JavaScript Code Interpreter-real-time JavaScript execution
AI-powered JavaScript coding made easy

Write a JS function to sort an array
Explain closures in JavaScript
How do I use promises in JavaScript?
Show the current deno version
Related Tools
Load More
Advanced JavaScript Assistant
A friendly JavaScript programming assistant, ready to assist you.

Java Script Prodigy
Ultimate JavaScript assistant with advanced knowledge and interactive learning support.
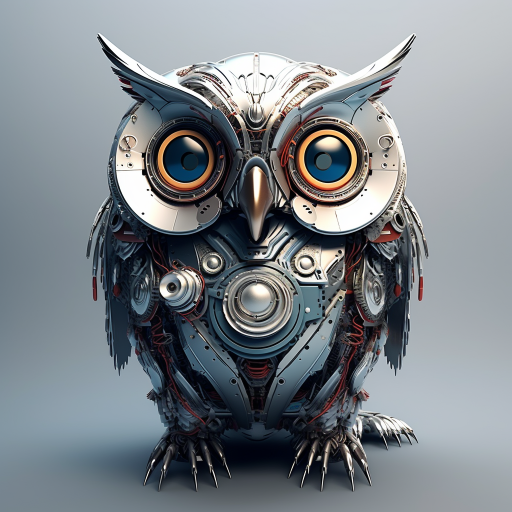
JavaScript Guru
Master Class - Teaching and creating code examples.

JavaScript Developer
This GPT model is tailored to teach and assist with JavaScript programming.

JavaScript expert
Transform your JavaScript code with expert refactoring tips and tricks!

JS Code Creative
A creative coding assistant for JavaScript, RealJS, Next.js, and CSS.
20.0 / 5 (200 votes)
Introduction to JavaScript Code Interpreter
The JavaScript Code Interpreter is a tool designed to execute JavaScript code in a sandboxed environment, providing immediate feedback and results. This interpreter can handle various tasks, from simple scripts to more complex programming challenges, making it an essential tool for developers, educators, and learners. By allowing users to run and test JavaScript code instantly, it facilitates rapid prototyping, debugging, and learning. For instance, if a user wants to understand how a particular JavaScript function works, they can write the function, execute it, and see the output immediately. This interactive approach aids in a deeper understanding of JavaScript concepts and improves coding skills.
Main Functions of JavaScript Code Interpreter
Code Execution
Example
Running a simple JavaScript function to add two numbers: `function add(a, b) { return a + b; } console.log(add(2, 3));`
Scenario
A developer can quickly test the logic of a function they are writing without needing to set up a full development environment. This speeds up the development process and helps in catching errors early.
Debugging
Example
Using `console.log` to print variable values at different stages of execution: `let x = 10; console.log(x); x += 5; console.log(x);`
Scenario
When encountering an issue in their code, a developer can insert `console.log` statements to track the values of variables and understand where things might be going wrong. This is particularly useful in scenarios where the bug is not immediately obvious.
Learning and Education
Example
Illustrating how closures work in JavaScript: `function outer() { let count = 0; return function inner() { count++; console.log(count); } } let closure = outer(); closure(); closure();`
Scenario
Educators can use the interpreter to demonstrate JavaScript concepts in real-time. Students can experiment with the code snippets provided and observe the outcomes, enhancing their learning experience through practical application.
Ideal Users of JavaScript Code Interpreter
Developers
Developers benefit from the JavaScript Code Interpreter by using it as a quick testing tool. They can write and execute snippets of code to verify logic, debug issues, or prototype new features without the overhead of a full development setup. This enhances productivity and allows for more efficient coding practices.
Students and Educators
Students learning JavaScript can use the interpreter to practice coding and see immediate results, which reinforces their understanding of programming concepts. Educators can leverage the tool to create interactive lessons and provide live coding demonstrations, making the learning process more engaging and effective.
How to Use JavaScript Code Interpreter
Step 1
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Step 2
Ensure your system meets the prerequisites: modern web browser, internet connection, and enabled JavaScript.
Step 3
Familiarize yourself with the interface, focusing on the code editor and console output sections.
Step 4
Write or paste your JavaScript code into the editor. Use console.log() for outputting results.
Step 5
Execute your code by clicking the 'Run' button and review the output in the console section.
Try other advanced and practical GPTs
GPT Finder by Skill Leap AI
Discover AI-powered solutions effortlessly.

