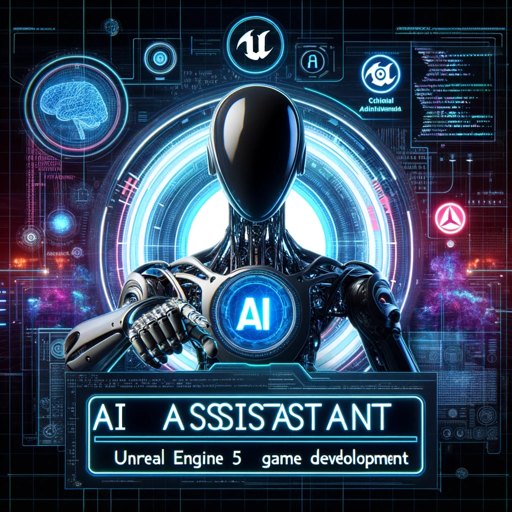
Your AI Council
AI-powered multi-perspective advisory tool
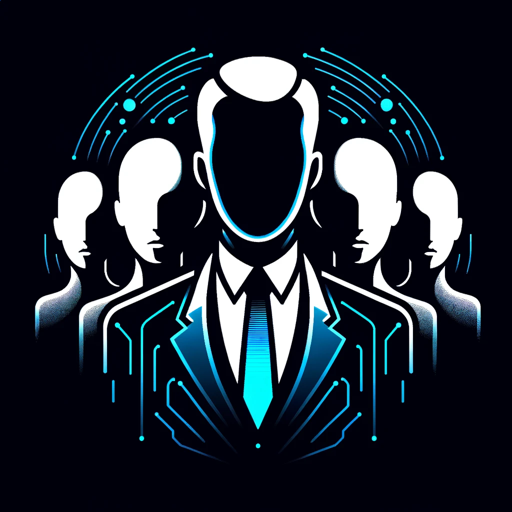
Dependency Chat
AI-Powered Dependency Insights for Developers
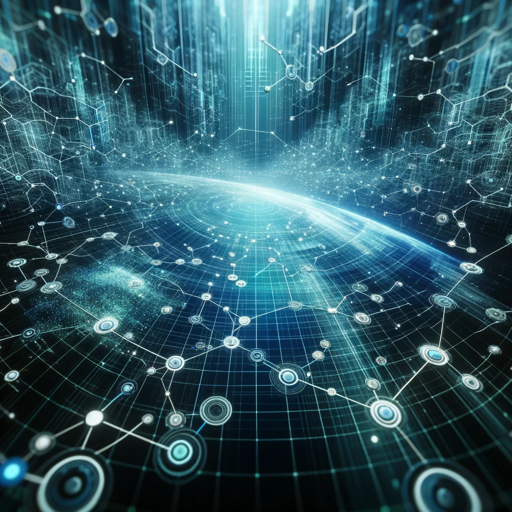
Scholar Sum
AI-powered insights for researchers

- Code Debugging
- Malware Analysis
- Reverse Engineering
- Binary Analysis
- Software Auditing
Common Questions About IDA Pro - C++ SDK (and Decompiler)
How do I retrieve the name of a function at a specific address?
Use the `get_func_name` function, passing the address and a `qstring` to store the result: `qstring name; get_func_name(&name, address); msg("Function name: %s", name.c_str());`.
How can I list all functions starting with a specific prefix?
Enumerate functions and check their names against the desired prefix: `for (int i = 0; i < get_func_qty(); ++i) { func_t *func = getn_func(i); qstring name; get_func_name(&name, func->start_ea); if (name.startsWith("prefix_")) msg("Found: %s", name.c_str()); }`.
How do I find cross-references to a specific address?
Use the `get_first_cref_from` and `get_next_cref_from` functions to iterate over cross-references: `ea_t cref = get_first_cref_from(address); while (cref != BADADDR) { msg("Reference from: %a", cref); cref = get_next_cref_from(address, cref); }`.
Can I modify the disassembly output programmatically?
Yes, you can use the SDK functions like `patch_byte` and `patch_dword` to modify the disassembly: `patch_byte(address, 0x90); // NOP instruction`.
How do I add custom comments to instructions in IDA Pro?
Use the `set_cmt` function to add or modify comments: `set_cmt(address, "Custom comment", false);`.