MaiL PRO
AI-driven email templates for all screens

Task Management Assistance
AI-powered task prioritization and planning.

StableDiffusionGPT
AI-powered image generation at your fingertips.

Superlocal
AI-powered local system control

Academic Research Reviewer
AI-powered feedback for academic manuscripts.
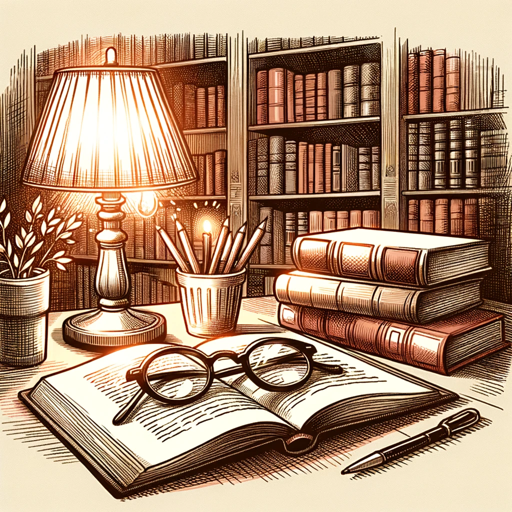
Asistente de Presentaciones Avanzadas
AI-powered tool for dynamic presentations

SensualGPT
Unleash playful conversations with AI-powered innuendo.
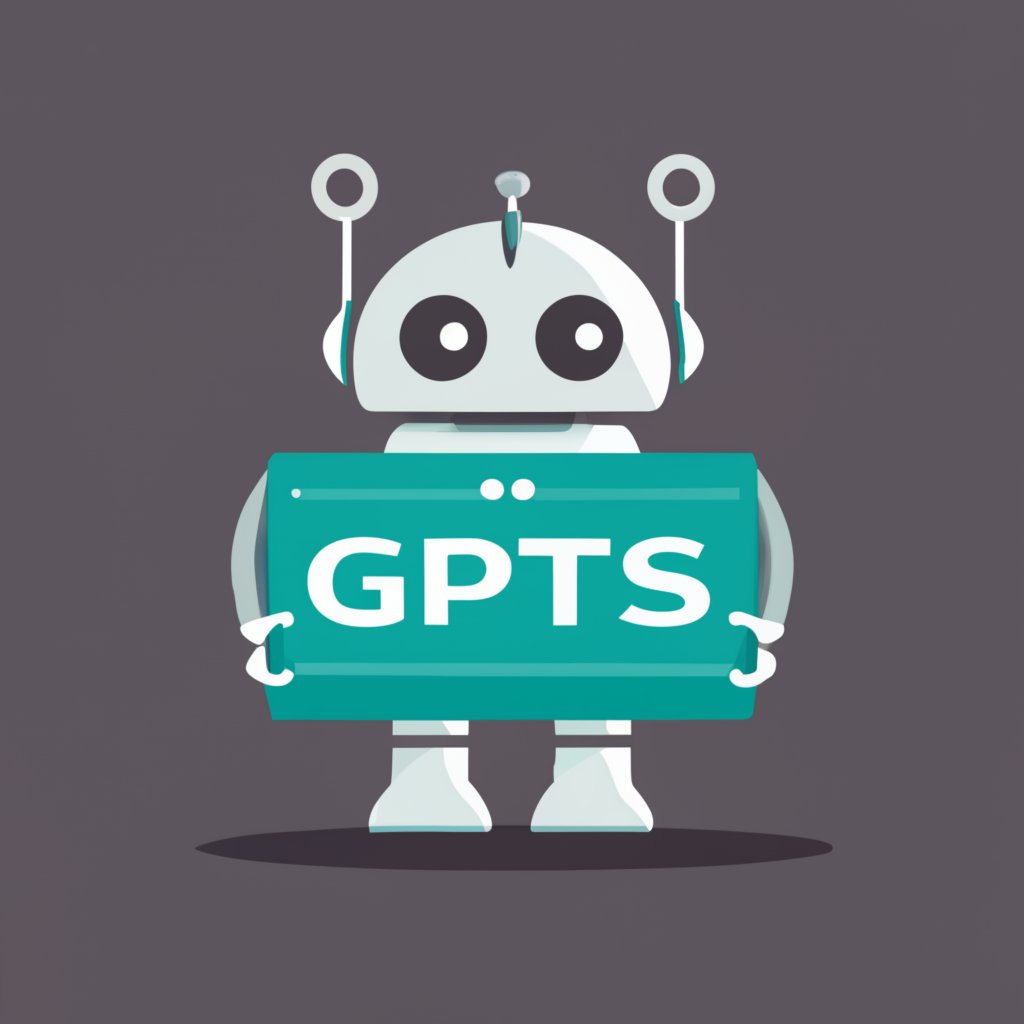
Notion Wizard
AI-powered Notion data insights

Balance Sheet Analyzer
AI-Powered Financial Insight Tool
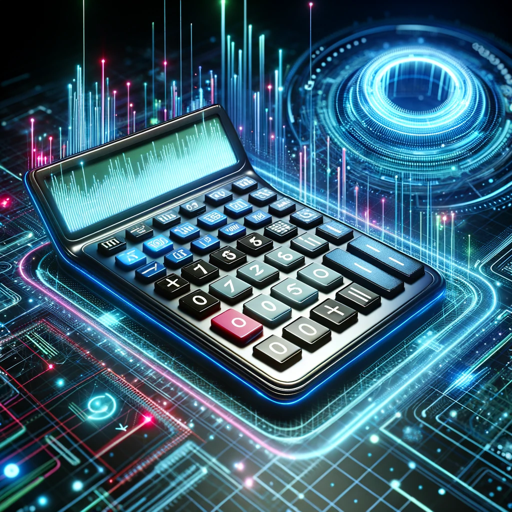
Crypto Coach
AI-powered cryptocurrency education and analysis.

Visual Character Recognition | Vision Assisted OCR
AI-Powered Text Extraction from Images
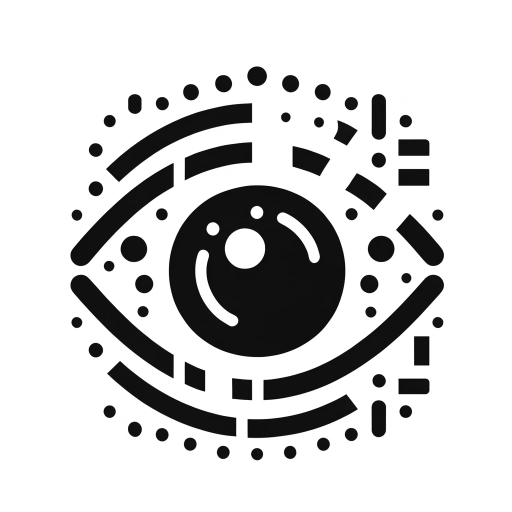
- Debugging
- Prototyping
- Learning Tool
- Code Testing
- Experimentation
JavaScript Code Interpreter Q&A
What is the JavaScript Code Interpreter?
The JavaScript Code Interpreter is an online tool that allows you to write, execute, and debug JavaScript code in real-time, directly in your web browser.
Do I need an account to use the JavaScript Code Interpreter?
No, you do not need an account or a subscription to use the JavaScript Code Interpreter. You can start using it immediately by visiting the website.
What are the common use cases for the JavaScript Code Interpreter?
Common use cases include learning JavaScript, testing code snippets, debugging code, and experimenting with new JavaScript features.
Can I save and download my JavaScript code?
Yes, the tool allows you to save your code to a file on your local system for future use or sharing with others.
Does the JavaScript Code Interpreter support libraries and frameworks?
Yes, you can include libraries and frameworks such as jQuery, React, or Lodash by including their CDN links in your code.